A.Three Friends
Three friends are going to meet each other. Initially, the first friend stays at the position x=a, the second friend stays at the position x=b and the third friend stays at the position x=c on the coordinate axis Ox.
In one minute each friend independently from other friends can change the position x by 1 to the left or by 1 to the right (i.e. set x:=x−1 or x:=x+1) or even don’t change it.
Let’s introduce the total pairwise distance — the sum of distances between each pair of friends. Let a′, b′ and c′ be the final positions of the first, the second and the third friend, correspondingly. Then the total pairwise distance is |a′−b′|+|a′−c′|+|b′−c′|, where |x| is the absolute value of x.
Friends are interested in the minimum total pairwise distance they can reach if they will move optimally. Each friend will move no more than once. So, more formally, they want to know the minimum total pairwise distance they can reach after one minute.
You have to answer q independent test cases.
Input
The first line of the input contains one integer q (1≤q≤1000) — the number of test cases.
The next q lines describe test cases. The i-th test case is given as three integers a,b and c (1≤a,b,c≤109) — initial positions of the first, second and third friend correspondingly. The positions of friends can be equal.
Output
For each test case print the answer on it — the minimum total pairwise distance (the minimum sum of distances between each pair of friends) if friends change their positions optimally. Each friend will move no more than once. So, more formally, you have to find the minimum total pairwise distance they can reach after one minute.
Example
Input
8
3 3 4
10 20 30
5 5 5
2 4 3
1 1000000000 1000000000
1 1000000000 999999999
3 2 5
3 2 6
Output
0
36
0
0
1999999994
1999999994
2
4
本题的大致题意是有三个人站在数轴的三个点上,每个人可至多移动一次(向左或者向右),求三者的两两之差的绝对值的最小值。
水题,直接求最大值和最小值之差的两倍即可,上代码
#include<iostream>
#include<cstdio>
#include<algorithm>
#include<cstring>
#include<string>
using namespace std;
typedef long long ll;
const int maxn = 200005;
ll n,a,Max, sum1, sum2, ans, cnt, i, j, k,t,b,c,Mid,Min;
ll max(ll a, ll b, ll c)
{
ll temp;
temp = max(a, b);
temp = max(temp, c);
return temp;
}
ll min(ll a, ll b, ll c)
{
ll temp;
temp = min(a, b);
temp = min(temp, c);
return temp;
}
int main()
{
ios::sync_with_stdio(false);
cin >> t;
while (t--)
{
cin >> a >> b >> c;
Max = max(a, b, c);
Min = min(a, b, c);
if (Max > Min) Min++;
if (Min < Max) Max--;
cout << 2 * (Max - Min) << endl;
}
return 0;
}
B. Snow Walking Robot
Recently you have bought a snow walking robot and brought it home. Suppose your home is a cell on an infinite grid.
You also have the sequence of instructions of this robot. It is written as the string consisting of characters ‘L’, ‘R’, ‘U’ and ‘D’. If the robot is in the cell right now, he can move to one of the adjacent cells (depending on the current instruction).
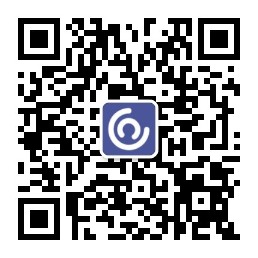
If the current instruction is ‘L’, then the robot can move to the left to ;
if the current instruction is ‘R’, then the robot can move to the right to ;
if the current instruction is ‘U’, then the robot can move to the top to ;
if the current instruction is ‘D’, then the robot can move to the bottom to .
You’ve noticed the warning on the last page of the manual: if the robot visits some cell (except ) twice then it breaks.
So the sequence of instructions is valid if the robot starts in the cell , performs the given instructions, visits no cell other than two or more times and ends the path in the cell . Also cell should be visited at most two times: at the beginning and at the end (if the path is empty then it is visited only once). For example, the following sequences of instructions are considered valid: “UD”, “RL”, “UUURULLDDDDLDDRRUU”, and the following are considered invalid: “U” (the endpoint is not ) and “UUDD” (the cell is visited twice).
