在做分享圈项目后台时,用的是layui提供的后端页面框架,页面挺好看的。
下载layui包:组装我们想要的页面,layui在线示例
github地址:下载示例包
看官方文档api:数据表格 实现数据显示,分页功能。
1.前端页面:
要导入layui的layui.css和layui.js
<link rel="stylesheet" href="<%=request.getContextPath()%>/public/layui/src/css/layui.css">
<script src="<%=request.getContextPath()%>/public/layui/src/layui.js" charset="utf-8"></script>
html:
<table id="test" lay-filter="test"></table>
js操作:
扫描二维码关注公众号,回复:
1099145 查看本文章
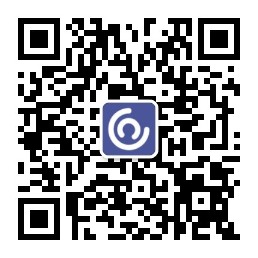
<script> layui.use('table', function(){ var table = layui.table; //渲染 table.render({ elem: '#test' //绑定table表格 ,height: 450 ,url: '<%=request.getContextPath()%>/user/backContent' //后台springmvc接收路径 ,page:true //true表示分页 /* page: { //支持传入 laypage 组件的所有参数(某些参数除外,如:jump/elem) - 详见文档 layout: ['limit', 'count', 'prev', 'page', 'next', 'skip'] //自定义分页布局 //,curr: 5 //设定初始在第 5 页 ,groups: 3 //只显示 1 个连续页码 ,first: true //不显示首页 ,last: true //不显示尾页 }*/ // ,where:{rows:limit} //传参数 ,limit: 10 ,id:'contenttable' ,toolbar: '#toolbarDemo' ,cols: [[ {type: 'checkbox', fixed: 'left'} ,{field:'id', title:'id', width:80, fixed: 'left', unresize: true, sort: true} ,{field:'content', title:'内容', width:120, edit: 'text'} ,{field:'userid', title:'用户id', width:80, edit: 'text', sort: true} ,{field:'nice', title:'点赞数', width:100} ,{field:'createtime', title:'分享时间', width:80, sort: true} ,{field:'pic1', title:'图片1', width:120} ,{field:'pic2', title:'图片2', width:100, sort: true} ,{field:'pic3', title:'图片3', width:120} ]] }); //监听表格行点击 table.on('tr', function(obj){ console.log(obj) }); //监听表格复选框选择 table.on('checkbox(test)', function(obj){ console.log(obj) }); //监听表格单选框选择 table.on('radio(test2)', function(obj){ console.log(obj) }); //监听单元格编辑 table.on('edit(test2)', function(obj){ var value = obj.value //得到修改后的值 ,data = obj.data //得到所在行所有键值 ,field = obj.field; //得到字段 }); //监听工具条 table.on('tool(test)', function(obj){ var data = obj.data; if(obj.event === 'del'){ layer.confirm('真的删除行么', function(index){ obj.del(); layer.close(index); }); } else if(obj.event === 'edit'){ layer.prompt({ formType: 2 ,value: data.username }, function(value, index){ obj.update({ username: value }); layer.close(index); }); } }); var $ = layui.jquery, active = { getCheckData: function(){//获取选中数据 var checkStatus = table.checkStatus('test') ,data = checkStatus.data; layer.alert(JSON.stringify(data)); } ,getCheckLength: function(){//获取选中数目 var checkStatus = table.checkStatus('test') ,data = checkStatus.data; layer.msg('选中了:'+ data.length + ' 个'); } ,isAll: function(){验证是否全选 var checkStatus = table.checkStatus('test'); layer.msg(checkStatus.isAll ? '全选': '未全选') } ,parseTable: function(){ table.init('parse-table-demo', { limit: 3 }); } ,add: function(){ table.addRow('test') } ,delete: function(){ layer.confirm('确认删除吗?', function(index){ table.deleteRow('test') layer.close(index); }); } ,reload:function () { var keyWord=$("#keyWord").val(); var keyType=$("#key_type option:selected").val(); table.reload('contenttable',{ where:{keyWord:keyWord,keyType:keyType} }); } }; $('i').on('click', function(){ var type = $(this).data('type'); active[type] ? active[type].call(this) : ''; }); $('.layui-btn').on('click', function(){ var type = $(this).data('type'); active[type] ? active[type].call(this) : ''; }); $('.demoTable .layui-btn').on('click', function(){ var type = $(this).data('type'); active[type] ? active[type].call(this) : ''; }); }); </script>
springmvc后台代码:
/** * layui-content后台代码 * @return */ @RequestMapping("/backContent") @ResponseBody public ResultMap<List<Content>> backContent(Page page,@RequestParam("limit") int limit){ System.out.println("backContent========================"+limit); page.setRows(limit); System.out.println("page:"+page.toString()); List<Content>contentList=contentService.selectPageList(page); int totals=contentService.selectPageCount(page); page.setTotalRecord(totals); return new ResultMap<List<Content>>("",contentList,0,totals); }
因为layui的数据表格需要的格式json不只是一个数据数组,而是
{
code: 0,msg: "",
count: 数据总记录数,
data: []
}
需要参数code(要为0,不然数据表格数据显示不出),msg(返回的消息),data(表格显示的数据),totals(查询到数据的总记录数),
所以用ResultMap返回数据
