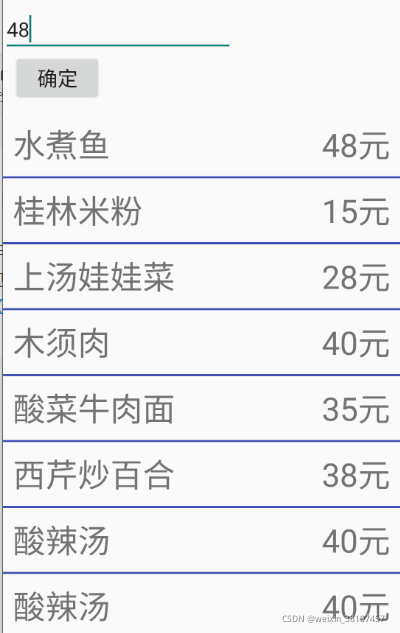
package com.example.testapplication;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import android.widget.Toast;
import androidx.annotation.Nullable;
import com.example.testapplication.adapter.ListViewAdapter;
import com.example.testapplication.entity.Food;
import java.util.ArrayList;
import java.util.List;
public class FoodListActivity extends ApplicationActivity{
ListView listView;
List<Food> foodList = new ArrayList<>();
List<Food> selectedList = new ArrayList<>();
EditText editText;
Button button;
ListViewAdapter listViewAdapter;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_food_list);
listView = findViewById(R.id.list_view);
button = findViewById(R.id.buttonfood);
editText = findViewById(R.id.edit_text);
foodList.add(new Food("麻辣香锅", 55));
foodList.add(new Food("水煮鱼", 48));
foodList.add(new Food("麻辣火锅", 80));
foodList.add(new Food("清蒸鲈鱼", 68));
foodList.add(new Food("桂林米粉", 15));
foodList.add(new Food("上汤娃娃菜", 28));
foodList.add(new Food("红烧肉", 60));
foodList.add(new Food("木须肉", 40));
foodList.add(new Food("酸菜牛肉面", 35));
foodList.add(new Food("西芹炒百合", 38));
foodList.add(new Food("酸辣汤", 40));
foodList.add(new Food("酸辣汤", 40));
foodList.add(new Food("酸辣汤", 40));
foodList.add(new Food("酸辣汤", 40));
selectedList.addAll(foodList);
listViewAdapter = new ListViewAdapter(selectedList,this);
listView.setAdapter(listViewAdapter);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
try {
int price = Integer.parseInt(editText.getText().toString());
selectedList.clear();
for (Food unit:foodList){
if(unit.getPrice() <= price){
selectedList.add(unit);
}
}
listViewAdapter.notifyDataSetChanged();
}catch (Exception e){
Toast.makeText(FoodListActivity.this,"请输入正确价格",Toast.LENGTH_SHORT).show();
}
}
});
}
}
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_marginTop="10dp"
android:id="@+id/head_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
>
<EditText
android:id="@+id/edit_text"
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:textSize="20sp"
android:hint="展示输入价格以下的食物"
/>
<Button
android:layout_marginLeft="10dp"
android:id="@+id/buttonfood"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="20sp"
android:text="确定"
/>
</LinearLayout>
<ListView
android:layout_below="@+id/head_layout"
android:id="@+id/list_view"
android:layout_marginTop="10dp"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:divider="@null"
>
</ListView>
</RelativeLayout>
package com.example.testapplication.adapter;
import android.content.Context;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.TextView;
import com.example.testapplication.R;
import com.example.testapplication.entity.Food;
import java.util.List;
public class ListViewAdapter extends BaseAdapter {
private List<Food> foodList;
private LayoutInflater layoutInflater;
public ListViewAdapter(List<Food> foodList, Context context){
this.foodList = foodList;
this.layoutInflater = LayoutInflater.from(context);
}
@Override
public int getCount() {
return foodList.size();
}
@Override
public Object getItem(int i) {
return null;
}
@Override
public long getItemId(int i) {
return 0;
}
@Override
public View getView(int i, View view, ViewGroup viewGroup) {
ViewHoler viewHoler;
if(view == null){
view = layoutInflater.inflate(R.layout.item_food,viewGroup,false);
viewHoler = new ViewHoler();
viewHoler.name = view.findViewById(R.id.name_tv);
viewHoler.price = view.findViewById(R.id.price_tv);
view.setTag(viewHoler);
}else{
viewHoler = (ViewHoler) view.getTag();
}
viewHoler.name.setText(foodList.get(i).getName());
viewHoler.price.setText(String.valueOf(foodList.get(i).getPrice()+"元"));
return view;
}
class ViewHoler{
TextView name;
TextView price;
}
}
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
android:paddingTop="10dp"
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:layout_marginLeft="10dp"
android:id="@+id/name_tv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="红烧肉"
android:textSize="32sp"/>
<TextView
android:layout_alignParentRight="true"
android:layout_marginRight="10dp"
android:id="@+id/price_tv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="100元"
android:textSize="32sp"/>
<View
android:layout_marginTop="10dp"
android:layout_width="match_parent"
android:layout_height="2dp"
android:layout_below="@id/name_tv"
android:background="@color/colorPrimary" />
</RelativeLayout>