java发送http请求的两种方式:HTTPClient和CloseableHttpClient和swagger接口的一些注解的认识
java发送http请求有三种方式,除了原生连接方式HttpURLConnection,还有另外两种方式:HTTPClient和CloseableHttpClient
下面分别简单介绍使用HTTPClient和CloseableHTTPClient进行Get和Post请求的方式。
详情使用链接
HttpClient
使用commons-httpclient.jar,maven依赖如下:
<!-- https://mvnrepository.com/artifact/commons-httpclient/commons-httpclient -->
<dependency>
<groupId>commons-httpclient</groupId>
<artifactId>commons-httpclient</artifactId>
<version>3.1</version>
</dependency>
简单代码如下:
private static String doGet(String url) {
String res = null;
HttpClient client = new HttpClient();
GetMethod getMethod = new GetMethod(url);
int code = 0;
try {
code = client.executeMethod(getMethod);
if (code == 200) {
res = getMethod.getResponseBodyAsString();
}
} catch (IOException e) {
e.printStackTrace();
}
return res;
}
private static String doPost(String url, Map<String, Object> paramMap) {
String res = null;
HttpClient client = new HttpClient();
PostMethod postMethod = new PostMethod(url);
postMethod.getParams().setContentCharset("UTF-8");
Iterator<Map.Entry<String, Object>> iterator = paramMap.entrySet().iterator();
while (iterator.hasNext()) {
Map.Entry<String, Object> next = iterator.next();
postMethod.addParameter(next.getKey(), next.getValue().toString());
}
try {
int code = client.executeMethod(postMethod);
if (code == 200) {
res = postMethod.getResponseBodyAsString();
}
} catch (IOException e) {
e.printStackTrace();
}
return res;
}
public static void main(String[] args) {
//get
System.out.println(doGet("http://localhost:8080/hello"));
//post
//设置传入参数的格式:请求参数是 map 的形式
Map<String, Object> paramMap = new HashMap<>(2);
paramMap.put("name", "赵云");
paramMap.put("age", 21);
System.out.println(doPost("http://localhost:8080/hello1", paramMap));
}
被调用方法:
测试结果:
CloseableHttpClient
使用httpclient.jar,maven依赖如下:
<!-- https://mvnrepository.com/artifact/org.apache.httpcomponents/httpclient -->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.12</version>
</dependency>
简单代码如下:
/**
* 发送HttpGet请求
* @param url
* @return
*/
public static String doGet(String url) {
//1.获得一个httpclient对象
CloseableHttpClient httpclient = HttpClients.createDefault();
//2.生成一个get请求
HttpGet httpget = new HttpGet(url);
CloseableHttpResponse response = null;
try {
//3.执行get请求并返回结果
response = httpclient.execute(httpget);
} catch (IOException e1) {
e1.printStackTrace();
}
String result = null;
try {
//4.处理结果,这里将结果返回为字符串
HttpEntity entity = response.getEntity();
if (entity != null) {
result = EntityUtils.toString(entity);
}
} catch (ParseException | IOException e) {
e.printStackTrace();
} finally {
try {
response.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return result;
}
/**
* 发送HttpPost请求,参数为map
* @param url
* @param map
* @return
*/
public static String doPost(String url, Map<String, Object> map) {
CloseableHttpClient httpclient = HttpClients.createDefault();
List<NameValuePair> formparams = new ArrayList<NameValuePair>();
for (Map.Entry<String, Object> entry : map.entrySet()) {
//给参数赋值
formparams.add(new BasicNameValuePair(entry.getKey(), String.valueOf(entry.getValue())));
}
UrlEncodedFormEntity entity = new UrlEncodedFormEntity(formparams, Consts.UTF_8);
HttpPost httppost = new HttpPost(url);
httppost.setEntity(entity);
CloseableHttpResponse response = null;
try {
response = httpclient.execute(httppost);
} catch (IOException e) {
e.printStackTrace();
}
HttpEntity entity1 = response.getEntity();
String result = null;
try {
result = EntityUtils.toString(entity1);
} catch (ParseException | IOException e) {
e.printStackTrace();
}
return result;
}
public static void main(String[] args) {
//get
System.out.println(doGet("http://localhost:8080/hello"));
//post
//设置传入参数的格式:请求参数是 map 的形式
Map<String, Object> paramMap = new HashMap<>(2);
paramMap.put("name", "张飞");
paramMap.put("age", 55);
System.out.println(doPost("http://localhost:8080/hello1", paramMap));
}
被调用方法:
测试结果:
swagger是当前最好用的Restful API文档生成的开源项目,通过swagger-spring项目实现了springMVC框架的无缝集成功能,方便生成restful风格的接口文档,
同时,swagger-ui还可以测试spring restful风格的接口功能
作用范围 | API | 使用位置 |
---|---|---|
对象属性 | @ApiModelProperty | 用在参数对象的字段上 |
协议集描述 | @Api | 用在Conntroller类上 |
协议描述 | @ApiOperation | 用在controller方法上 |
Response集 | @ApiResponses | 用在controller方法上 |
Response | @ApiResponse | 用在@ApiResponses里面 |
非对象参数集 | @ApilmplicitParams | 用在controller方法上 |
非对象参数描述 | @ApiImplicitParam | 用在@ApiImplicitParams的方法里边 |
描述返回对象的意义 | @ApiModel | 用在返回对象类上 |
@Api:用在请求的类上,表示对类的说明
tags=“说明该类的作用,可以在UI界面上看到的注解”
value=“该参数没什么意义,在UI界面上也看到,所以不需要配置”
@ApiOperation:用在请求的方法上,说明方法的用途、作用
value=“说明方法的用途、作用”
notes=“方法的备注说明”
@ApiImplicitParams:用在请求的方法上,表示一组参数说明
@ApiImplicitParam:用在@ApiImplicitParams注解中,指定一个请求参数的各个方面
name:参数名
value:参数的汉字说明、解释
required:参数是否必须传
paramType:参数放在哪个地方
· header --> 请求参数的获取:@RequestHeader
· query --> 请求参数的获取:@RequestParam
· path(用于restful接口)–> 请求参数的获取:@PathVariable
· body(不常用)
· form(不常用)
dataType:参数类型,默认String,其它值dataType=“Integer”
defaultValue:参数的默认值
@ApiResponses:用在请求的方法上,表示一组响应
@ApiResponse:用在@ApiResponses中,一般用于表达一个错误的响应信息
code:数字,例如400
message:信息,例如"请求参数没填好"
response:抛出异常的类
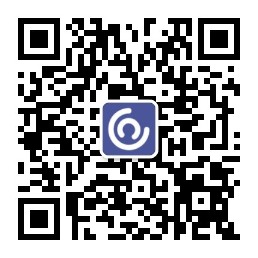
@ApiModel:用于响应类上,表示一个返回响应数据的信息
(这种一般用在post创建的时候,使用@RequestBody这样的场景,
请求参数无法使用@ApiImplicitParam注解进行描述的时候)
@ApiModelProperty:用在属性上,描述响应类的属性