1161. Maximum Level Sum of a Binary Tree**
https://leetcode.com/problems/maximum-level-sum-of-a-binary-tree/
题目描述
Given the root
of a binary tree, the level of its root
is 1, the level of its children is 2, and so on.
Return the smallest level X
such that the sum of all the values of nodes at level X
is maximal.
Example 1:
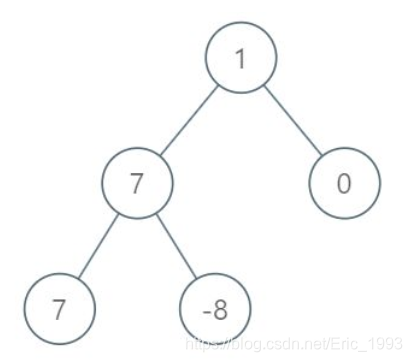
Input: [1,7,0,7,-8,null,null]
Output: 2
Explanation:
Level 1 sum = 1.
Level 2 sum = 7 + 0 = 7.
Level 3 sum = 7 + -8 = -1.
So we return the level with the maximum sum which is level 2.
Note:
- The number of nodes in the given tree is between
1
and10^4
. -10^5 <= node.val <= 10^5
C++ 实现 1
层序遍历, 统计每层的节点之和.
class Solution {
public:
int maxLevelSum(TreeNode* root) {
queue<TreeNode*> q;
q.push(root);
int level = 0, imax = INT32_MIN;
int res = 0;
while (!q.empty()) {
auto size = q.size();
level += 1;
int sum = 0;
while (size --) {
auto r = q.front();
q.pop();
sum += r->val;
if (r->left) q.push(r->left);
if (r->right) q.push(r->right);
}
if (sum > imax) {
res = level;
imax = sum;
}
}
return res;
}
};