11.SSM框架集~SpringAOP
本文是上一篇文章的后续,详情点击该链接
SpringAOP的介绍
在前面的文章里,我们学习了SpringIOC之后,我们可以使用IOC的知识将代码中层与层之间的耦合性进行解耦,便于后期维护。但是在实际生产环境中,我们发现随着公司业务的增长,我们会升级某个业务层的业务方法的代码逻辑。升级后的业务方法还需要兼容以前的逻辑处理,也就说再保留原有功能逻辑的基础上,在方法中新增新的逻辑代码。而这个时候,就需要我们去修改当前功能方法的源码,增加新的逻辑代码,然后重新运行项目.这时候出现如下问题:
1.假如我们当前项目已经有其他程序员写好的代码,就需要阅读当前方法的源码,然后再增加自己新的逻辑代码.并且修改好后,需要将新的类文件替换旧的类文件,而阅读代码本身效率极低.
2.假如我们没有源码文件,那么就无法直接修改源码增加新的功能逻辑,怎么办?
解决:
在不修改原有功能逻辑的基础上完成功能扩展.
方案:
SpringAOP
简介:
AOP为Aspect Oriented Programming的缩写,意为:面向切面编程,通过预编译方式和运行期间动态代理实现程序功能的统一维护的一种技术。AOP是OOP的延续,是软件开发中的一个热点,也是Spring框架中的一个重要内容,是函数式编程的一种衍生范型。利用AOP可以对业务逻辑的各个部分进行隔离,从而使得业务逻辑各部分之间的耦合度降低,提高程序的可重用性,同时提高了开发的效率。
AOP, 在纵向执行过程中, 横向的切一刀, 在原有代码逻辑基础上额外补充一些辅助功能. 动态代理的应用过程.
我们将要进行功能扩展相关的材料以及对应的组织规则告诉Spring容器,Spring容器帮我们动态创建一个代理对象。我们直接从Spring容器中获取代理对象完成功能开发。
还是老规矩,先导包....
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.alvin</groupId>
<artifactId>CodeSystem</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjweaver</artifactId>
<version>1.9.4</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-log4j12</artifactId>
<version>1.7.25</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.2.2.RELEASE</version>
</dependency>
</dependencies>
</project>
我们先来回顾一下传统的方法
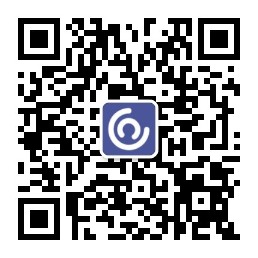
applicationContext.xml
User
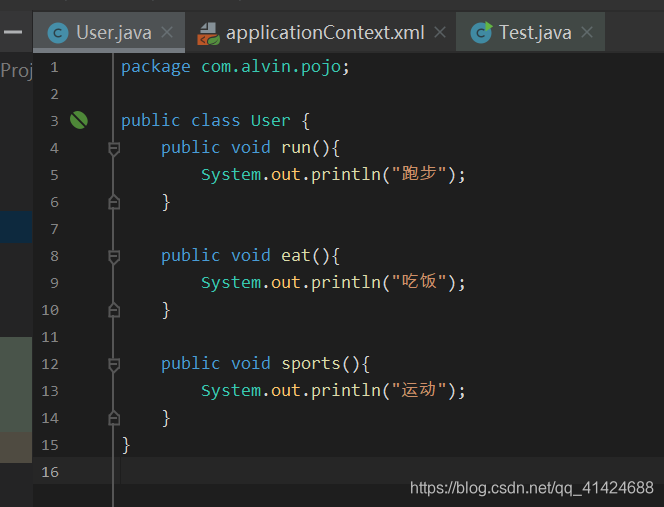
Test
运行
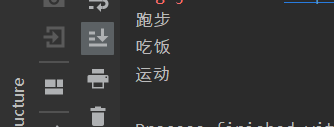
我们看到正常方法在调用执行的时候,都是纵向执行,由上而下。
我们可以把这三个方法想象成是一年前写的代码,然后现在我准备对这个项目进行扩展。而我们AOP则是通过增加切面扩展
SpringAOP的专业概念
连接点:执行的所有方法都可以看成连接点
切点:要进行功能扩展的方法
前置通知:在切点之前执行的方法
后置通知:在切点之后执行的扩展方法
切面:由前置通知+切点+后置通知形成的横向执行的面
织入:由前置通知+切点+后置通知形成切面的过程
AOP前置通知实现
需求:对方法Eat进行代码功能扩展,在方法Eat之前,添加日志记录实现
aop:
切点: 方法Eat
通知: 前置通知
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
https://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
https://www.springframework.org/schema/tx/spring-tx.xsd">
<bean id="user" class="com.alvin.pojo.User"> </bean>
<!-- 创建通知类的对象 -->
<bean id="before" class="com.alvin.advice.BeforAdvice"/>
<!-- 进行切面的织入 -->
<aop:config>
<!-- 配置切点 -->
<aop:pointcut id="pt" expression="execution(* com.alvin.pojo.User.eat())"/>
<!-- 给指定的切点配置通知 -->
<aop:advisor advice-ref="before" pointcut-ref="pt"></aop:advisor>
</aop:config>
</beans>
BeforAdvice
//前置通知
public class BeforAdvice implements MethodBeforeAdvice {
public void before(Method method, Object[] objects, Object o) throws Throwable {
System.out.println("扩展日志操作--前置通知");
}
}
test
public class Test {
public static void main(String[] args) {
ApplicationContext applicationContext = new ClassPathXmlApplicationContext("applicationContext.xml");
User user = applicationContext.getBean("user",User.class);
user.run();
user.eat();
user.sports();
}
}
运行结果
此时我们会发现一个有趣的现象,明明Test类并没有发生改变,可是执行的时候,却多执行了一个方法。这就是我们的前置通知
那么现在我们来添加一个后置通知吧
AOP后置通知实现
新增一个AfterAdvice类
public class AfterAdvice implements AfterReturningAdvice {
public void afterReturning(Object o, Method method, Object[] objects, Object o1) throws Throwable {
System.out.println("方法扩展代码----后置通知");
}
}
applicationContext.xml配置
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
https://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
https://www.springframework.org/schema/tx/spring-tx.xsd">
<bean id="user" class="com.alvin.pojo.User"> </bean>
<!-- 创建通知类的对象 -->
