题意:给定一个字符串s,问在字符串右边最少添加多少个字符可以s成为一个回文串。
KMP做法:
将原串reverse后为t,t和s进行匹配,最后匹配上的长度就是两者可以重叠的部分,最后字符串长度*2再减去重叠部分长度。
#include <iostream>
#include <cstdio>
#include <algorithm>
#include <queue>
#include <cmath>
#include <string>
#include <vector>
#include <stack>
#include <map>
#include <sstream>
#include <cstring>
#include <set>
#include <cctype>
#include <bitset>
#define IO \
ios::sync_with_stdio(false); \
// cin.tie(0); \
// cout.tie(0);
using namespace std;
typedef long long LL;
const int maxn = 1e6 + 100;
const int maxm = 1e6 + 10;
const LL INF = 0x3f3f3f3f3f3f3f3f;
const int inf = 0x3f3f3f3f;
const LL mod = 1e9 + 7;
int dis[8][2] = {0, 1, 1, 0, 0, -1, -1, 0, 1, -1, 1, 1, -1, 1, -1, -1};
string s, p;
int nextp[maxn];
void Get_nextp(int n)
{
for (int i = 1; i < n; i++)
{
int j = i;
while (j > 0)
{
j = nextp[j];
if (p[i] == p[j])
{
nextp[i + 1] = j + 1;
break;
}
}
}
}
int Kmp(int n)
{
int i, j;
for (i = 0, j = 0; i < n; i++)
{
if (j < n && s[i] == p[j])
j++;
else
{
while (j > 0)
{
j = nextp[j];
if (s[i] == p[j])
{
j++;
break;
}
}
}
}
return j;
}
int main()
{
#ifdef ONLINE_JUDGE
#else
freopen("in.txt", "r", stdin);
// freopen("out.txt", "w", stdout);
#endif
IO;
int T, n;
int kase = 0;
cin >> T;
while (T--)
{
cin >> s;
p = s;
reverse(p.begin(), p.end());
// cout << s << endl;
// cout << p << endl;
n = s.size();
Get_nextp(n);
cout << "Case " << ++kase << ": " << n + n - Kmp(n) << endl;
}
return 0;
}
Mancher做法:由题目可知,我们可以求从字符串右端点向左延伸的最长回文串长度,然后再在字符串右端添加左边不是回文串的部分即可。mancher算法从左往右,找到一个位置,这个位置的回文串可以延伸到右端点,然后返回这个回文串长度,对于这个mancher最后返回的地方为什么这么写,还有点不太明白。。。o(╥﹏╥)o
light oj 还因为数组开的太大(1e7)莫名RE。。o(╥﹏╥)o
#include <iostream>
#include <cstdio>
#include <algorithm>
#include <queue>
#include <cmath>
#include <string>
#include <vector>
#include <stack>
#include <map>
#include <sstream>
#include <cstring>
#include <set>
#include <cctype>
#include <bitset>
#define IO \
ios::sync_with_stdio(false); \
// cin.tie(0); \
// cout.tie(0);
using namespace std;
typedef long long LL;
const int maxn = 2e6 + 100;
const int maxm = 1e6 + 10;
const LL INF = 0x3f3f3f3f3f3f3f3f;
const int inf = 0x3f3f3f3f;
const LL mod = 1e9 + 7;
int dis[8][2] = {0, 1, 1, 0, 0, -1, -1, 0, 1, -1, 1, 1, -1, 1, -1, -1};
char s[maxn];
char t[maxn];
int len[maxn];
int init(char *s)
{
int len = strlen(s);
t[0] = '@';
for (int i = 1; i <= 2 * len; i += 2)
{
t[i] = '#';
t[i + 1] = s[i / 2];
}
t[2 * len + 1] = '#';
t[2 * len + 2] = '$';
t[2 * len + 3] = 0;
return 2 * len + 1;
}
int mancher(char *s, int n)
{
int mx = 0;
int ans = 0;
int pos = 0;
for (int i = 1; i <= n; i++)
{
if (mx > i)
len[i] = min(mx - i, len[2 * pos - i]);
else
len[i] = 1;
while (s[i - len[i]] == s[i + len[i]])
{
len[i]++;
}
if (len[i] + i > mx)
{
mx = len[i] + i;
pos = i;
}
if (len[i] + i >= n)
return n - i;
}
}
int main()
{
#ifdef ONLINE_JUDGE
#else
freopen("in.txt", "r", stdin);
// freopen("out.txt", "w", stdout);
#endif
// IO;
int T, n;
int kase = 0;
scanf("%d", &T);
while (T--)
{
scanf("%s", s);
int n = strlen(s);
int len = init(s); // 返回的是T字符串的长度
printf("Case %d: %d\n", ++kase, 2 * n - mancher(t, len));
}
return 0;
}
扫描二维码关注公众号,回复:
11953931 查看本文章
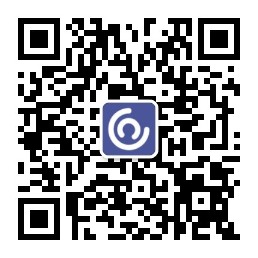