4.1、Object类
描述:该类是所有类的最终根类
方法 | 描述 |
---|---|
public boolean equals(Object obj) | 表示某个其它对象是否“等于”此对象 |
public final class<?> getClass() | 返回此Object的运行时类 |
public int hashCode() | 返回对象的哈希码值 |
public String toString() | 返回对象的字符串表示形式 |
4.2、System类
描述:该类包含几个有用的类字段和方法
方法 | 描述 |
---|---|
public static long currentTimeMillis() | 以毫秒为单位返回当前时间 |
public static void exit(int status) | 终止当前运行的Java虚拟机 |
public static void gc() | 运行垃圾收集器 |
public static String lineSeparator() | 返回依赖于系统的行分隔符字符串 |
4.3、Arrays类
描述:该类包含用于操作数组的各种方法
方法 | 描述 |
---|---|
public static List asList(T… a) | 描述:返回由指定数组支持的固定大小的列表 举例:List stooges = Arrays.asList(“Larry”, “Moe”, “Curly”); |
public static String toString(int[] a) | 描述:返回指定数组的内容的字符串表示形式 举例: int[] a = { 10, 20, 30, 4, 5, 60, 70, 80 }; String as = Arrays.toString(a); |
public static void sort(int[] a) | 描述:按照数字升序顺序排列指定的数组 举例: int[] a = { 10, 20, 30, 4, 5, 60, 70, 80 }; Arrays.sort(a); |
public static void sort(int[] a, int fromIndex, int toIndex) | 描述:按照数字升序顺序对数组的指定范围进行排序 举例: int[] a = { 10, 20, 30, 4, 5, 60, 70, 80 }; Arrays.sort(a, 2, 7); |
public static int binarySearch(int[] a, int key) | 描述:使用二分查找算法在指定的int数组中搜索指定的值 举例: int[] a = { 10, 20, 30, 4, 5, 60, 70, 80 }; Arrays.sort(a); int index = Arrays.binarySearch(a, 5); |
public static int binarySearch(int[] a, int fromIndex, int toIndex, int key) | 描述:使用二分查找算法在指定的int数组中的指定范围搜索指定的值 举例: int[] a = { 10, 20, 30, 4, 5, 60, 70, 80 }; Arrays.sort(a); int index = Arrays.binarySearch(a, 2, 7, 60); |
4.4、Math类
描述:该类包含执行基本数字运算的方法,如基本指数,对数,平方根和三角函数
成员变量:
变量 | 描述 |
---|---|
public static final double E | 比其它任何一个更接近 e ,自然对数的基数 |
public static final double PI | 比其它任何一个更接近 pi ,圆周长与其直径的比率 |
成员方法:
方法 | 描述 |
---|---|
public static int abs(int a) | 返回值为int绝对值 |
public static int max(int a, int b) | 返回两个int的较大值 |
public static int min(int a, int b) | 返回两个int的较小值 |
public static double floor(double a) | 返回小于或等于参数的最大整数 |
public static double ceil(double a) | 返回大于或等于参数的最小整数 |
public static long round(double a) | 返回四舍五入后的整数值 |
public static double pow(double a, double b) | 返回a的b次幂 |
public static double log(double a) | 返回log以e为底的值 |
public static double sqrt(double a) | 返回a的正平方根 |
public static double random() | 返回一个[0.0 , 1.0)之间的随机数 public static int getRand(double min, double max) { return (int) (Math.random() * (max - min + 1) + min); } |
4.6、Date类
描述:该类是一个日期类
构造方法:
方法 | 描述 |
---|---|
public Date() | 构造一个 Date对象,它代表当前的毫秒值 |
public Date(long date) | 使用给定的毫秒时间值构造一个Date对象 |
4.7、SimpleDateFormat类
1、将日期格式化为字符串
扫描二维码关注公众号,回复:
11597502 查看本文章
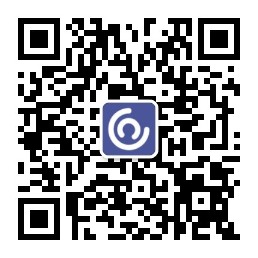
Date d = new Date();
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd hh:mm:ss");
String s = sdf.format(d);
2、将字符串格式化为日期
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd hh:mm:ss");
Date d = sdf.parse("2020-07-15 01:02:03");
4.8、Calendar类
Calendar c = Calendar.getInstance();
int year = c.get(Calendar.YEAR);
int month = c.get(Calendar.MONTH) + 1;
int day = c.get(Calendar.DATE);
int hour = c.get(Calendar.HOUR);
int minute = c.get(Calendar.MINUTE);
int second = c.get(Calendar.SECOND);
System.out.println(year + "-" + month + "-" + day + " " + hour + ":" + minute + ":" + second);