效果
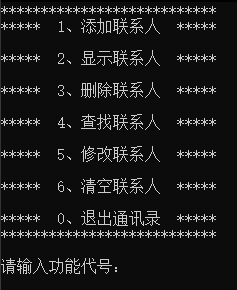
讲义要求:
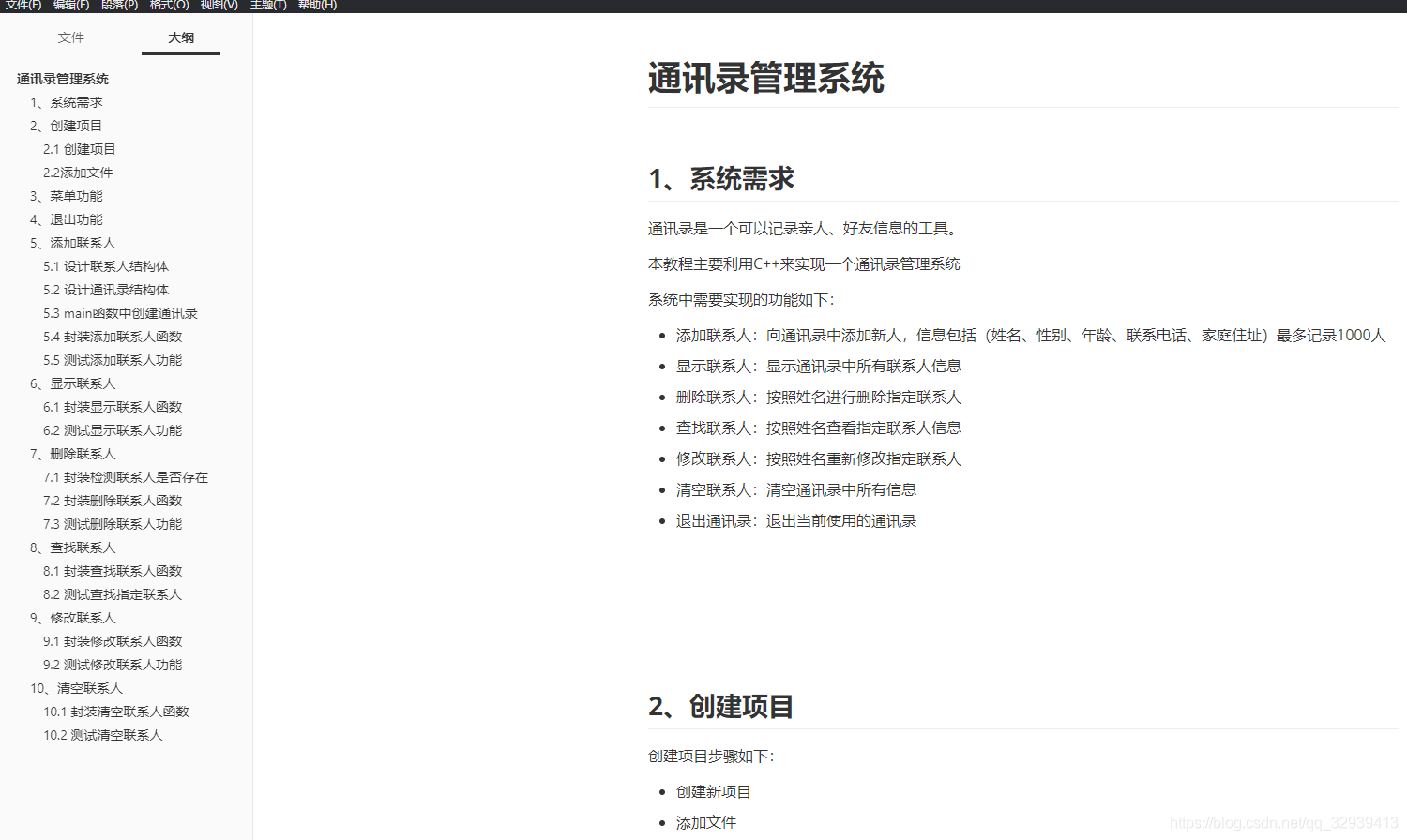
讲义下载链接
代码:
#include <iostream>
#include <string>
using namespace std;
const int MAX_PERSON = 10;
struct Person
{
string name;
string sex;
int age;
string phone;
string address;
};
struct AddressBook
{
Person pArray[MAX_PERSON];
int Adr_Size;
};
void showMenu()
{
cout << "***************************" << endl;
cout << "***** 1、添加联系人 *****\n"
<< endl;
cout << "***** 2、显示联系人 *****\n"
<< endl;
cout << "***** 3、删除联系人 *****\n"
<< endl;
cout << "***** 4、查找联系人 *****\n"
<< endl;
cout << "***** 5、修改联系人 *****\n"
<< endl;
cout << "***** 6、清空联系人 *****\n"
<< endl;
cout << "***** 0、退出通讯录 *****" << endl;
cout << "***************************" << endl;
}
int Add_Person(AddressBook *abs)
{
int i = abs->Adr_Size;
int flag = 0;
int sex_select = 0;
if (abs->Adr_Size - MAX_PERSON >= 0)
{
cout << "通讯录已满,无法添加" << endl;
return 0;
}
else
{
while (true)
{
cout << "通讯录还可添加 " << MAX_PERSON - abs->Adr_Size << " 人!" << endl;
cout << "请输入联系人姓名:" << endl;
cin >> abs->pArray[i].name;
cout << "请选择联系人性别[输入0或1]:" << endl;
cout << "\t0.女\t1.男" << endl;
cin >> sex_select;
sex_choose:
if(sex_select == 0)abs->pArray[i].sex = "女";
else if(sex_select == 1)abs->pArray[i].sex = "男";
else
{
cout << "您的输入有误!,请重新输入"<<endl;
goto sex_choose;
}
cout << "请输入联系人年龄:" << endl;
cin >> abs->pArray[i].age;
cout << "请输入联系人联系电话:" << endl;
cin >> abs->pArray[i].phone;
cout << "请输入联系人家庭住址:" << endl;
cin >> abs->pArray[i].address;
abs->Adr_Size++;
i++;
cout << "添加成功!\n\n是否继续添加?" << endl;
cout << "输入“ 0 ”返回菜单" << endl;
cout << "输入“ 1 ”继续添加" << endl;
cin >> flag;
if (flag == 0)
{
system("cls");
return 0;
}
else
{
system("cls");
}
}
}
return 0;
}
int Show_Persons(AddressBook *abs)
{
int select = 0;
if (abs->Adr_Size > 0)
{
while (true)
{
for (int i = 0; i < abs->Adr_Size; i++)
{
cout << "[ " << i + 1 << " ]" << endl;
cout << "姓名:" << abs->pArray[i].name << "\t";
cout << "联系电话:" << abs->pArray[i].phone << "\t" << endl;
}
cout << "\n【输入“ 0 ”退出】输入通讯录姓名对应数字查看详情!" << endl;
cin >> select;
if (select == 0 || select > abs->Adr_Size)
return 0;
else
{
cout << "[ " << select << " ]" << endl;
