29:统计字符数
总时间限制:1000ms 内存限制:65536kB
描述
给定一个由a-z这26个字符组成的字符串,统计其中哪个字符出现的次数最多。
输入
输入包含一行,一个字符串,长度不超过1000。
输出
输出一行,包括出现次数最多的字符和该字符出现的次数,中间以一个空格分开。如果有多个字符出现的次数相同且最多,那么输出ascii码最小的那一个字符。
样例输入
abbccc
样例输出
c 3
扫描二维码关注公众号,回复:
11392385 查看本文章
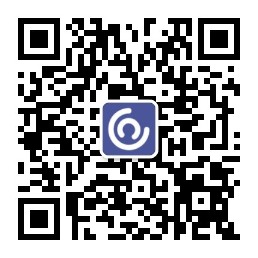
这道题目和我写的上一篇博客采用的思想基本一致,链接如下:
众数(哈尔滨工业大学)
方法一:双数组法
代码如下:
#include<iostream>
#include<cstring>
using namespace std;
#define num 1001
char s[num];
int a[150];
int main()
{
int max=0,max_n=0;
cin >> s;
int len=strlen(s);
for(int i=0; i<len; ++i)
{
a[s[i]]++;
}
for(int i=97; i<123; ++i)
{
if(a[i]>max)
{
max=a[i];
max_n=i;
}
}
cout << (char)max_n << " " << max;
return 0;
}
//29:统计字符数
//a-z的ASCII码:97-122
方法二:map大法
代码如下:
#include<iostream>
#include<map>
#include<cstring>
using namespace std;
#define num 1001
char s[num];
map <char,int> ace;
int main()
{
cin >> s;
int len=strlen(s);
for(int i=0; i<len; ++i)
++ace[s[i]];
int maxn=0;
char a;
for(map <char,int>::iterator i=ace.begin();
i!=ace.end(); ++i)
{
if(i->second>maxn)
{
maxn=i->second;
a=i->first;
}
}
cout << a << " " << maxn;
return 0;
}
//29:统计字符数
//map <key,value> 键值对
//当 map内元素值为 int类型时,默认值为 0
//map的默认排序规则是按照 key从小到大进行排序 即 a.key < b.key 为 true则 a排在 b 前面
方法三:map和set合用
代码如下:
#include<bits/stdc++.h>
using namespace std;
#define num 1001
char s[num];
struct Str
{
int times;
char ch;
};
struct Rule
{
bool operator () ( const Str & s1,const Str & s2) const
{
if(s1.times != s2.times)
return s1.times > s2.times;
else
return s1.ch < s2.ch;
}
};
int main()
{
set<Str,Rule> st;
map<char,int> mp;
cin >> s;
int len = strlen(s);
for(int i=0; i<len; ++i)
++mp[s[i]];
for(map<char,int>::iterator i=mp.begin();
i!=mp.end(); ++i)
{
Str tmp;
tmp.ch=i->first;
tmp.times=i->second;
st.insert(tmp);
}
set<Str,Rule>::iterator i=st.begin();
cout << i->ch << " " << i->times;
return 0;
}
//29:统计字符数
感谢观看!
I’m coming home,Ace!