续上,我们已经掌握了自定义控件的基础知识(不清楚的自己去查看Android自定义控件(一)---基础篇),下面,我们来简单的写一个自定义 mTextView 控件(提醒一下:控件名字和属性名字最好不要和系统一样,容易报冲突错误),废话不多说,上码!!!
第一步:在 res 文件 下的 values 文件夹下新建一个名称为 attrs 的 attrs.xml 文件(这里可以改成别的文件名,但我觉得没必要,别闲的蛋疼)
第二步:在 attrs.xml 文件夹里自定义一些自己规划好的属性,这里我们就简单写几个
<?xml version="1.0" encoding="utf-8"?>
<resources>
<!-- name 最好是自定义view的名字 例如:mTextView -->
<declare-styleable name="mTextView">
<!-- name 属性名称 不要和系统冲突
format 格式 string 字符串
color 颜色
dimension 宽高 字体大小
integer 数字
reference 资源(drawable)
-->
<attr name="mTextSize" format="dimension"/>
<attr name="mTextColor" format="color"/>
<attr name="mText" format="string"/>
</declare-styleable>
</resources>
第三步:在布局文件中应用
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<com.example.mytextview.mTextView
android:layout_width="50dp"
android:layout_height="50dp"
android:background="@color/colorAccent"
app:mText="关注我,不迷路,哈哈哈,打了一波广告"
app:mTextSize="16dp"
app:mTextColor="#ff0000"/>
</LinearLayout>
第四步:新建一个 自定义控件的类,如 mTextView,在这个类里获取自定义属性值
package com.example.mytextview;
//import javax.swing.text.View;
import android.content.Context;
import android.content.res.TypedArray;
import android.graphics.Canvas;
import android.graphics.Color;
import android.util.AttributeSet;
import android.view.View;
import androidx.annotation.Nullable;
public class mTextView extends View {
//1、设置自定义属性变量
private int mTextSize = 16;
private int mTextColor = Color.RED;
private String mText;
public mTextView(Context context) {
this(context,null);
}
public mTextView(Context context, @Nullable AttributeSet attrs) {
this(context, attrs,0);
}
public mTextView(Context context, @Nullable AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
//2、获取装有自定义属性值的数值
TypedArray typedArray = context.obtainStyledAttributes(attrs, R.styleable.mTextView);
//3、精确获取自定义属性值
mTextSize = typedArray.getDimensionPixelSize(R.styleable.mTextView_mTextSize,mTextSize);//源码用的这个方法,参照 TextView
mTextColor = typedArray.getColor(R.styleable.mTextView_mTextColor,mTextColor);
mText = typedArray.getString(R.styleable.mTextView_mText);
//4、回收 typedArray
typedArray.recycle();
}
//5、测量
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
super.onMeasure(widthMeasureSpec, heightMeasureSpec);
}
//6、绘制
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
}
}
写到这里,已经成功了一大半,但是,如果你将项目运行起来,会发现什么也没有,因为你还没有 测量(onMeasure)和绘制(onDraw),后续我们会详细阐述这两个方法。
扫描二维码关注公众号,回复:
12558680 查看本文章
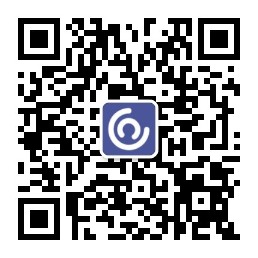