The initial sequence of instructions, however, might be not valid. You don’t want your robot to break so you decided to reprogram it in the following way: you will remove some (possibly, all or none) instructions from the initial sequence of instructions, then rearrange the remaining instructions as you wish and turn on your robot to move.
Your task is to remove as few instructions from the initial sequence as possible and rearrange the remaining ones so that the sequence is valid. Report the valid sequence of the maximum length you can obtain.
Note that you can choose any order of remaining instructions (you don’t need to minimize the number of swaps or any other similar metric).
You have to answer independent test cases.
Input
The first line of the input contains one integer () — the number of test cases.
The next lines contain test cases. The -th test case is given as the string consisting of at least and no more than characters ‘L’, ‘R’, ‘U’ and ‘D’ — the initial sequence of instructions.
It is guaranteed that the sum of (where is the length of ) does not exceed over all test cases ().
Output
For each test case print the answer on it. In the first line print the maximum number of remaining instructions. In the second line print the valid sequence of remaining instructions the robot has to perform. The moves are performed from left to right in the order of the printed sequence. If there are several answers, you can print any. If the answer is , you are allowed to print an empty line (but you can don’t print it).
Example
inputCopy
6
LRU
DURLDRUDRULRDURDDL
LRUDDLRUDRUL
LLLLRRRR
URDUR
LLL
outputCopy
2
LR
14
RUURDDDDLLLUUR
12
ULDDDRRRUULL
2
LR
2
UD
0
Note
There are only two possible answers in the first test case: “LR” and “RL”.
The picture corresponding to the second test case:
Note that the direction of traverse does not matter
Another correct answer to the third test case: “URDDLLLUURDR”.
水题,大致题意就是除原点外不能走重复的点,在给定的字符串中尽量删减较少的字符,然后重新排序使机器人按此顺序走时最终能回到原点且满足题意。
思路较简单,上代码
#include<iostream>
#include<algorithm>
#include<cstdio>
#include<iomanip>
#include<cmath>
#include<cstring>
#include<string>
using namespace std;
typedef long long ll;
ll t,n,r,i,j,k,sum,ans,cnt,a,b,m,c,d;
const int maxn=100005;
char str[maxn],str1[maxn];
int main()
{
ios::sync_with_stdio(false);
cin>>t;
while(t--)
{
cin>>str;
int len=strlen(str);
a=0;
b=0;
c=0;
d=0;
for(int i=0;i<len;i++)
{
if(str[i]=='L')
a++;
if(str[i]=='R')
b++;
if(str[i]=='U')
c++;
if(str[i]=='D')
d++;
}
ans=min(a,b);
cnt=min(c,d);
sum=2*ans+2*cnt;
if(ans&&cnt)
{
cout<<sum<<endl;
for(i=1;i<=ans;i++) cout<<"L";
for(i=1;i<=cnt;i++) cout<<"U";
for(i=1;i<=ans;i++) cout<<"R";
for(i=1;i<=cnt;i++) cout<<"D";
cout<<endl;
}
else
{
if(ans) cout<<"2"<<endl<<"LR"<<endl;
else if(cnt) cout<<"2"<<endl<<"UD"<<endl;
else cout<<0<<endl;
}
}
}
C.Yet Another Broken Keyboard
Recently, Norge found a string s=s1s2…sn consisting of n lowercase Latin letters. As an exercise to improve his typing speed, he decided to type all substrings of the string s. Yes, all n(n+1)2 of them!
A substring of s is a non-empty string x=s[a…b]=sasa+1…sb (1≤a≤b≤n). For example, “auto” and “ton” are substrings of “automaton”.
Shortly after the start of the exercise, Norge realized that his keyboard was broken, namely, he could use only k Latin letters c1,c2,…,ck out of 26.
After that, Norge became interested in how many substrings of the string s he could still type using his broken keyboard. Help him to find this number.