cout << "姓名:" << abs->pArray[select - 1].name << "\t";
cout << "性别:" << abs->pArray[select - 1].sex << "\t";
cout << "年龄:" << abs->pArray[select - 1].age << "\t";
cout << "联系电话:" << abs->pArray[select - 1].phone << "\t";
cout << "家庭住址:" << abs->pArray[select - 1].address << "\t\n";
system("pause");
}
}
}
else
{
system("cls");
cout << "通讯录为空!" << endl;
system("pause");
return 0;
}
return 0;
}
int isExist(AddressBook *abs, string name)
{
for (int i = 0; i < abs->Adr_Size; i++)
{
if (abs->pArray[i].name == name)
{
return i + 1;
}
}
return 0;
}
int Delete_Person(AddressBook *abs)
{
string name = "";
int is_exist = 0;
int flag = 0;
while (true)
{
cout << "请输入要删除的联系人的姓名:" << endl;
cin >> name;
is_exist = isExist(abs, name);
if (is_exist == 0)
{
cout << "联系人" << name << " 不存在!" << endl;
system("pause");
}
else
{
for (int i = is_exist - 1; i < abs->Adr_Size; i++)
{
abs->pArray[i] = abs->pArray[i + 1];
}
cout << "联系人" << name << " 成功!\n"
<< endl;
abs->Adr_Size--;
}
cout << "输入“ 0 ”返回菜单" << endl;
cout << "输入“ 1 ”继续删除" << endl;
cin >> flag;
if (flag == 0)
{
system("cls");
return 0;
}
else
{
cout << "-----继续删除-----" << endl;
}
}
}
int Search_Person(AddressBook *abs)
{
string name = "";
int flag = 0;
int is_exist = 0;
while (true)
{
cout << "请输入要查找的联系人的姓名:" << endl;
cin >> name;
is_exist = isExist(abs, name);
if (is_exist == 0)
{
cout << "联系人" << name << " 不存在!" << endl;
system("pause");
}
else
{
cout << "姓名:" << abs->pArray[is_exist - 1].name << "\t";
cout << "性别:" << abs->pArray[is_exist - 1].sex << "\t";
cout << "年龄:" << abs->pArray[is_exist - 1].age << "\t";
cout << "联系电话:" << abs->pArray[is_exist - 1].phone << "\t";
cout << "家庭住址:" << abs->pArray[is_exist - 1].address << "\t\n";
system("pause");
}
cout << "输入“ 0 ”返回菜单" << endl;
cout << "输入“ 1 ”继续查找" << endl;
cin >> flag;
if (flag == 0)
{
system("cls");
return 0;
}
else
{
cout << "-----继续查找-----" << endl;
}
}
}
int Alter_Person(AddressBook *abs)
{
string name = "";
int is_exist = 0;
int flag = 0;
int sex_select = 0;
while (true)
{
cout << "请输入要修改的联系人的姓名:" << endl;
cin >> name;
is_exist = isExist(abs, name);
if (is_exist == 0)
{
cout << "联系人" << name << " 不存在!" << endl;
system("pause");
}
else
{
