试题编号: |
201812-2 |
试题名称: |
小明放学 |
时间限制: |
1.0s |
内存限制: |
512.0MB |
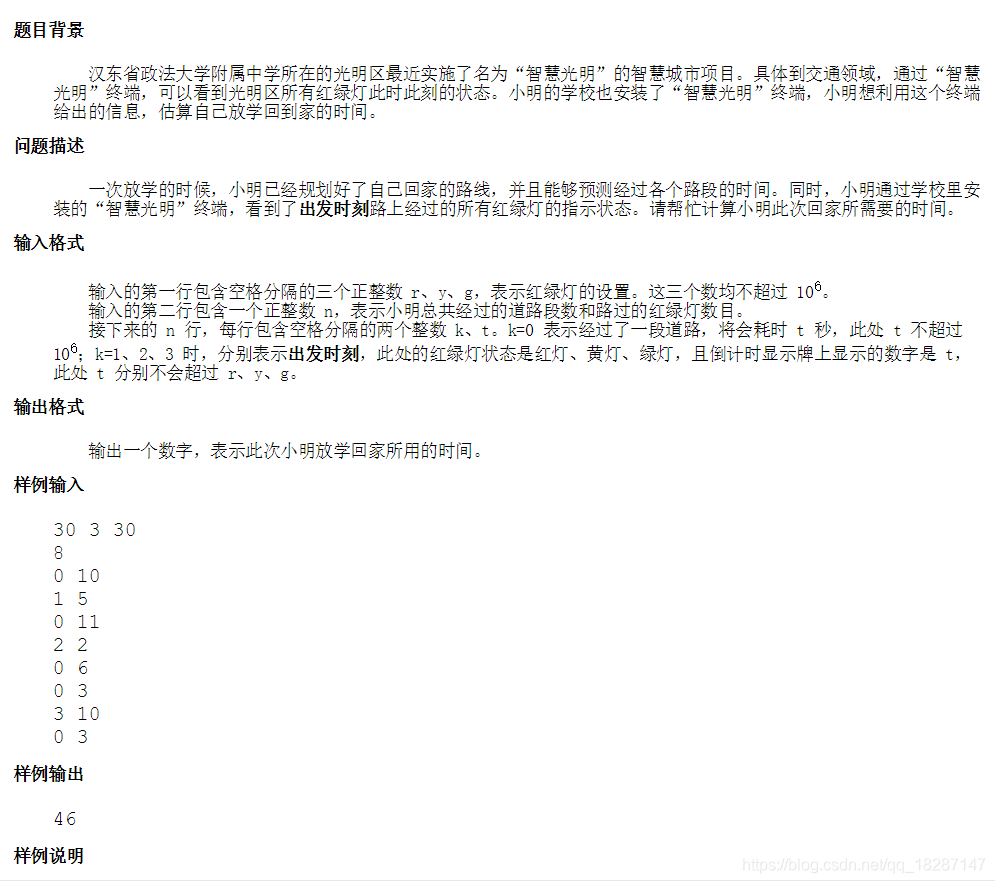
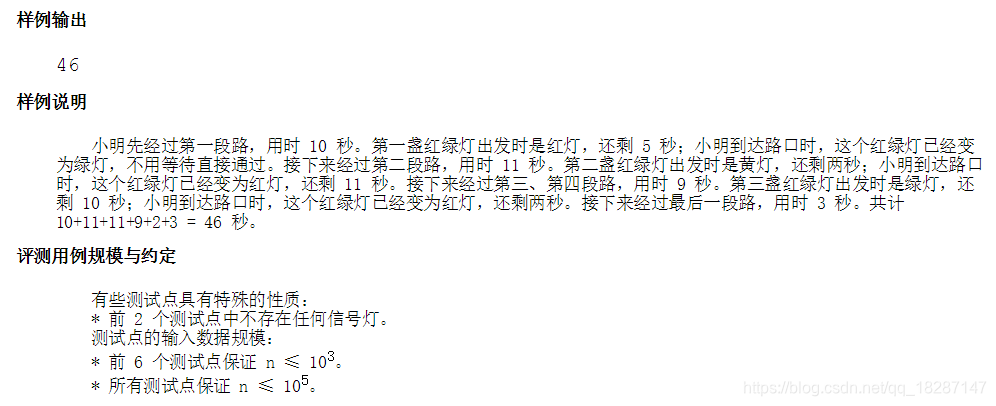
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
class Main{
//将红绿灯时间设为静态变量,便于静态方法调用
public static long red;
public static long yellow;
public static long green;
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
//输入红黄绿灯时长
red = in.nextInt();
yellow = in.nextInt();
green = in.nextInt();
//输入路段
long n = in.nextInt();
List<Light> list = new ArrayList<Light>();
//初始化路段红绿灯
for(long i = 0;i < n;i++){
list.add(new Light(in.nextInt(),in.nextInt()));
}
in.close();
//经历的时间总和
long sum = 0;
//遍历存储路段红绿灯状况的list
for(int i=0;i<n;i++){
//如果状态0 直接通过该路段
if(list.get(i).state == 0){
sum += list.get(i).remain_time;
}else if(list.get(i).state == 1){
//如果是红灯
//把时间重置成时间轴上的数字
//比如题中数字可以想象成一个时间轴
long time = red - list.get(i).remain_time;
//经过当前sum时间,还需等待多长时间
//就调用howLongToStay(long sum,long time)
long howLongToStay = howLongToStay(sum,time);
//最后sum把需要等待的时间累加上
sum += howLongToStay;
}else if(list.get(i).state == 2){ //黄灯
//转换成时间轴上的时间
long time = red + green + yellow - list.get(i).remain_time;
//需要等待的时长
long howLongToStay = howLongToStay(sum,time);
//时间累加
sum+=howLongToStay;
}else{ //绿灯
//转换成时间轴上的时间
long time = red + green - list.get(i).remain_time;
//需要等待的时长
long howLongToStay = howLongToStay(sum,time);
//时间累加
sum += howLongToStay;
}
}
//输出
System.out.println(sum);
}
private static long howLongToStay(long sum, long time){
//(sum+time)对(red+yellow+green)取模,表示当前total时刻落在时间轴上的哪个位置
long total = (sum + time) % (red + yellow + green);
//判断该时刻需要等待多长时间
if(total >=0 && total < red){ //红灯区间
return red - total;
}else if(total >= red && total < red + green){ //绿灯区间
return 0;
}else{
return (red + yellow + green) - total + red; //黄灯区间
}
}
}
class Light{
long state; //此时的状态0 1 2 3
long remain_time;
public Light(long state,long remain_time){
this.state = state;
this.remain_time = remain_time;
}
}