Input
The first line contains two space-separated integers n and k (1≤n≤2⋅105, 1≤k≤26) — the length of the string s and the number of Latin letters still available on the keyboard.
The second line contains the string s consisting of exactly n lowercase Latin letters.
The third line contains k space-separated distinct lowercase Latin letters c1,c2,…,ck — the letters still available on the keyboard.
Output
Print a single number — the number of substrings of s that can be typed using only available letters c1,c2,…,ck.
Examples
Input
7 2
abacaba
a b
Output
12
Input
10 3
sadfaasdda
f a d
Output
21
Input
7 1
aaaaaaa
b
Output
0
这个题题意就是给你一串字符串,但是主人公的键盘只有k个键好用,问你仅用这k个键,可以打出多少子字符串。
水题,我直接遍历,没想到一遍过。
#include<iostream>
#include<cstdio>
#include<algorithm>
#include<cstring>
#include<string>
using namespace std;
typedef long long ll;
const int maxn = 200005;
ll n,a,Max, sum, ans, cnt, i, j, k,t,b,c,Mid,Min;
char ch[30],str[maxn];
int main()
{
ios::sync_with_stdio(false);
cin >> n >> k;
cin >> str;
for (i = 1;i <= k;i++)
cin >> ch[i];
for (i = 0;i < n;i++)
{
for (j = 1;j <= k;j++)
if (str[i] == ch[j]) break;
if (j == k + 1)
{
sum += (ans * (ans + 1)) / 2;
ans = 0;
}
else
ans++;
}
sum += (ans * (ans + 1)) / 2;
cout << sum << endl;
return 0;
}
D. Remove One Element
You are given an array a consisting of n integers.
You can remove at most one element from this array. Thus, the final length of the array is n−1 or n.
Your task is to calculate the maximum possible length of the strictly increasing contiguous subarray of the remaining array.
Recall that the contiguous subarray a with indices from l to r is a[l…r]=al,al+1,…,ar. The subarray a[l…r] is called strictly increasing if al<al+1<⋯<ar.
Input
The first line of the input contains one integer n (2≤n≤2⋅105) — the number of elements in a.
The second line of the input contains n integers a1,a2,…,an (1≤ai≤109), where ai is the i-th element of a.
Output
Print one integer — the maximum possible length of the strictly increasing contiguous subarray of the array a after removing at most one element.
Examples
Input
5
1 2 5 3 4
Output
4
Input
2
1 2
Output
2
Input
7
6 5 4 3 2 4 3
Output
2
Note
In the first example, you can delete a3=5. Then the resulting array will be equal to [1,2,3,4] and the length of its largest increasing subarray will be equal to 4.
这个题大意就是给出一串数列,可以从这列数中去除至多一个数,寻找改变后的最长上升连续序列。
这个题我想了足足一个小时,尝试了好几种办法,刚开始是想计算从此位置开始到往后数第二个突出点之前的长度,我觉得思路应该没问题但是太难实现,后来进入了一个误区,只开了一个一维dp数组导致无法计算去除后的长度,一直样例过不去。最后才尝试了二维dp数组,从前往后和从后往前分别遍历,找到a[i + 1] > a[i - 1]时再计算二者对应的长度和,这样就能ac了
#include<iostream>
#include<cstdio>
#include<algorithm>
#include<cstring>
#include<string>
using namespace std;
typedef long long ll;
const int maxn = 200005;
ll n,a[maxn],Max, sum1, sum2, ans, cnt, i, j, k,dp[maxn][3];
int main()
{
ios::sync_with_stdio(false);
cin >> n>>a[1];
dp[1][1] = 1;
for (i = 2;i <= n;i++)
{
cin >> a[i];
if (a[i] > a[i - 1])
dp[i][1] = dp[i - 1][1] + 1;
else
dp[i][1] = 1;
}
dp[n][2] = 1;
for (i = n - 1;i >= 1;i--)
{
if (a[i] < a[i + 1])
dp[i][2] = dp[i + 1][2] + 1;
else
dp[i][2] = 1;
}
Max = 0;
for (i = 1;i <= n;i++)
Max = max(Max, dp[i][1]);
for(i=2;i<=n-1;i++)
{
if (a[i + 1] > a[i - 1])
Max = max(Max, dp[i - 1][1] + dp[i + 1][2]);
}
cout << Max << endl;
}
E. Nearest Opposite Parity
You are given an array a consisting of n integers. In one move, you can jump from the position i to the position i−ai (if 1≤i−ai) or to the position i+ai (if i+ai≤n).