cout << "-------------------原信息为--------------------:" << endl;
cout << "姓名:" << abs->pArray[is_exist - 1].name << "\t";
cout << "性别:" << abs->pArray[is_exist - 1].sex << "\t";
cout << "年龄:" << abs->pArray[is_exist - 1].age << "\t";
cout << "联系电话:" << abs->pArray[is_exist - 1].phone << "\t";
cout << "家庭住址:" << abs->pArray[is_exist - 1].address << "\t\n";
system("pause");
cout << "-------------------修改信息--------------------:" << endl;
cout << "请输入新的联系人姓名:" << endl;
cin >> abs->pArray[is_exist - 1].name;
cout << "请选择新联系人的性别[输入0或1]:" << endl;
cout << "\t0.女\t1.男" << endl;
cin >> sex_select;
sex_choose:
if(sex_select == 0)abs->pArray[is_exist - 1].sex = "女";
else if(sex_select == 1)abs->pArray[is_exist - 1].sex = "男";
else
{
cout << "您的输入有误!,请重新输入"<<endl;
goto sex_choose;
}
cout << "请输入新的联系人年龄:" << endl;
cin >> abs->pArray[is_exist - 1].age;
cout << "请输入新的联系人联系电话:" << endl;
cin >> abs->pArray[is_exist - 1].phone;
cout << "请输入新的联系人家庭住址:" << endl;
cin >> abs->pArray[is_exist - 1].address;
cout << "-------------------修改结束--------------------:" << endl;
}
cout << "输入“ 0 ”返回菜单" << endl;
cout << "输入“ 1 ”继续修改" << endl;
cin >> flag;
if (flag == 0)
{
system("cls");
return 0;
}
else
{
cout << "-----继续修改-----" << endl;
}
}
}
int Clean_All_Persons(AddressBook *abs)
{
for (int i = 0; i < abs->Adr_Size; i++)
{
abs->pArray[i] = abs->pArray[abs->Adr_Size];
}
abs->Adr_Size = 0;
cout << "联系人已清空!\n" << endl;
system("pause");
}
int main()
{
int function = 0;
AddressBook abs;
abs.Adr_Size = 0;
while (true)
{
system("cls");
showMenu();
cout << endl
<< "请输入功能代号:" << endl;
cin >> function;
switch (function)
{
case 1:
system("cls");
Add_Person(&abs);
break;
case 2:
system("cls");
Show_Persons(&abs);
break;
case 3:
system("cls");
Delete_Person(&abs);
break;
case 4:
system("cls");
Search_Person(&abs);
break;
case 5:
system("cls");
Alter_Person(&abs);
break;
case 6:
system("cls");
Clean_All_Persons(&abs);
break;
case 0:
cout << "\n欢迎再次使用通讯录管理系统,再见!" << endl;
break;
default:
system("cls");
cout << "---------------------------" << endl;
cout << "\n您的输入有误,请重新输入!\n"
<< endl;
cout << "---------------------------" << endl;
break;
}
if (function == 0)
break;
}
system("pause");
}