文章目录
codewars-js练习
2021/1/25
github 地址
【1】<8kyu>【Quarter of the year】
Given a month as an integer from 1 to 12, return to which quarter of the year it belongs as an integer number.
For example: month 2 (February), is part of the first quarter; month 6 (June), is part of the second quarter; and month 11 (November), is part of the fourth quarter.
给定一个月作为从1到12的整数,以整数形式返回它所属的季度。
example:
quarterOf(3)// 1
quarterOf(8)//3
quarterOf(11)//4
solution:
<script type="text/javascript">
const quarterOf = (month) => {
switch(month>0&& month<13){
case month>0 && month<=3:
// console.log('1');
return 1;
break;
case month>3 && month<=6:
// console.log('2');
return 2;
break;
case month>6 && month<=9:
// console.log('3');
return 3;
break;
case month>9 && month<=12:
// console.log('4');
return 4;
break;
default:
break;
}
}
// 验证
console.log(quarterOf(3));// 1
console.log(quarterOf(8)); //3
</script>
【2】<6kyu>【Detect Pangram】
A pangram is a sentence that contains every single letter of the alphabet at least once. For example, the sentence “The quick brown fox jumps over the lazy dog” is a pangram, because it uses the letters A-Z at least once (case is irrelevant).
Given a string, detect whether or not it is a pangram. Return True if it is, False if not. Ignore numbers and punctuation.
pangram是至少包含一次字母表中每一个字母的句子。即a-z都含有
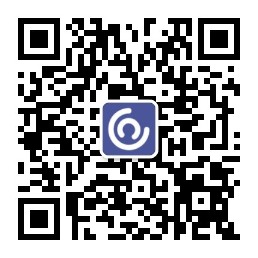
example:
var string = "The quick brown fox jumps over the lazy dog."
Test.assertEquals(isPangram(string), true)
var string = "This is not a pangram."
Test.assertEquals(isPangram(string), false)
思路
- 先将字符串全部转为大写
- 65-90代表a-z,则循环将65-90转换成a-z;
- 用indexOf进行判断,如果为-1,则说明不存在。
solution:
<script type="text/javascript">
function isPangram(string){
// 先将字符串全部转为大写
var str = string.toUpperCase();
// 65-90是a-z
for(var i=65;i<90;i++){
if(str.indexOf(String.fromCharCode(i)) == -1){
return false;
}
}
return true;
}
// 验证
var string = "The quick brown fox jumps over the lazy dog."
console.log(isPangram(string));//true
var string2 = "This is not a pangram."
console.log(isPangram(string2));//false
</script>
【3】<7kyu>【Descending Order】
Your task is to make a function that can take any non-negative integer as an argument and return it with its digits in descending order. Essentially, rearrange the digits to create the highest possible number.
降序排序
example:
Input: 42145 Output: 54421
Input: 145263 Output: 654321
Input: 123456789 Output: 987654321
solution:
<script type="text/javascript">
function descendingOrder(n){
// 先将n转换成数组
var arr = n.toString().split('');
// 进行降序排序
arr.sort((a,b)=>{
return b-a});
// console.log(arr);
// 返回成number类型
var result = parseInt(arr.join(''));
// console.log(result);
return result;
}
// 验证
console.log(descendingOrder(42145));//54421
console.log(descendingOrder(145263));//654321
</script>
【4】<7kyu>【Highest and Lowest】
In this little assignment you are given a string of space separated numbers, and have to return the highest and lowest number.
返回最大、最小值
example:
highAndLow("1 2 3 4 5"); // return "5 1"
highAndLow("1 2 -3 4 5"); // return "5 -3"
highAndLow("1 9 3 4 -5"); // return "9 -5"
solution
<script type="text/javascript">
function highAndLow(numbers){
var numStr = numbers.split(' ');
// 降序排序
numStr.sort((a,b)=>{
return b-a;});
// 返回第一个(最大值)和最后一个(最小值)
return numStr[0] +' '+numStr[numStr.length-1];;
}
// 验证
console.log(highAndLow("1 2 3 4 5"));//'5 1'
console.log(highAndLow("1 2 -3 4 5")); // "5 -3"
</script>
【5】<7kyu>【Sum of all the multiples of 3 or 5】
Upto and including n
, this function will return the sum of all multiples of 3 and 5.
查找到n为止所有满足3的倍数或者5的倍数,然后返回这些数的和
example:
findSum(5) should return 8 (3 + 5)
findSum(10) should return 33 (3 + 5 + 6 + 9 + 10)
solution
<script type="text/javascript">
function findSum(n) {
var sum = 0;
// 遍历找到所有3或5的倍数,再相加返回
for(var i=1;i<=n;i++){
if(i % 3==0 || i %5 ==0){
// console.log(i);
sum = sum+i;
}
}
// console.log('sum:',sum);
return sum;
}
// 验证
console.log(findSum(5));//8
console.log(findSum(10)); // 33
</script>
【6】<6kyu>【Counting Duplicates】
Write a function that will return the count of distinct case-insensitive alphabetic characters and numeric digits that occur more than once in the input string. The input string can be assumed to contain only alphabets (both uppercase and lowercase) and numeric digits.
**Count the number of Duplicates **
example:
"abcde" -> 0 //no characters repeats more than once
"aabbcde" -> 2 # 'a' and 'b'
"aabBcde" -> 2 # 'a' occurs twice and 'b' twice (`b` and `B`)
"indivisibility" -> 1 # 'i' occurs six times
"Indivisibilities" -> 2 # 'i' occurs seven times and 's' occurs twice
"aA11" -> 2 # 'a' and '1'
"ABBA" -> 2 # 'A' and 'B' each occur twice
思路
- 先将text全部转为小写;然后分隔放到数组中
- 对数组进行sort排序(sort排序是按照unicode编码进行排序)
- 利用正则表达式,将相同的合并,然后作为一个整体放在数组中
- 最后读取数组的长度即可
solution
<script type="text/javascript">
function duplicateCount(text){
// 先将text全部转为小写;然后分隔放到数组中,然后进行sort排序
var textArrAfterSort = text.toLowerCase().split('').sort();
// console.log(textArrAfterSort);
var newText = textArrAfterSort.join('');
// console.log(newText);
// 正则表达式匹配
var result = newText.match(/([^])\1+/g);
// console.log(result);
return (result|| []).length;
}
// 验证
console.log(duplicateCount("abcde"));//0
console.log(duplicateCount('aabbcde')); // 2
console.log(duplicateCount('indivisibility'));//1
</script>
以上为自己思路供大家参考,可能有更优的思路。