For each position i from 1 to n you want to know the minimum the number of moves required to reach any position j such that aj has the opposite parity from ai (i.e. if ai is odd then aj has to be even and vice versa).
Input
The first line of the input contains one integer n (1≤n≤2⋅105) — the number of elements in a.
The second line of the input contains n integers a1,a2,…,an (1≤ai≤n), where ai is the i-th element of a.
Output
Print n integers d1,d2,…,dn, where di is the minimum the number of moves required to reach any position j such that aj has the opposite parity from ai (i.e. if ai is odd then aj has to be even and vice versa) or -1 if it is impossible to reach such a position.
Example
input
10
4 5 7 6 7 5 4 4 6 4
output
1 1 1 2 -1 1 1 3 1 1
题意:
给出一个数组,对于数组中每个元素,都可以跳向i+a[i]或i-a[i]处,求每个位置跳到与他奇偶性相反的位置所最少需要跳几步。
这个题看了大佬代码,用到了bfs,每次遍历队首的那个结点可达到的(注意已经反向存储)点,查看这些点是否需要更新信息,如果需要更新,更新完之后需要重新将该点加入队列。
#include<bits/stdc++.h>
using namespace std;
const int maxn=2e5+100;
const int inf=0x3f3f3f3f;
int arr[maxn];
int ans[maxn];
vector<int>edge[maxn];
int n;
void solve(int flag)
{
int temp[maxn];
bool vis[maxn];
memset(temp,0,sizeof temp);
memset(vis,false,sizeof vis);
queue<int>q;
for(int i=1;i<=n;++i)
{
if((arr[i]&1)==flag)
{
q.push(i);
//cout<<"??"<<i<<endl;
vis[i]=true;
}
}
while(!q.empty())
{
int f=q.front();
q.pop();
//cout<<"pls"<<f<<endl;
for(int i=0;i<edge[f].size();++i)
{
if(!vis[edge[f][i]])
{
q.push(edge[f][i]);
vis[edge[f][i]]=true;
temp[edge[f][i]]=temp[f]+1;
}
}
}
for(int i=1;i<=n;++i)
{
if(vis[i]&&!((arr[i]&1)==flag))
ans[i]=temp[i];
}
}
int main()
{
cin>>n;
for(int i=1; i<=n; ++i)
cin>>arr[i];
for(int i=1;i<=n;++i)
{
if(i-arr[i]>=1)
{
edge[i-arr[i]].push_back(i);
}
if(i+arr[i]<=n)
{
edge[i+arr[i]].push_back(i);
}
}
solve(1);
solve(0);
for(int i=1;i<=n;++i)
{
if(ans[i])
cout<<ans[i]<<" ";
else
cout<<-1<<" ";
}
return 0;
}
F. Two Bracket Sequences
You are given two bracket sequences (not necessarily regular) s and t consisting only of characters ‘(’ and ‘)’. You want to construct the shortest regular bracket sequence that contains both given bracket sequences as subsequences (not necessarily contiguous).
Recall what is the regular bracket sequence:
() is the regular bracket sequence;
if S is the regular bracket sequence, then (S) is a regular bracket sequence;
if S and T regular bracket sequences, then ST (concatenation of S and T) is a regular bracket sequence.
Recall that the subsequence of the string s is such string t that can be obtained from s by removing some (possibly, zero) amount of characters. For example, “coder”, “force”, “cf” and “cores” are subsequences of “codeforces”, but “fed” and “z” are not.