<bean id="before" class="com.alvin.advice.BeforAdvice"/>
<bean id="after" class="com.alvin.advice.AfterAdvice"/>
<!-- 进行切面的织入 -->
<aop:config>
<!-- 配置切点 -->
<aop:pointcut id="pt" expression="execution(* com.alvin.pojo.User.eat())"/>
<!-- 给指定的切点配置通知 -->
<aop:advisor advice-ref="before" pointcut-ref="pt"></aop:advisor>
<!-- 给指定的切点配置后置通知 -->
<aop:advisor advice-ref="after" pointcut-ref="pt"/>
</aop:config>
</beans>
Test保持不变,运行看看
执行的顺序正好如图所示
AOP环绕通知实现
前面我们已经使用前置通知方式和后置通知方式完成了AOP的扩展代码的编写。而我们之前学过过滤器的概念,在过滤器中会先执行一部分代码,执行后如果放行了则继续执行Servlet,Servlet执行后再次回到过滤器中执行。那么,从AOP的角度过滤器就相当于Servlet的扩展对象了。过滤器中的拦截方法,就相当于扩展方法,而我们将扩展代码和调用原有切点方法的代码全部直接声明在一个方法中了,那么能不能采用此种方案来完成我们会自己的AOP扩展呢?
新增一个AroundAdvice类
public class AroundAdvice implements MethodInterceptor {
public Object invoke(MethodInvocation methodInvocation) throws Throwable {
System.out.println("扩展方法前--环绕");
//执行切点中的方法
Object proceed = methodInvocation.proceed();
System.out.println("扩展方法后--环绕");
return proceed;
}
}
配置pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
https://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
https://www.springframework.org/schema/tx/spring-tx.xsd">
<bean id="user" class="com.alvin.pojo.User"> </bean>
<!-- 创建通知类的对象 -->
<bean id="before" class="com.alvin.advice.BeforAdvice"/>
<bean id="after" class="com.alvin.advice.AfterAdvice"/>
<bean id="around" class="com.alvin.advice.AroundAdvice"/>
<!-- 进行切面的织入 -->
<aop:config>
<!-- 配置切点 -->
<aop:pointcut id="pt" expression="execution(* com.alvin.pojo.User.eat())"/>
<!-- 给指定的切点配置通知 -->
<aop:advisor advice-ref="before" pointcut-ref="pt"></aop:advisor>
<!-- 给指定的切点配置后置通知 -->
<aop:advisor advice-ref="after" pointcut-ref="pt"/>
<!-- 给指定的切点配置环绕通知 -->
<aop:advisor advice-ref="around" pointcut-ref="pt"/>
</aop:config>
</beans>
Test保持不变,我们运行看看
AOP异常通知
在我们封装一个功能方法时,一般方法处理数据所造成的异常信息需要抛出,或者 代码编译没有问题,运行期间出现问题,该异常也应该有调用者来处理。那么在Spring AOP中,代理对象是动态生成的,在代理对象中会调用前置通知,后置通知,环绕通 知,切点方法,那么如果这些方法出现异常信息,理论上来说应该在扩展对象中的扩展 方法中完成异常的处理。但是尴尬的是,代理对象是动态生成的,不是由我们创建类然 后根据类文件创建出来的,那么我们就无法直接的声明异常处理代码了,怎么办呢?
在外部声明异常处理的功能方法,让SpringAOP动态生成的代理对象,在生成的catch中调用我们声明的异常处理方法即可。
新增一个ThrowsAdviceImpl类
public class ThrowsAdviceImpl implements ThrowsAdvice {
public void afterThrowing(Exception ex) throws Throwable{
System.out.println("异常通知操作");
}
}
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
https://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
https://www.springframework.org/schema/tx/spring-tx.xsd">
<bean id="user" class="com.alvin.pojo.User"> </bean>
<!-- 创建通知类的对象 -->
<bean id="before" class="com.alvin.advice.BeforAdvice"/>
<bean id="after" class="com.alvin.advice.AfterAdvice"/>
<bean id="around" class="com.alvin.advice.AroundAdvice"/>
<bean id="throws" class="com.alvin.advice.ThrowsAdviceImpl"/>
<!-- 进行切面的织入 -->
<aop:config>
<!-- 配置切点 -->
<aop:pointcut id="pt" expression="execution(* com.alvin.pojo.User.eat())"/>
<!-- 给指定的切点配置通知 -->
<aop:advisor advice-ref="before" pointcut-ref="pt"></aop:advisor>
<!-- 给指定的切点配置后置通知 -->
<aop:advisor advice-ref="after" pointcut-ref="pt"/>
<!-- 给指定的切点配置环绕通知 -->
<aop:advisor advice-ref="around" pointcut-ref="pt"/>
<!-- 给指定的切点配置异常通知 -->
<aop:advisor advice-ref="throws" pointcut-ref="pt"/>
</aop:config>
</beans>
随便报个错看看
public void eat(){
int a = 5 / 0;
System.out.println("吃饭");
}