欢迎点击: 个人官网博客
为了解决频繁触发DOM事件,项目中需要使用函数防抖或节流,我们来看看吧!
1.函数防抖:对于连续的事件响应我们只需要执行一次回调
就类似于在某个时间范围内,再次执行的话清除上一次执行
效果图:
应用场景:
1.每次 resize/scroll 触发统计事件
2.文本输入的验证,连续输入文字后发送 AJAX 请求进行验证,验证一次就好
demo代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<input type="text">
<script>
function antiShake(fn, wait) {
let timeOut = null;
return args => {
if (timeOut) clearTimeout(timeOut)
timeOut = setTimeout(fn, wait)
// 每次调用debounce函数都会将前一次的timer清空,确保只执行一次
}
}
function demo2() {
console.log('请求数据了')
}
let telInput = document.querySelector('input');
telInput.addEventListener('input', antiShake(demo2, 1000))
</script>
</body>
</html>
2.函数节流:一个函数执行一次后,只有大于设定的执行周期后才会执行第二次
一定时间内js方法只跑一次。让一个函数无法在短时间内连续调用,只有当上一次函数执行后,过了规定的时间间隔,才能进行下一次该函数的调用
效果图:
应用场景:(触发频率高)
扫描二维码关注公众号,回复:
13143886 查看本文章
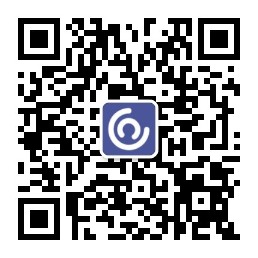
1.DOM 元素的拖拽功能实现(mousemove)
2.搜索联想(keyup)
3.计算鼠标移动的距离(mousemove)
4.Canvas 模拟画板功能(mousemove)
5.射击游戏的 mousedown/keydown 事件(单位时间只能发射一颗子弹)
6.监听滚动事件判断是否到页面底部自动加载更多:给 scroll 加了 debounce 后,只有用户停止滚动后,才会判断是否到了页面底部;如果是 throttle 的话,只要页面滚动就会间隔一段时间判断一次
demo代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<input type="text">
<script>
function demo2() {
console.log('请求数据了')
}
// 时间戳方案
function throttle(fn, wait) {
var pre = Date.now();
return function () {
var context = this;
var args = arguments;
var now = Date.now();
if (now - pre >= wait) {
fn.apply(context, args);
pre = Date.now();
}
}
}
// 定时器方案
function throttle2(fn, wait) {
var timer = null;
return function () {
var context = this;
var args = arguments;
if (!timer) {
timer = setTimeout(function () {
fn.apply(context, args);
timer = null;
}, wait)
}
}
}
let telInput = document.querySelector('input');
telInput.addEventListener('input', throttle2(demo2, 1000))
</script>
</body>
</html>