安装依赖
yarn add ol
百度组件
import React from "react";
import "ol/ol.css";
import Feature from "ol/Feature";
import Map from "ol/Map";
import View from "ol/View";
import Point from "ol/geom/Point";
import { Tile as TileLayer, Vector as VectorLayer } from "ol/layer";
import { Cluster, Vector as VectorSource } from "ol/source";
import { Circle as CircleStyle, Fill, Stroke, Style, Text } from "ol/style";
import { TileImage } from "ol/source";
import TileGrid from "ol/tilegrid/TileGrid";
export default class MyBdMap extends React.Component {
constructor(props) {
super(props);
this.state = { opacity: 1.0 };
}
componentDidMount() {
this.makeMap();
}
makeMap = () => {
var distance = document.getElementById("distance");
var count = 20;
var features = new Array(count);
var e = 4500000;
for (var i = 0; i < count; ++i) {
var coordinates = [2 * e * Math.random() - e, 2 * e * Math.random() - e];
console.log(coordinates);
features[i] = new Feature({
geometry: new Point(coordinates),
});
}
var source = new VectorSource({
features: features,
});
var clusterSource = new Cluster({
distance: parseInt(distance.value, 10),
source: source,
});
var styleCache = {};
var clusters = new VectorLayer({
source: clusterSource,
style: function (feature) {
debugger;
var size = feature.get("features").length;
var style = styleCache[size];
if (!style) {
style = new Style({
image: new CircleStyle({
radius: 20,
stroke: new Stroke({
color: "#fff",
}),
fill: new Fill({
color: "#AA99CC",
}),
}),
text: new Text({
text: size.toString(),
fill: new Fill({
color: "#fff",
}),
}),
});
styleCache[size] = style;
}
return style;
},
});
let resolutions = [];
let baiduX, baiduY;
for (let i = 0; i < 19; i++) {
resolutions[i] = Math.pow(2, 18 - i);
}
let tilegrid = new TileGrid({
origin: [0, 0],
resolutions: resolutions,
});
let baidu_source = new TileImage({
projection: "EPSG:3857",
tileGrid: tilegrid,
tileUrlFunction: function (tileCoord) {
if (!tileCoord) return "";
let z = tileCoord[0];
let x = tileCoord[1];
let y = tileCoord[2];
// 对编号xy处理
baiduX = x < 0 ? x : "M" + -x;
baiduY = -y;
return (
"http://online3.map.bdimg.com/onlinelabel/?qt=tile&x=" +
baiduX +
"&y=" +
baiduY +
"&z=" +
z +
"&styles=pl&udt=20151021&scaler=1&p=1"
);
},
});
var raster = new TileLayer({
source: baidu_source,
});
var map = new Map({
layers: [raster, clusters],
target: "map",
view: new View({
projection: "EPSG:3857",
center: [13531290.967373039, 4675318.865056401],
zoom: 3,
}),
});
distance.addEventListener("input", function () {
clusterSource.setDistance(parseInt(distance.value, 10));
});
map.on("select", function (e) {
var features = e.target.getFeatures().getArray();
if (features.length > 0) {
var data = features[0].values_.data;
if (data.callback) {
data.callback(e, data.callbackParams);
}
}
});
map.on("click", function (evt) {
var pixel = map.getEventPixel(evt.originalEvent);
console.log("点击的图像位置为:" + pixel);
var feature = map.forEachFeatureAtPixel(evt.pixel, function (feature) {
return feature;
});
if (feature) {
debugger;
var coordinates = feature.getGeometry().getCoordinates();
console.log("点击的坐标位置为:" + coordinates);
}
});
};
render() {
return (
<div>
<form>
<label>cluster distance</label>
<input
id="distance"
type="range"
min="0"
max="100"
step="1"
value="40"
/>
</form>
<div
id="map"
class="map"
style={
{ width: "1200px", height: "600px" }}
></div>
</div>
);
}
}
实现效果
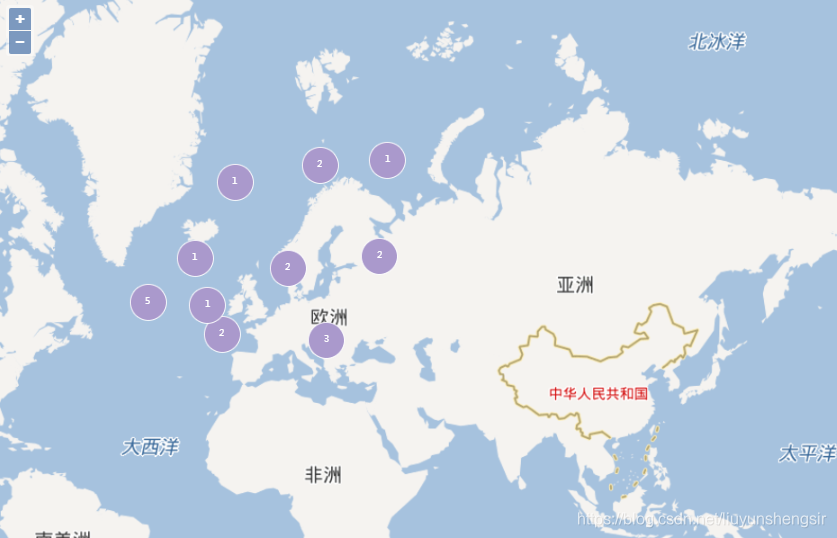