1. 什么是IOU?
IoU 的全称为交并比(Intersection over Union),通过这个名称我们大概可以猜到 IoU 的计算方法。IoU 计算的是 “预测的边框” 和 “真实的边框” 的交集和并集的比值。该值越大,说明”预测框“越接近”真实框“。
iou定义如下:
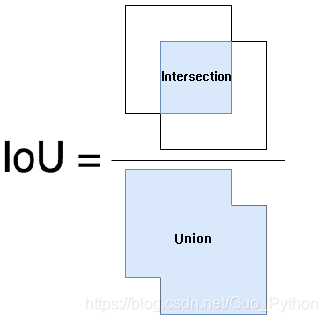
2. IOU计算
直接上代码:
# --*-- coding:utf-8 -*-
import cv2
def draw_box(img, box):
x,y,x1,y1 = box
cv2.rectangle(img, (x,y), (x1, y1), (0,0,255), 2)
return img
def iou(bbox1, bbox2):
"""
Calculates the intersection-over-union of two bounding boxes.
"""
bbox1 = [float(x) for x in bbox1]
bbox2 = [float(x) for x in bbox2]
(x0_1, y0_1, x1_1, y1_1) = bbox1
(x0_2, y0_2, x1_2, y1_2) = bbox2
# get the overlap rectangle
overlap_x0 = max(x0_1, x0_2)
overlap_y0 = max(y0_1, y0_2)
overlap_x1 = min(x1_1, x1_2)
overlap_y1 = min(y1_1, y1_2)
# check if there is an overlap
if overlap_x1 - overlap_x0 <= 0 or overlap_y1 - overlap_y0 <= 0:
return 0
# if yes, calculate the ratio of the overlap to each ROI size and the unified size
size_1 = (x1_1 - x0_1) * (y1_1 - y0_1)
size_2 = (x1_2 - x0_2) * (y1_2 - y0_2)
size_intersection = (overlap_x1 - overlap_x0) * (overlap_y1 - overlap_y0)
size_union = size_1 + size_2 - size_intersection
return size_intersection / size_union
if __name__ == "__main__":
img_path = "test.jpg"
img = cv2.imread(img_path)
person_box = [210, 33, 328, 191]
horse_box = [65, 73, 403, 310]
img = draw_box(img, person_box)
img = draw_box(img, horse_box)
iou_result = iou(person_box, horse_box)
print("The IOU of person and horse is:%s" % iou_result)
cv2.imwrite("result.jpg", img)
python iou.py
输出:The IOU of person and horse is:0.16414778487727819
test.jpg和result.jpg如下图:
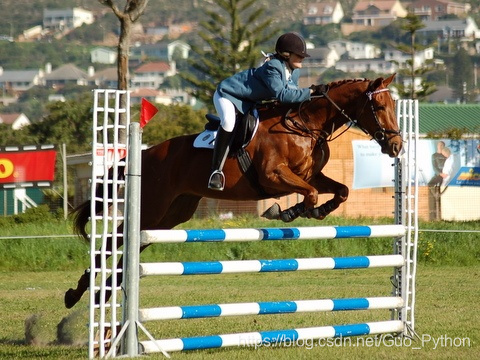
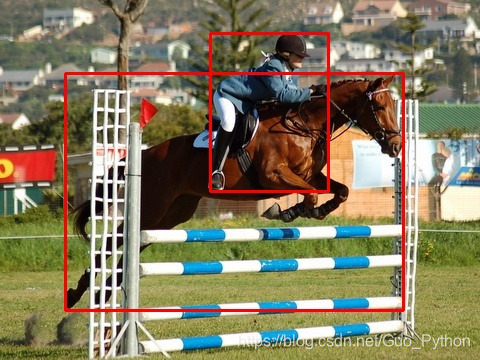
人和马的IOU为:0.164
IOU为目标检测的基础,从这里开始吧!