我们使用vue-router及vuex时引入它们的方式是直接以<script src =“xxx”>的方式引入且就在一个文件中写。但是实际开发时,我们并不是这样操作的,一般我们会使用VUE-CLI脚手架。但是使用VUE-CLI的话,我们在引入VUE路由器及vuex时写法会稍有不同,这里我来举个通俗易懂的例子(每一步都很详细且代码中比较重要或者难以理解的地方我都注释出来了,希望对大家有所帮助)。
安装VUE-CLI步骤我就不细写了...
全局安装 vue-cli
npm install --global vue-cli
创建一个基于 webpack 模板的新项目
vue init webpack vue-cli
安装依赖,走你
cd vue-cli
npm install
npm run dev
一,在VUE-CLI中使用VUE路由器
最终实现效果:点击链接进入相应的路由(URL)。

实现步骤:
如图1所示,首先确保你安装了VUE路由器
可在VUE-CLI根目录中的的的package.json中的依赖关系看有无VUE路由器;
2,确保VUE-CLI根目录下的SRC中的main.js中引入了路由器;
//main.js
import Vue from 'vue'
import App from './App'
import router from './router' //这里引入了router
Vue.config.productionTip = false
/* eslint-disable no-new */
new Vue({
el: '#app',
router, //这里挂载了router
template: '<App/>',
components: { App }
})
3,由于在main.js中导入路由器了import router from './router'
,因此我们要确保src / router / index.js里的代码完整且正确;
//index.js
import Vue from 'vue'
import Router from 'vue-router'
import Hello from '@/components/Hello'
//import xxx from '@/components/xxx' 中的@表示src
// 因为webpack.base.conf中别名这样写了==> alias: {'@': resolve('src')}
import Hello1 from '@/components/Hello1' //引入Hello1组件
import Hello2 from '@/components/Hello2' //引入Hello2组件
import notFind from '@/components/notFind' //引入notFind组件
Vue.use(Router) //这句别忘记了
export default new Router({
mode:'history', //使用history防止url中出现#
routes: [
{
path: '/',
name: 'Hello',
component: Hello
}
,{
path: '/hello1',
name: 'Hello1',
component: Hello1
}
,{
path: '/hello2',
name: 'Hello2',
component: Hello2
}
,{
path: '*',
name: 'notFind',
component: notFind
}
]
})
如图4所示,由于在index.js中引入了(进口)的src目录下的组件中的Hello.vue,Hello1.vue,Hello2.vue及notFind.vue,因此我需要完善相应代码:
Hello.vue
//Hello.vue
<template>
<div class="hello">
<h1>{{ msg }}</h1>
</div>
</template>
<script>
export default {
name: 'hello',
data () {
return {
msg: '我是src/components里的hello.vue,我通过app.vue里的router-view展示'
}
}
}
</script>
<!-- Add "scoped" attribute to limit CSS to this component only -->
<style scoped>
h1, h2 {
font-weight: normal;
}
ul {
list-style-type: none;
padding: 0;
}
li {
display: inline-block;
margin: 0 10px;
}
a {
color: #42b983;
}
</style>
Hello1.vue
//Hello1.vue
<template>
<div>
<h1>我是Hello1.vue</h1>
</div>
</template>
<script>
export default {
name:'Hello1',
data:function () {
return {}
}
}
</script>
<!-- Add "scoped" attribute to limit CSS to this component only -->
<style scoped>
h1, h2 {
font-weight: normal;
}
ul {
list-style-type: none;
padding: 0;
}
li {
display: inline-block;
margin: 0 10px;
}
a {
color: #42b983;
}
</style>
Hello2.vue
//Hello2.vue
<template>
<div>
<qipa1-component></qipa1-component>
<qipa2-component></qipa2-component>
</div>
</template>
<script>
import qipa1Component from '@/components/qipa1Component'
//import xxx from '@/components/xxx' 中的@表示src
// 因为webpack.base.conf中别名这样写了==> alias: {'@': resolve('src')}
import qipa2Component from '@/components/qipa2Component'
export default {
components:{
qipa1Component,
qipa2Component
}
}
</script>
<!-- Add "scoped" attribute to limit CSS to this component only -->
<style scoped>
h1, h2 {
font-weight: normal;
}
ul {
list-style-type: none;
padding: 0;
}
li {
display: inline-block;
margin: 0 10px;
}
a {
color: #42b983;
}
</style>
由于我们在Hello2.vue使用了自定义两个组件qipa1-component
状语从句:qipa2-component
御姐进口了相应的组件,因此我们在此也要完善这两个组件
qipa1Component.vue
<template>
<h1>我是Hello2组件中的一朵奇葩</h1>
</template>
<!--<script>-->
<!--export default {}-->
<!--</script>-->
<!-- Add "scoped" attribute to limit CSS to this component only -->
<style scoped>
h1, h2 {
font-weight: normal;
}
ul {
list-style-type: none;
padding: 0;
}
li {
display: inline-block;
margin: 0 10px;
}
a {
color: #42b983;
}
</style>
qipa2Component.vue
<template>
<h1>我是Hello2组件中的另一朵奇葩</h1>
</template>
<!--<script>-->
<!--export default {}-->
<!--</script>-->
<!-- Add "scoped" attribute to limit CSS to this component only -->
<style scoped>
h1, h2 {
font-weight: normal;
}
ul {
list-style-type: none;
padding: 0;
}
li {
display: inline-block;
margin: 0 10px;
}
a {
color: #42b983;
}
</style>
接下来我们还为未匹配的路径单独写了个组件应对:
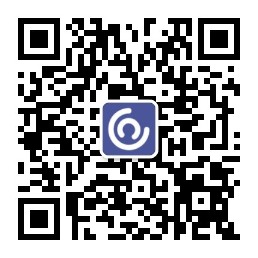
notFind.vue
<template>
<div class="hello">
<h1>{{ msg }}</h1>
</div>
</template>
<script>
export default {
name: 'notFind',
data () {
return {
msg: '对不起,匹配不到对应资源,我是notFind.vue'
}
}
}
</script>
<!-- Add "scoped" attribute to limit CSS to this component only -->
<style scoped>
h1, h2 {
font-weight: normal;
}
ul {
list-style-type: none;
padding: 0;
}
li {
display: inline-block;
margin: 0 10px;
}
a {
color: #42b983;
}
</style>
5,修改App.vue内容
<template>
<div id="app">

