1 url地址格式
格式:schema://host:port/path?query#fragment
|--schema:协议。例如:http https ftp等
|--host:域名或IP地址
|--port:端口,http默认端口80,可以进行省略
|--path:路径,例如/abc/a/b/c
|--query:查询参数,例如:uname=lisi&age=12
|--fragment:锚点(哈希),用于定位页面的某个位置
2 异步调用
(1)异步效果分析
|--定时任务
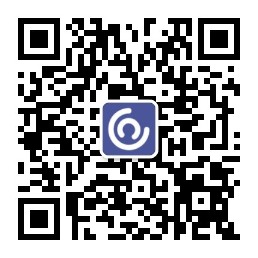
|--ajax
|--事件函数
(2)多次异步调用的以来分析
|--多次异步调用的结果额顺序是不确定的
|--异步调用结果如果存在依赖需要进行嵌套
3 Promise
(1)概述:Promise是一种异步编程的解决方案,从语法而言,Promise是一个对象,从它可以获取异步操作的消息。
(2)优点:
|--可以避免多层异步调用嵌套问题(回调地狱)
|--Promise对象提供了简介的API,使得控制异步操作更加容易
(3)基本用法
|--实例化Promise对象,构造函数中传递函数,该函数中用于处理异步任务
|--resolve和reject两个参数用于处理成功和失败两种情况,并通过p.then获取处理结果
//实例化Promise对象
var p = new Promise((resolve,reject)=>{
// 成功时调用
resolve();
// 失败时调用
reject();
});
// 进行调用
p.then(
(ret)=>{
// 从resolve得到正常结果
},
(ret)=>{
// 从reject得到错误结果
});
(4)处理ajax请求
function queryData(url) {
// 实例化Promise对象
var p = new Promise((resolve, reject) => {
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if (xhr.readyState != 4) return;
if (xhr.readyState == 4 && xhr.status == 200) {
// 处理正常情况
resolve(xhr.responseText);
} else {
// 处理异常情况
reject("服务器异常");
}
};
xhr.open("get", url);
xhr.send(null);
});
return p;
};
//单ajax请求
queryData("http://localhost:3000/data")
.then((data) => {
console.log(data);
}, (info) => {
console.log(info);
});
//多ajax请求
queryData("http://localhost:3000/data")
.then((data) => {
console.log(data);
return queryData("http://localhost:3000/data");
})
.then((data) => {
console.log(data);
return queryData("http://localhost:3000/data");
})
.then((data) => {
console.log(data);
})
(5)then参数中的函数返回值
|--返回Promise实例对象
返回的该实例对象会调用下一个then
|--返回普通值
返回的普通值会直接传递给下一个then,通过then参数中函数的参数接收这个值
| --返回Promise实例对象
返回的该实例对象会调用下一个then
| --返回普通值
返回的普通值会直接传递给下一个then,通过then参数中函数的参数接收这个值
queryData("http://localhost:3000/data").then(function (data) {
return queryData("http://localhost:/data1");
}).then((data) => {
return new Promise(function (resolve, reject) {
setTimeout(() => {
resolve(123);
}, 1000);
})
}).then((data) => {
return "hello";
}).then((data) => {
console.log(data);
})
(6)Promise常用的API
|--实例方法
|--p.then() 得到异步任务的正确结果
|--p.catch() 得到异常信息
|--p.finally() 成功与否都会执行(尚且不是正式标准)
queryData().then((data)=>{
console.log(data);
}).catch((data)=>{
console.log(data);
}).finally(()=>{
console.log("finished");
});
|--对象方法
|--Promise.all() 并发处理多个异步任务,所有任务都执行完成之后才能得到结果
Promise.all([p1, p2, p3]).then((result) => {
console.log(result);
})
|--Promise.race() 并发处理多个一部任务,只要一个任务完成就可以得到结果
Promise.race([p1, p2, p3]).then((result) => {
console.log(result);
})
(7)fetch用法
|--基本用法
fetch("http://localhost:3000/data").then(data=>{
//text()方法属于fetchAPI的一部分,它返回一个Promise实例对象,用于获取后台返回的数据
return data.text();//返回的是一个对象
}).then(ret=>{
//ret是获取的真实的数据
console.log(ret)
})
|--常用配置选项
|--method(String):http请求方式,默认为GET
|--以下是put、post必须设置的
|--body(String):http的请求参数
|--headers(Object):http的请求头,默认为{}
|--请求参数
|--get/delete
fetch("http://localhost:3000/data/123",{
method:"get"//method:"delete"
}).then((data)=>{
return data.text();
}).then(data=>{
console.log(data);
});
|--post/put
普通格式
fetch("http://localhost:3000/data/123",{
method:"post"//method:"put"
body: "uname=lisi$pwd=123",
headers:{
"Content-Type":"application/x-www-form-urlcoded"
}
}).then((data)=>{
return data.text();
}).then(data=>{
console.log(data);
});
JSON格式
fetch("http://localhost:3000/data/123",{
method:"post"//method:"put"
body: JSON.stringify{
uname:"lisi",
pwd:123
},
headers:{
"Content-Type":"application/json"
}
}).then((data)=>{
return data.text();
}).then(data=>{
console.log(data);
});
|--fetch获取的数据格式
text():返回体处理成字符串类型
json格式
fetch("http://localhost:3000/data/123").then(
(data)=>{
return data.json();
//return data.text() 使用时对应下面JSON.parse(data)
}
}).then(data=>{
console.log(data.uname);
//var obj = JSON.parse(data)
//console.log(obj.uname)
});