这章讲了JavaScript的语法、数据类型、流控制语句和函数。理解还是挺好理解的,但有很多和C、C++、Java不同的地方需要记忆。比如,
- JavaScript标识符可以由Unicode字符字符组成。
- JavaScript每条语句后面的分号也不是必需的,语句后面没有分号时,解析器会自己确定语句的结尾。
- “+”,“-”,“*”、“/”、“&&”、“||”、“!”对不同类型操作对象进行计算的规则是不同的。
- switch()括号内不像其他语言必须是字符或数字,而可以放任何类型数据。
- 函数和其他语言也不一样,函数内有个arguments对象用来访问参数数组,函数不介意传递来多少个参数,也不在乎传进来的参数是什么的类型;函数没有重载,如果存在相同的函数名,只会保存最后一个函数。
- 有个label标签语句在以后由break,continue引用
下面是这章做的笔记
typeof用来返回数据类型
var message = "some string"; alert(typeof message); //"string" alert(typeof 95); //"number"
只声明不定义的变量的值为undefined
var message; alert(message == undefined); //true
也可以用undefined显式初始化变量
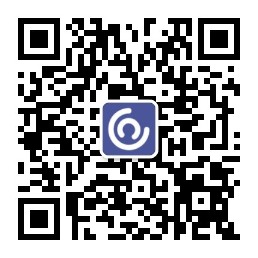
var message = undefined; alert(message == undefined); //true
没声明的变量除了typeof和delete操作,进行其他运算都会报错
var message; //this variable is declared but has a value of undefined //make sure this variable isn't declared //var age alert(message); //"undefined" alert(age); //causes an error
没声明的变量进行typeof操作返回值也是undefined
var message; //this variable is declared but has a value of undefined //make sure this variable isn't declared //var age alert(typeof message); //"undefined" alert(typeof age); //"undefined"
null的typeof操作返回"object"
var car = null; alert(typeof car); //"object"
undefined派生自null,因此他们进行相等测试时,返回true
alert(null == undefined); //true
Boolean()可以将所有类型数据转换成布尔值
var message = "Hello world!"; var messageAsBoolean = Boolean(message); alert(messageAsBoolean); //true
流控制语句会自动将传进来的值转换成Boolean
var message = "Hello world!"; if (message){ alert("Value is true"); }
NaN与任何值都不相等包括他自己
isNaN()先尝试将接收到的值转换成数值,然后判断转换后的值是否是数字,如果是数字则返回false,否则返回true
alert(NaN == NaN); //false alert(isNaN(NaN)); //true alert(isNaN(10)); //false � 10 is a number alert(isNaN("10")); //false � can be converted to number 10 alert(isNaN("blue")); //true � cannot be converted to a number alert(isNaN(true)); //false � can be converted to number 1
Number()将何种数据类型转换成数值
var num1 = Number("Hello world!"); //NaN var num2 = Number(""); //0 var num3 = Number("000011"); //11 var num4 = Number(true); //1 alert(num1); alert(num2); alert(num3); alert(num4);
parseInt()可以识别出整型格式(二、八、十、十六进制)
var num1 = parseInt("1234blue"); //1234 var num2 = parseInt(""); //NaN var num3 = parseInt("0xA"); //10 - hexadecimal var num4 = parseInt(22.5); //22 var num5 = parseInt("70"); //70 - decimal var num6 = parseInt("0xf"); //15 � hexadecimal
paseInt()如果指定了第二个参数,字符串可以不必带0、0x前缀
var num1 = parseInt("10", 2); //2 � parsed as binary var num2 = parseInt("10", 8); //8 � parsed as octal var num3 = parseInt("10", 10); //10 � parsed as decimal var num4 = parseInt("10", 16); //16 � parsed as hexadecimal
toString()返回一个值的字符串表现
var age = 11; var ageAsString = age.toString(); //the string "11" var found = true; var foundAsString = found.toString(); //the string "true" alert(ageAsString); alert(typeof ageAsString); alert(foundAsString); alert(typeof foundAsString);
toString()可以返回数值的二进制、八进制、十六进制、默认情况下返回十进制
var num = 10; alert(num.toString()); //"10" alert(num.toString(2)); //"1010" alert(num.toString(8)); //"12" alert(num.toString(10)); //"10" alert(num.toString(16)); //"a"
toString()不能转换null和undefined的值,而String()可以转换任意类型的值
var value1 = 10; var value2 = true; var value3 = null; var value4; alert(String(value1)); //"10" alert(String(value2)); //"true" alert(String(value3)); //"null" alert(String(value4)); //"undefined"
“++”和“--”会将运算对象先转换成数值再进行递增、递减
var s1 = "2"; var s2 = "z"; var b = false; var f = 1.1; var o = { valueOf: function() { return -1; } }; s1++; //value becomes numeric 3 s2++; //value becomes NaN b++; //value becomes numeric 1 f--; //value becomes 0.10000000000000009 o--; //value becomes numeric �2 alert(s1); alert(s2); alert(b); alert(f); alert(o);
一元加减运算会将值转换成数值