Input
The first line of the input contains one bracket sequence s consisting of no more than 200 characters ‘(’ and ‘)’.
The second line of the input contains one bracket sequence t consisting of no more than 200 characters ‘(’ and ‘)’.
Output
Print one line — the shortest regular bracket sequence that contains both given bracket sequences as subsequences (not necessarily contiguous). If there are several answers, you can print any.
Examples
Input
(())(()
()))()
Output
(())()()
Input
)
((
Output
(())
Input
)
)))
Output
((()))
Input
())
(()(()(()(
Output
(()()()(()()))
大致题意:
给你两个串,构造出一个字符串使得给出的两个字符串是所构造的字符串的子串,争取使这个构造的字符串最短,输出构造的这个串。
这个题看上去感觉思路不难,但是尝试过dp发现自己实现不了。最后看了大佬们的代码觉得仿佛豁然开朗。这个题不仅用到了dp,还有树的应用。但是我关于树方面的知识一直掌握的不是很好,也许没做出来就是因为基础不牢。
下面贴一下大佬的代码
#include <iostream>
#include <queue>
#include <cstring>
using namespace std;
const int maxn=210;
const int inf=0x3f3f3f3f;
int dp[maxn][maxn][maxn];
struct node{int x,y,z;char c;}st[maxn][maxn][maxn];
string s,t;
int sz,tz;
int nx,ny,nz;
inline void bfs(){
sz=s.size(),tz=t.size();
memset(dp,0x3f,sizeof dp);
dp[0][0][0]=0;
queue<node> q;q.push(node{0,0,0});
while(!q.empty()){
node tp=q.front();q.pop();
//'('
nx=tp.x+(tp.x<sz&&s[tp.x]=='(');
ny=tp.y+(tp.y<tz&&t[tp.y]=='(');
nz=tp.z+1;
if(nz<=200&&dp[nx][ny][nz]==inf){
dp[nx][ny][nz]=dp[tp.x][tp.y][tp.z]+1;
q.push(node{nx,ny,nz});
st[nx][ny][nz]=node{tp.x,tp.y,tp.z,'('};
}
//)
nx=tp.x+(tp.x<sz&&s[tp.x]==')');
ny=tp.y+(tp.y<tz&&t[tp.y]==')');
nz=tp.z-1;
if(nz>=0&&dp[nx][ny][nz]==inf){
dp[nx][ny][nz]=dp[tp.x][tp.y][tp.z]+1;
q.push(node{nx,ny,nz});
st[nx][ny][nz]=node{tp.x,tp.y,tp.z,')'};
}
}
}
int main(){
cin>>s>>t;
bfs();
string res="";
int x=sz,y=tz,z=0;
int px,py,pz;
while(x||y||z){
res+=st[x][y][z].c;
px=st[x][y][z].x;
py=st[x][y][z].y;
pz=st[x][y][z].z;
x=px,y=py,z=pz;
}
sz=res.size();
for(int i=sz-1;i>=0;--i)cout<<res[i];
cout<<endl;
return 0;
}
大佬用了枚举,dp[i][j][k]表示匹配到s的第i个字符,匹配到t的第j个字符,并且此时(的个数比)多k个的时候的最小合法序列长度,虽然看起来长,但是不复杂,还是比较容易懂的。
今天比赛中出了三个题,赛后自己补出来一个题,看大佬代码学了两个题,总结来看我这次没AK首先是水平问题,一个dp题就耗费了我一个多小时的时间,做完之后已经精疲力尽,脑袋已经转不过弯了,只想挑两个简单的题继续充充数,其次的原因是我积极性有点差,做完dp题就没有想继续拼的劲儿了。补题之后我发现我的思路还是太局限,很多明明学过的内容却用不起来,也有很简单的题让我想复杂了的(就比如那个dp题,尝试了很久才用的二维数组dp),明天继续努力吧,争取一个假期能提升到出四个题(梦还是要有的,万一实现了呢)。嘻嘻嘻,加油吧。