<h1>我是src/app.vue的h1</h1>
<router-link to="/">点击进入hello首页</router-link>
<br>
<router-link to="/hello1">点击进入hello1</router-link>
<br>
<router-link to="/hello2">点击进入hello2</router-link>
<br>
<router-link to="/h">点击进入不存在的路径</router-link>
<br>
<router-view></router-view>
</div>
</template>
<style>
#app {
font-family: 'Avenir', Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
通过以上几个步骤,就可以实现了最开始的效果图啦接下来我们来使用vuex。
二,在VUE-CLI中使用vuex
最终效果:通过Hello1.vue中的按钮来改变gettersMsg数据,从而使得只要用到gettersMsg的组件的数据都会相应改变;

1,同样首先要确保安装了vuex
如果未安装,则将目录切换至VUE-CLI后执行 npm install vuex --save
2,创建vuex相关文件夹及文件
在src文件夹下新建store文件夹后,在store文件夹下新建如下文件:
index.js,mutations.js,actions.js,getters.js和rootState.js
3,修改SRC下的入口文件main.js
import Vue from 'vue'
import App from './App'
import router from './router'
import store from './store/index'; //引入store
Vue.config.productionTip = false
/* eslint-disable no-new */
new Vue({
el: '#app',
router,
store, //挂载store
template: '<App/>',
components: { App }
})
4,修改存储/ index.js
由于上面我们引入了存储/ index.js,因此接下来我们需要修改index.js文件:
import Vue from 'vue';
import Vuex from 'vuex';
import * as actions from './actions';
// * 表示将 './actions'模块中的所有接口挂载到actions对象上 as 表示别名的意思
import * as mutations from './mutations';
import * as getters from './getters';
import state from './rootState';
Vue.use(Vuex)
const store = new Vuex.Store({
state,
getters,
actions,
mutations
})
export default store;
5,修改mutations.js,actions.js,getters.js及rootState.js
由于上面引用了这些文件,因此接下来我们来修改这些文件
rootState.js
const state = {
msg: '我是原始数据',
}
export default state;
mutations.js
export const mutationsMsg = (state, payload) => {
state.msg = payload.msg;
}
actions.js
export const changeMsg = ({commit}) => {
commit({
type: 'mutationsMsg', //对应mutation.js中的mutationsMsg方法
msg: '我是修改后的数据~~~'
});
};
getters.js
export const gettersMsg = state => state.msg;
6,修改Hello.vue,Hello1.vue及App.vue
我们想通过Hello1.vue中的按钮来改变数据,因此Hello1.vue修改后的代码如下:
<template>
<div>
<h1>我是Hello1.vue</h1>
<button @click="changeMsg">点我改变数据</button>
<p>gettersMsg数据目前是: {{ gettersMsg }}</p>
</div>
</template>
<script>
import {mapGetters, mapActions} from 'vuex';
export default {
name:'Hello1',
data:function () {
return {}
},
computed: {...mapGetters(['gettersMsg'])},
//对应getters.技术中的gettersMsg
methods: {...mapActions(['changeMsg'])}
//对应 Actions中changeMsg方法|| 映射this.changeMsg() 为 this.$store.dispatch('changeMsg')
}
</script>
<!-- Add "scoped" attribute to limit CSS to this component only -->
<style scoped>
h1, h2 {
font-weight: normal;
}
ul {
list-style-type: none;
padding: 0;
}
li {
display: inline-block;
margin: 0 10px;
}
a {
color: #42b983;
}
</style>
Hello.vue用来展示数据
<template>
<div class="hello">
<h1>{{ gettersMsg }}</h1>
</div>
</template>
<script>
import {mapGetters} from 'vuex';
//引入mapGetters
export default {
name: 'hello',
computed: {...mapGetters(['gettersMsg'])}
//使用mapGetters 辅助函数
}
</script>
<!-- Add "scoped" attribute to limit CSS to this component only -->
<style scoped>
h1, h2 {
font-weight: normal;
}
ul {
list-style-type: none;
padding: 0;
}
li {
display: inline-block;
margin: 0 10px;
}
a {
color: #42b983;
}
</style>
当然,为了方便观察数据,你也可将App.vue修改下
<template>
<div id="app">

<router-link to="/">点击进入hello首页</router-link>
<br>
<router-link to="/hello1">点击进入hello1</router-link>
<br>
<router-view></router-view>
<p> 我是App.vue中的p, gettersMsg 的值是----- {{gettersMsg}}</p>
</div>
</template>
<script>
import {mapGetters} from 'vuex';
export default {
name: 'app',
computed:{...mapGetters(['gettersMsg'])}
}
</script>
<style>
#app {
font-family: 'Avenir', Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
推荐GitHub:(vue的手册的思维导图) https ://github.com/have-not-BUG/XMind
VUE路由器官网路由:https : //router.vuejs.org/zh/guide/
vuex的官网地址:https ://vuex.vuejs.org/zh/getting-started.html
vuex的教程地址:http ://jspang.com/2017/05/03/vuex/
vue-loader官网地址:https ://vue-loader-v14.vuejs.org/zh-cn/