/** * * layui数据表格返回数据处理类 * Created by ASUS on 2018/5/19 * * @Authod Grey Wolf */ public class ResultMap<T> { private String msg; private T data; private int code; private int count; public String getMsg() { return msg; } public void setMsg(String msg) { this.msg = msg; } public T getData() { return data; } public void setData(T data) { this.data = data; } public int getCode() { return code; } public void setCode(int code) { this.code = code; } public int getCount() { return count; } public void setCount(int count) { this.count = count; } public ResultMap(String msg, T data, int code, int count) { this.msg = msg; this.data = data; this.code = code; this.count = count; } public ResultMap() { } }
Page类是我用来封装分页的数据的,比如layui的当前页数,点击下一页的值。不用类封装,也可以用springmvc的@RequestBody("page")int page 获取layui传到后台的当前页数。
/** * 处理分页 * Created by ASUS on 2018/5/7 * * @Authod Grey Wolf */ public class Page implements Serializable { //当前页 private Integer page=1; //页大小 private Integer rows=5; // 总记录 数 private Integer totalRecord; //总页数 private Integer totalPage; //关键字类型 private String keyType; //查询关键字 private String keyWord; //开始记录位置 private Integer start; //用户id private String userid; //其他用户id private String otherid; public String getKeyType() { return keyType; } public void setKeyType(String keyType) { this.keyType = keyType; } public String getOtherid() { return otherid; } public void setOtherid(String otherid) { this.otherid = otherid; } public String getUserid() { return userid; } public void setUserid(String userid) { this.userid = userid; } public Integer getPage() { return page; } public void setPage(Integer page) { this.page = page; } public Integer getRows() { return rows; } public void setRows(Integer rows) { this.rows = rows; } public Integer getTotalRecord() { return totalRecord; } public void setTotalRecord(Integer totalRecord) { this.totalRecord = totalRecord; } public Integer getTotalPage() { totalPage=(totalRecord-1)/rows+1; return totalPage; } public void setTotalPage(Integer totalPage) { this.totalPage = totalPage; } public String getKeyWord() { return keyWord; } public void setKeyWord(String keyWord) { this.keyWord = keyWord; } public Integer getStart() { start=(page-1)*rows; return start; } public void setStart(Integer start) { this.start = start; } public Page() { } public Page(Integer page, Integer rows, Integer totalRecord, Integer totalPage, String keyType, String keyWord, Integer start, String userid, String otherid) { this.page = page; this.rows = rows; this.totalRecord = totalRecord; this.totalPage = totalPage; this.keyType = keyType; this.keyWord = keyWord; this.start = start; this.userid = userid; this.otherid = otherid; } @Override public String toString() { return "Page{" + "page=" + page + ", rows=" + rows + ", totalRecord=" + totalRecord + ", totalPage=" + totalPage + ", keyType='" + keyType + '\'' + ", keyWord='" + keyWord + '\'' + ", start=" + start + ", userid='" + userid + '\'' + ", otherid='" + otherid + '\'' + '}'; } }根据所传来的参数,mapper执行相应的sql语句,返回结果集
<!-- 通过条件分页查询,返回数据集 --> <select id="selectPageList" parameterType="net.stxy.one.model.Page" resultMap="BaseResultMap" > select <include refid="Base_Column_List" /> from content <where> <if test="userid!=null and userid !=''">AND userid = #{userid}</if> <if test="otherid!='' and otherid!=null">AND userid not in ( select DISTINCT userid FROM content where userid = #{otherid} )</if> <if test="keyWord!='' and keyType=='userid' "> AND userid like '%' #{keyWord} '%' </if> <if test="keyWord!='' and keyType=='content' "> AND content like '%' #{keyWord} '%' </if> </where> order by id DESC limit #{start},#{rows} </select> <!-- 通过条件分页查询,返回总记录数 --> <select id="selectPageCount" parameterType="net.stxy.one.model.Page" resultType="java.lang.Integer"> select count(1) from content <where> <if test="userid!=null and userid !=''">AND userid = #{userid}</if> <if test="otherid!='' and otherid!=null">AND userid not in ( select DISTINCT userid FROM content where userid = #{otherid} )</if> <if test="keyWord!='' and keyType=='userid' "> AND userid like '%' #{keyWord} '%' </if> <if test="keyWord!='' and keyType=='content' "> AND content like '%' #{keyWord} '%' </if> </where> </select>