题目链接:https://atcoder.jp/contests/abc188/tasks
A - Three-Point Shot
题目大意
给你x,y问你较小值加3能否超过较大值
思路
直接判断
ac代码
#include<bits/stdc++.h>
using namespace std;
#define ll long long
int main(){
int x, y;
cin >> x >> y;
if(x > y) swap(x, y);
if(x + 3 > y) cout << "Yes" << endl;
else cout << "No" << endl;
return 0;
}
B - Orthogonality
题目大意
两个数组,对应位置相乘 求和 看是否等于0
思路
直接模拟
ac代码
#include<bits/stdc++.h>
using namespace std;
#define ll long long
const int maxn = 1e5 + 5;
int a[maxn], b[maxn];
int main(){
int n; cin >> n;
for(int i = 1; i <= n; i ++) cin >> a[i];
for(int i = 1; i <= n; i ++) cin >> b[i];
ll ans = 0;
for(int i = 1; i <= n; i ++) ans += a[i] * b[i];
if(ans == 0) cout << "Yes" << endl;
else cout << "No" << endl;
return 0;
}
C - ABC Tournament
题目大意
给你一个n,然后2^n个数,表示第i个人的分数,每轮相邻两位pk,分数高的获胜,进入下一轮,输出最终亚军的初始下标
思路
vector模拟
ac代码
#include<bits/stdc++.h>
using namespace std;
#define ll long long
const int maxn = 1e5 + 5;
vector<pair<int, int>> a, b;
int main(){
int n; cin >> n;
for(int i = 1; i <= (1 << n); i ++) {
int x; cin >> x;
a.push_back({x, i});
}
for(int i = 1; i < n; i ++){
for(int j = 0; j < a.size(); j += 2){
if(a[j].first > a[j + 1].first) b.push_back(a[j]);
else b.push_back(a[j + 1]);
}
a = b;
b.clear();
}
if(a[0].first > a[1].first) cout << a[1].second << endl;
else cout << a[0].second << endl;
return 0;
}
D - Snuke Prime
题目大意
Takahashi 出去游玩,现在提供了n个项目,时间是[ai, bi],这些项目每天分别需要花费ci,但它可以选择一天花费C元玩这天出现的所有项目,项目出现了他就一定要玩,求他的最少花费
比如第一个样例,2个项目,一天玩所有出现的项目花费6,第一个项目出现在[1,2],每天花费4,第二个项目出现在[2,2],每天花费4,也就是说第一天需要花费4,第二天需要花4 + 4=8,但是8<6可以选择花6块钱玩项目1,2,所以一共花费4+6=10
思路
差分求该天单付每个项目的花费,然后每天的花费取当前天的花费与C的较小值
ac代码
#include<bits/stdc++.h>
using namespace std;
#define ll long long
const int maxn = 2e5 + 5;
map<int, ll> mp; // 差分数组
//s表示差分的前缀和,表示到这天单付当天所有项目的花费
//last表示一个天数,(it.first - last)表示s价格出现的天数,也就是在这个期间价格不变
//x表示当天花的钱
//ans表示求的最小花费
int main(){
ll n, c;
cin >> n >> c;
for(int i = 1; i <= n; i ++){
ll x, y, w;
cin >> x >> y >> w;
mp[x] += w; mp[y + 1] -= w; // 差分操作
}
ll s = 0, ans = 0;
int last = 0;
for(auto it : mp){
ll x = min(s, c);
ans += x * (it.first - last);
s += it.second;
last = it.first;
}
cout << ans << endl;
return 0;
}
E - Peddler
题目大意
第一行n,m表示n个点m条边,
扫描二维码关注公众号,回复:
12451826 查看本文章
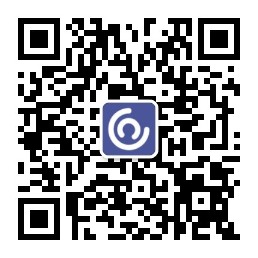
第二行n个ai,表示该点黄金的价格,
接下来m行表示边,每条边是有向边,其中xi一定小于yi
现在他要选一个点买黄金,然后走到另外一个点卖黄金,求最大利润。
思路
拓扑序列上递推答案,dp[i]表示从源点(不知道那个点)跑到点i途径点的黄金最便宜的价钱,由于是单向边,那么这个点肯定不会往回跑,只会往下跑,那么可以线性递推,然后只需要更新a[son]-dp[i]的最大值即可,son是i的子节点
ac代码
#include<bits/stdc++.h>
using namespace std;
#define ll long long
const int maxn = 2e5 + 5;
int dp[maxn], in[maxn], a[maxn];
vector<int> v[maxn];
queue<int> q;
int main(){
int n, m;
cin >> n >> m;
for(int i = 1; i <= n; i ++) cin >> a[i];
while(m --){
int x, y;
cin >> x >> y;
v[x].push_back(y);
in[y] ++;
}
for(int i = 1; i <= n; i ++){
if(in[i] == 0) q.push(i);
dp[i] = a[i]; //刚开始只初始化入度为0的节点然后wa了7个点,原因是存在2 3, 1 3种情况,也就是说这个图有可能是发散的,必须在跑到他之前就要初始化
}
int ans = -0x3f3f3f3f;
while(q.size()){
int t = q.front(); q.pop();
for(auto it : v[t]){
if(ans == -0x3f3f3f3f || ans < a[it] - dp[t]) ans = a[it] - dp[t];
dp[it] = min(dp[it], dp[t]);
if(--in[it] == 0) q.push(it);
}
}
cout << ans << endl;
return 0;
}
F - +1-1x2
题目大意
给你两个数x,y,每次对一个数都可以进行 +1 或者 -1 或者 *2 ,问你最少需要操作几次,可以把x变成y
思路
记忆化搜索,dfs(y)表示将x变成y需要的步数
具体看代码
ac代码
#include<bits/stdc++.h>
using namespace std;
#define ll long long
const int maxn = 2e5 + 5;
map<ll, ll> dp; //dp[y]表示将x变成y需要的步数
ll x, y;
ll dfs(ll yy){
if(yy <= x) return x - yy; //如果x大于等于yy,那么x只能进行x-yy次 减操作到达yy
if(dp.count(y)) return dp[yy]; //记忆化操作直接返回
ll res = yy - x; //表示x进行yy-x次 加操作到达y
if(yy % 2 == 0) res = min(res, dfs(yy / 2) + 1); //如果yy是偶数,那么就存在乘2的操作,使得x到达yy
else res = min(res, 1 + min(dfs(yy + 1), dfs(yy - 1))); //不然就看看加1减1操作使得x到达yy,取一个最小值
return dp[yy] = res; //记忆化存
}
int main(){
cin >> x >> y;
cout << dfs(y);
return 0;
}