文章目录
codewars-js练习
2021/1/21
github 地址
【1】<8kyu>【N-th Power】
You are given an array with positive numbers and a non-negative number N. You should find the N-th power of the element in the array with the index N. If N is outside of the array, then return -1. Don’t forget that the first element has the index 0.
example:
array = [1, 2, 3, 4] and N = 2, then the result is 3^2 == 9;
array = [1, 2, 3] and N = 3, but N is outside of the array, so the result is -1.
solution:
<script type="text/javascript">
function index(array, n){
var length = array.length;
if(n>=length){
return -1;
}else{
for(var i=0;i<length;i++){
if(i == n){
return Math.pow(array[i],n);
}
}
}
}
// 验证
console.log(index([1, 2, 3, 4],2));//9
console.log(index([1, 2],3));//-1
</script>
【2】<8kyu>【Aspect Ratio Cropping - Part 1】
Write a function that accepts arbitrary X and Y resolutions and converts them into resolutions with a 16:9 aspect ratio that maintain equal height. Round your answers up to the nearest integer.
example:
aspectRatio(640, 480)//[854,480]
aspectRatio(960,720)//[1280,720]
solution:
<script type="text/javascript">
function aspectRatio(x,y){
var width = Math.ceil(y*16/9);
var result = [width,y];
// console.log(result);
return result;
}
// 验证
console.log(aspectRatio(640, 480));//[854,480]
console.log(aspectRatio(960,720));//[1280,720]
</script>
【3】<8kyu>【Grasshopper - Basic Function Fixer】
I created this function to add five to any number that was passed in to it and return the new value. It doesn’t throw any errors but it returns the wrong number.
solution:
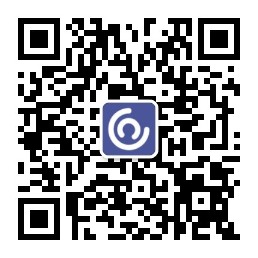
<script type="text/javascript">
function addFive(num) {
var total = num + 5;
return total
}
// 验证
console.log(addFive(5));//10
</script>
【4】<8kyu>【Convert a Boolean to a String】
Implement a function which convert the given boolean value into its string representation.
example:
booleanToString(true)//'true'
solution:
<script type="text/javascript">
function booleanToString(b){
if(typeof b == 'boolean'){
// console.log(typeof String(b));
return String(b);
}
}
// 验证
console.log(booleanToString(true));//'true'
</script>
以上为自己思路供大家参考,可能有更优的思路。