1、前言
Python实现的qq连连看辅助, 仅用于学习, 请在练习模式下使用, 请不要拿去伤害玩家们...
很多人学习python,不知道从何学起。
很多人学习python,掌握了基本语法过后,不知道在哪里寻找案例上手。
很多已经做案例的人,却不知道如何去学习更加高深的知识。
那么针对这三类人,我给大家提供一个好的学习平台,免费领取视频教程,电子书籍,以及课程的源代码!
QQ群:1097524789
2、基本环境配置
版本:Python3.6
系统:Windows
3、相关模块:
1import PIL.ImageGrab
2import pyautogui
3import win32api
4import win32gui
5import win32con
6import time
7import random
1import PIL.ImageGrabimport pyautoguiimport win32apiimport win32guiimport win32conimport timeimport random
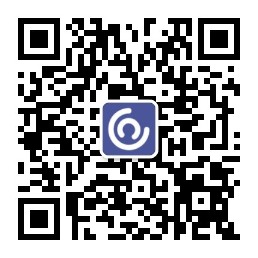
4、使用方法
开始游戏后运行就行了, 再次提示, 请在练习模式中使用, 否则可能会被其他玩家举报。
效果图
5、代码实现
1import PIL.ImageGrab
2import pyautogui
3import win32api
4import win32gui
5import win32con
6import time
7import random
8
9def color_hash(color):
10 value = ""
11 for i in range(5):
12 value += "%d,%d,%d," % (color[0], color[1], color[2])
13 return hash(value)
14
15
16def image_hash(img):
17 value = ""
18 for i in range(5):
19 c = img.getpixel((i * 3, i * 3))
20 value += "%d,%d,%d," % (c[0], c[1], c[2])
21 return hash(value)
22
23
24def game_area_image_to_matrix():
25 pos_to_image = {}
26
27 for row in range(ROW_NUM):
28 pos_to_image[row] = {}
29 for col in range(COL_NUM):
30 grid_left = col * grid_width
31 grid_top = row * grid_height
32 grid_right = grid_left + grid_width
33 grid_bottom = grid_top + grid_height
34
35 grid_image = game_area_image.crop((grid_left, grid_top, grid_right, grid_bottom))
36
37 pos_to_image[row][col] = grid_image
38
39 pos_to_type_id = {}
40 image_map = {}
41
42 empty_hash = color_hash((48, 76, 112))
43
44 for row in range(ROW_NUM):
45 pos_to_type_id[row] = {}
46 for col in range(COL_NUM):
47 this_image = pos_to_image[row][col]
48 this_image_hash = image_hash(this_image)
49 if this_image_hash == empty_hash:
50 pos_to_type_id[row][col] = 0
51 continue
52 image_map.setdefault(this_image_hash, len(image_map) + 1)
53 pos_to_type_id[row][col] = image_map.get(this_image_hash)
54
55 return pos_to_type_id
56
57
58def solve_matrix_one_step():
59 for key in map:
60 arr = map[key]
61 arr_len = len(arr)
62 for index1 in range(arr_len - 1):
63 point1 = arr[index1]
64 x1 = point1[0]
65 y1 = point1[1]
66 for index2 in range(index1 + 1, arr_len):
67 point2 = arr[index2]
68 x2 = point2[0]
69 y2 = point2[1]
70 if verifying_connectivity(x1, y1, x2, y2):
71 arr.remove(point1)
72 arr.remove(point2)
73 matrix[y1][x1] = 0
74 matrix[y2][x2] = 0
75 if arr_len == 2:
76 map.pop(key)
77 return y1, x1, y2, x2
78
79
80def verifying_connectivity(x1, y1, x2, y2):
81 max_y1 = y1
82 while max_y1 + 1 < ROW_NUM and matrix[max_y1 + 1][x1] == 0:
83 max_y1 += 1
84 min_y1 = y1
85 while min_y1 - 1 >= 0 and matrix[min_y1 - 1][x1] == 0:
86 min_y1 -= 1
87
88 max_y2 = y2
89 while max_y2 + 1 < ROW_NUM and matrix[max_y2 + 1][x2] == 0:
90 max_y2 += 1
91 min_y2 = y2
92 while min_y2 - 1 >= 0 and matrix[min_y2 - 1][x2] == 0:
93 min_y2 -= 1
94
95 rg_min_y = max(min_y1, min_y2)
96 rg_max_y = min(max_y1, max_y2)
97 if rg_max_y >= rg_min_y:
98 for index_y in range(rg_min_y, rg_max_y + 1):
99 min_x = min(x1, x2)
100 max_x = max(x1, x2)
101 flag = True
102 for index_x in range(min_x + 1, max_x):
