递归与回溯的不同:
递归是能够确定唯一解,向着一个方向不断深入
回溯则是有不同的决策,每一步都需要试探,需要根据状态决定是否回滚。
例题
439. 三元表达式解析器
https://leetcode-cn.com/problems/ternary-expression-parser/
Key point:
T的话取3-最后一个:之间的数据
F的话取最后一个:之后的数据
21. 合并两个有序链表
https://leetcode-cn.com/problems/merge-two-sorted-lists/
解法1:
Java自带API,sort
练习点:
链表的构造以及返回
/**
* Definition for singly-linked list.
* public class ListNode {
* int val;
* ListNode next;
* ListNode() {}
* ListNode(int val) { this.val = val; }
* ListNode(int val, ListNode next) { this.val = val; this.next = next; }
* }
*/
class Solution {
private ListNode buildFromList(List<Integer> list) {
ListNode result = new ListNode();
ListNode tmp = result;
for (int i = 0; i < list.size(); i++) {
ListNode next = null;
if(i != list.size() - 1) {
next = new ListNode();
}
tmp.val = list.get(i);
tmp.next = next;
tmp = next;
}
return result;
}
public ListNode mergeTwoLists(ListNode l1, ListNode l2) {
List<Integer> list = new LinkedList<>();
if (l1 == null) {
return l2;
}
if (l2 == null) {
return l1;
}
while (l1.next != null) {
list.add(l1.val);
l1 = l1.next;
}
list.add(l1.val);
while (l2.next != null) {
list.add(l2.val);
l2 = l2.next;
}
list.add(l2.val);
if (list.isEmpty()) {
return null;
}
list.sort(Comparator.naturalOrder());
return buildFromList(list);
}
}
解法2:递归
class Solution {
public ListNode mergeTwoLists(ListNode l1, ListNode l2) {
// 设置出口条件1:异常出口条件
if (l1 == null) {
return l2;
}
if (l2 == null) {
return l1;
}
// 正常业务逻辑
ListNode result = null;
if(l2.val > l1.val) {
// 本次处理部分
result = l1;
// 下次处理部分
result.next = mergeTwoLists(l1.next, l2);
} else {
result = l2;
result.next = mergeTwoLists(l1, l2.next);
}
// 设置出口条件2:正常出口条件
return result;
}
}
779. 第K个语法符号
暴力解法:
问题是:超时
原因:每次递归都会计算下一个字符串,这样导致的问题是,字符串2的倍数增长长度,N比较大时处理不掉。
解法:只需要得到解即可无需进行字符串替换
扫描二维码关注公众号,回复:
13007617 查看本文章
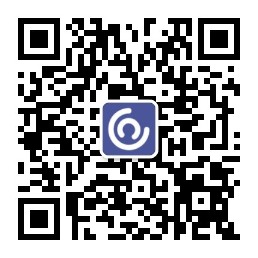
很容易理解,每行的第K个数字是由上一次产生的,我们只需要记录一位数字即可,而不需要反复每次求取字符串。