教程视频:Element UI + NodeJs(Express)全栈开发后台管理界面
1 目录结构
项目目录:
2 安装相应类库
2.1 element
官方文档:添加链接描述
安装:
// 方式一
npm i element-ui -S
// 方式二
// 使用如下方式安装element,则不需要再引入样式
vue add element
引入样式(完整引入):
在main.js中引入如下内容,
import Vue from 'vue';
import ElementUI from 'element-ui';
import 'element-ui/lib/theme-chalk/index.css';
import App from './App.vue';
Vue.use(ElementUI);
new Vue({
el: '#app',
render: h => h(App)
});
2.2 cors(后端跨域)
安装:
npm install cors
使用:
// app.js文件中引入,允许跨域
app.use(require('cors')()); // 允许跨域
【补充:node.js中利用cors解决跨域问题 ---- 安装、引入、使用】
安装模块:
npm install cors # 安装解决跨域模块
npm i axios # 用来发送请求
引入模块:
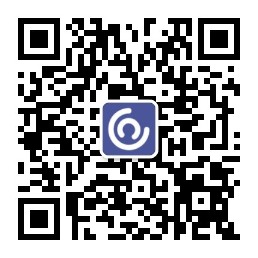
const cors = require('cors');
使用模块:
app.use(express.json()); # 解析json编码数据
app.use(express.urlencoded()); # 解析url编码的数据
app.use(cors()); # 不加上这句代码跨域访问时会出现错误,加上就不会出现跨域错误情况
参考博客:nodejs中利用cors解决跨域方法
2.3 axios(前端跨域)
安装:
npm install axios
使用(main.js文件中):
// 引入
import axios from 'axios'
// $ 是在Vue 所有实例中都可用的属性的一个简单约定。
// 需要设置全局变量,可以在main.js文件中通过 Vue.prototype 定义全局变量,
// 使其在每个Vue实例中可用。
Vue.prototype.$http = axios.create({
// 所以 $http 在所有Vue实例中可用
// axios.create() 根据指定配置创建一个新的 axios
baseURL:"http://localhost:3001/api" // 配置接口根地址
});
【补充:前后端数据调用】
axios的使用(三种方法):vue全局使用axios的方法
同时,注意一下vue的插件相关知识。
2.4 router
在创建项目的时候可以自动安装router,在App.vue文件中需要写入<router-view></router-view>
(一般创建项目的时候App.vue文件中自动写入),如下图,
在main.js中导入import router from './router
’路由并使用,如下图,
在index.js中设置路由的时候,需要导入相应的组件,如下图,
路由设置如下,
export default new Router({
routes: [
{
// 此处表示一打开网址所显示的页面,即首页默认展示页面
path: '/',
name: 'home',
redirect:'/articles/index'
},
{
// 文章列表页
path: '/articles/index',
name: 'list-article',
component:ListArtical
},
{
// 创建文章页
path: '/articles/create',
name: 'create-article',
component:CreateArtical
},
{
// 修改编辑文章页
path: '/articles/:id/edit',
name: 'edit-article',
component:EditArtical
}
]
})
3 vue中部分函数
3.1 methods()
用来定义template
模块中需要用到的函数。
3.2 created()
生命周期钩子函数,一般用于调用ajax获取页面初始化所需的数据。例如,
created(){
// 获取数据
this.$http.get('articles').then(res =>{
this.articles = res.data; // data表示真正的数据,上面template模块里面的:data
})
}
4 ES6的模版字符串
1)用`(反引号)标识,用${}将变量括起来;
2)在${}
中的大括号里可以放入任意的JavaScript表达式,还可以进行运算,以及引用对象属性;
3)模版字符串还可以调用函数。
saveArticle() {
// this.$http.put()里面的参数跟后端接口文件 app.js 里面的数据库操作函数的参数是一致的,
// 其中里面的put() 表示 app.js 中的提交方式
this.$http.put(`/articles/${
this.$route.params.id}`,this.article).then(res =>{
console.log(res.data);
this.$message({
message: '文章修改成功',
type: 'success'
});
this.$router.push('/articles/index'); // 实现页面跳转
})
}
5 this.$router.push({)实现路由跳转
push 后面可以是对象,也可以是字符串,如下,
// 字符串
this.$router.push('/home/first')
// 对象
this.$router.push({
path: '/home/first' })
// 命名的路由
this.$router.push({
name: 'home', params: {
userId: wise }})
<!--scope表示当前这一行的数据-->
<el-button @click="edit(scope.row._id)" type="text" size="small">编辑</el-button>
// 一般在点击事件里面使用
edit(id){
// 实现页面跳转
this.$router.push(`/articles/${
id}/edit`); // 此处为反引号(es6的模板字符串),跳转到对应ID的编辑页面
},
6 this.$ route.params和 this.$route.query
参考博客:vue之this.$ route.params和this.$route.query的区别
7 $route 和 $router
7.1 $route 表示 当前路由信息对象
表示当前激活的路由的状态信息,包含了当前 URL 解析得到的信息,还有 URL 匹配到的 route records(路由记录)。
路由信息对象:即$router会被注入每个组件中,可以利用它进行一些信息的获取。
**1.$route.path**
字符串,对应当前路由的路径,总是解析为绝对路径,如 "/foo/bar"。
**2.$route.params**
一个 key/value 对象,包含了 动态片段 和 全匹配片段,
如果没有路由参数,就是一个空对象。
**3.$route.query**
一个 key/value 对象,表示 URL 查询参数。
例如,对于路径 /foo?user=1,则有 $route.query.user == 1,
如果没有查询参数,则是个空对象。
**4.$route.hash**
当前路由的 hash 值 (不带 #) ,如果没有 hash 值,则为空字符串。锚点
**5.$route.fullPath**
完成解析后的 URL,包含查询参数和 hash 的完整路径。
**6.$route.matched**
数组,包含当前匹配的路径中所包含的所有片段所对应的配置参数对象。
**7.$route.name 当前路径名字**
**8.$route.meta 路由元信息
7.2 $router
参考博客:vue-router中$route 和 $router
全局的路由实例,是router构造方法的实例。
在 Vue 实例内部,你可以通过 $router 访问路由实例。
1)路由实例方法push
// 字符串
this.$router.push('home')
// 对象
this.$router.push({
path: 'home' })
// 命名的路由
this.$router.push({
name: 'user', params: {
userId: 123 }})
// 带查询参数,变成 /register?plan=123
this.$router.push({
path: 'register', query: {
plan: '123' }})
push方法其实和 <router-link :to="...">
是等同的。
注意:push方法的跳转会向 history 栈添加一个新的记录,当我们点击浏览器的返回按钮时可以看到之前的页面。
2)路由实例方法go
// 页面路由跳转 前进或者后退
this.$router.go(-1) // 后退
3)路由实例方法replace
//push方法会向 history 栈添加一个新的记录,而replace方法是替换当前的页面,
不会向 history 栈添加一个新的记录
<router-link to="/05" replace>05</router-link>
// 一般使用replace来做404页面
this.$router.replace('/')
8 req.params, req.query, req.body
参考博客:node中req.params,req.query,req.body三者的细微区别
// 指定ID获取数据
app.get('/api/articles/:id',async (req,res) =>{
var article = await Article.findById(req.params.id);
res.send(article);
});
9 解析json文件数据(post请求)
方式一:
// 配置body-parser,实现post接受数据
app.use(bodyParser.urlencoded({
extended:false}));
app.use(bodyParser.json());
方式二:
app.use(express.json());
9 前端实现路由跳转的关键
如图所示,