var s1 = "01"; var s2 = "1.1"; var s3 = "z"; var b = false; var f = 1.1; var o = { valueOf: function() { return -1; } }; s1 = +s1; //value becomes numeric 1 s2 = +s2; //value becomes numeric 1.1 s3 = +s3; //value becomes NaN b = +b; //value becomes numeric 0 f = +f; //no change, still 1.1 o = +o; //value becomes numeric �1 alert(s1); alert(s2); alert(s3); alert(b); alert(f); alert(o);
var s1 = "01"; var s2 = "1.1"; var s3 = "z"; var b = false; var f = 1.1; var o = { valueOf: function() { return -1; } }; s1 = -s1; //value becomes numeric -1 s2 = -s2; //value becomes numeric -1.1 s3 = -s3; //value becomes NaN b = -b; //value becomes numeric 0 f = -f; //change to �1.1 o = -o; //value becomes numeric 1 alert(s1); alert(s2); alert(s3); alert(b); alert(f); alert(o);
“<<<”、“>>>”是无符号左移和无符号右移运算符
var oldValue = 64; //equal to binary 1000000 var newValue = oldValue >>> 5; //equal to binary 10 which is decimal 2 alert(newValue); //2
var oldValue = -64; //equal to binary 11111111111111111111111111000000 var newValue = oldValue >>> 5; //equal to decimal 134217726 alert(newValue); //134217726
“!”运算也是先将操作对象转换成布尔值在进行取非
“!!”相当于是Boolean()操作
alert(!false); //true alert(!"blue"); //false alert(!0); //true alert(!NaN); //true alert(!""); //true alert(!12345); //false alert(!!"blue"); //true alert(!!0); //false alert(!!NaN); //false alert(!!""); //false alert(!!12345); //true
逻辑与和逻辑或运算都存在逻辑短路现象
var found = true; var result = (found && someUndeclaredVariable); //error occurs here alert(result); //this line never executes
var found = false; var result = (found && someUndeclaredVariable); //no error alert(result); //works
var found = true; var result = (found || someUndeclaredVariable); //no error alert(result); //works
var found = false; var result = (found || someUndeclaredVariable); //error occurs here alert(result); //this line never executes
加性操作优先转换成字符串,减性操作优先转换成数值
var result1 = 5 + 5; //two numbers alert(result1); //10 var result2 = 5 + "5"; //a number and a string alert(result2); var num1 = 5; var num2 = 10; var message = "The sum of 5 and 10 is " + num1 + num2; alert(message); //"The sum of 5 and 10 is 510"
var num1 = 5; var num2 = 10; var message = "The sum of 5 and 10 is " + (num1 + num2); alert(message); //"The sum of 5 and 10 is 15"
全等“===”只在两个操作数未经转换之前就相等的情况下(数据类型一样)返回true,与之对应的是“!==”
var result1 = ("55" == 55); //true � equal because of conversion var result2 = ("55" === 55); //false � not equal because different data types var result1 = ("55" != 55); //false � equal because of conversion var result2 = ("55" !== 55); //true � not equal because different data types
break与标签配合使用,跳出最外层循环
var num = 0; outermost: for (var i=0; i < 10; i++) { for (var j=0; j < 10; j++) { if (i == 5 && j == 5) { break outermost; } num++; } }
continue与标签配合使用,使跳出最外层之外的循环
var num = 0; outermost: for (var i=0; i < 10; i++) { for (var j=0; j < 10; j++) { if (i == 5 && j == 5) { continue outermost; } num++; } } alert(num); //95 i==5的那一层执行到5时跳出内层循环,继续执行外层循环
switch()括号内可以是任何数据类型
switch ("hello world") { case "hello" + " world": alert("Greeting was found."); break; case "goodbye": alert("Closing was found."); break; default: alert("Unexpected message was found.");
arguments对象可以和命名参数一起使用
function doAdd(num1, num2) { if(arguments.length == 1) { alert(num1 + 10); } else if (arguments.length == 2) { alert(arguments[0] + num2); } } doAdd(10); //20 doAdd(30, 20); //50
JavaScript没有函数重载,后定义的同名函数会覆盖前面的函数
function addSomeNumber(num){ return num + 100; } function addSomeNumber(num) { return num + 200; } var result = addSomeNumber(100); //300 alert(result);
for-in语句
for (var propName in window) { document.write(propName); document.write("<br />"); }
2020-04-23 18:29:22