103 if matrix[index_y][index_x] != 0:
104 flag = False
105 break
106 if flag:
107 return True
108
109 max_x1 = x1
110 while max_x1 + 1 < COL_NUM and matrix[y1][max_x1 + 1] == 0:
111 max_x1 += 1
112 min_x1 = x1
113 while min_x1 - 1 >= 0 and matrix[y1][min_x1 - 1] == 0:
114 min_x1 -= 1
115
116 max_x2 = x2
117 while max_x2 + 1 < COL_NUM and matrix[y2][max_x2 + 1] == 0:
118 max_x2 += 1
119 min_x2 = x2
120 while min_x2 - 1 >= 0 and matrix[y2][min_x2 - 1] == 0:
121 min_x2 -= 1
122
123 rg_min_x = max(min_x1, min_x2)
124 rg_max_x = min(max_x1, max_x2)
125 if rg_max_x >= rg_min_x:
126 for index_x in range(rg_min_x, rg_max_x + 1):
127 min_y = min(y1, y2)
128 max_y = max(y1, y2)
129 flag = True
130 for index_y in range(min_y + 1, max_y):
131 if matrix[index_y][index_x] != 0:
132 flag = False
133 break
134 if flag:
135 return True
136
137 return False
138
139
140def execute_one_step(one_step):
141 from_row, from_col, to_row, to_col = one_step
142
143 from_x = game_area_left + (from_col + 0.5) * grid_width
144 from_y = game_area_top + (from_row + 0.5) * grid_height
145
146 to_x = game_area_left + (to_col + 0.5) * grid_width
147 to_y = game_area_top + (to_row + 0.5) * grid_height
148
149 pyautogui.moveTo(from_x, from_y)
150 pyautogui.click()
151
152 pyautogui.moveTo(to_x, to_y)
153 pyautogui.click()
154
155
156if __name__ == '__main__':
157
158 COL_NUM = 19
159 ROW_NUM = 11
160
161 screen_width = win32api.GetSystemMetrics(0)
162 screen_height = win32api.GetSystemMetrics(1)
163
164 hwnd = win32gui.FindWindow(win32con.NULL, 'QQ游戏 - 连连看角色版')
165 if hwnd == 0:
166 exit(-1)
167
168 win32gui.ShowWindow(hwnd, win32con.SW_RESTORE)
169 win32gui.SetForegroundWindow(hwnd)
170 window_left, window_top, window_right, window_bottom = win32gui.GetWindowRect(hwnd)
171 if min(window_left, window_top) < 0 or window_right > screen_width or window_bottom > screen_height:
172 exit(-1)
173 window_width = window_right - window_left
174 window_height = window_bottom - window_top
175
176 game_area_left = window_left + 14.0 / 800.0 * window_width
177 game_area_top = window_top + 181.0 / 600.0 * window_height
178 game_area_right = window_left + 603 / 800.0 * window_width
179 game_area_bottom = window_top + 566 / 600.0 * window_height
180
181 game_area_width = game_area_right - game_area_left
182 game_area_height = game_area_bottom - game_area_top
183 grid_width = game_area_width / COL_NUM
184 grid_height = game_area_height / ROW_NUM
185
186 game_area_image = PIL.ImageGrab.grab((game_area_left, game_area_top, game_area_right, game_area_bottom))
187
188 matrix = game_area_image_to_matrix()
189
190 map = {}
191
192 for y in range(ROW_NUM):
193 for x in range(COL_NUM):
194 grid_id = matrix[y][x]
195 if grid_id == 0:
196 continue
197 map.setdefault(grid_id, [])
198 arr = map[grid_id]
199 arr.append([x, y])
200
201 pyautogui.PAUSE = 0
202
203 while True:
204 one_step = solve_matrix_one_step()
205 if not one_step:
206 exit(0)
207 execute_one_step(one_step)
208 time.sleep(random.randint(0,0)/1000)
主要思路就是利用pywin32获取连连看游戏句柄, 获取游戏界面的图片, 对方块进行切割, 对每个方块取几个点的颜色进行比对, 均相同则认为是同一个方块,
然后模拟鼠标取消就行了, 代码的最后一行是每次点击的间隔。