题目是LeetCode第183场周赛的第三题,链接:最长快乐字符串。具体描述为:如果字符串中不含有任何 ‘aaa’,‘bbb’ 或 ‘ccc’ 这样的字符串作为子串,那么该字符串就是一个「快乐字符串」。给你三个整数 a,b ,c,请你返回 任意一个 满足下列全部条件的字符串 s:
- s 是一个尽可能长的快乐字符串。
- s 中 最多 有a 个字母 ‘a’、b 个字母 ‘b’、c 个字母 ‘c’ 。
- s 中只含有 ‘a’、‘b’ 、‘c’ 三种字母。
如果不存在这样的字符串 s ,请返回一个空字符串 “”。其中:
- 0 <= a, b, c <= 100
- a + b + c > 0
示例1:
输入:a = 1, b = 1, c = 7
输出:"ccaccbcc"
解释:"ccbccacc" 也是一种正确答案。
示例2:
输入:a = 2, b = 2, c = 1
输出:"aabbc"
示例3:
输入:a = 7, b = 1, c = 0
输出:"aabaa"
解释:这是该测试用例的唯一正确答案。
这其实是一道贪心算法的题目,所以只需要“贪心”就好了。怎么个贪心法呢,就是每次总是在a,b,c三者中找到剩余次数最多的那个,加入到结果字符串中去,同时将其次数减一,需要注意的是为了防止出现出现连续3个同样字符的情况,在末尾两个字符已经一样的情况下只能从另外两个字符中选一个剩余次数最多的字符。时间复杂度为 ,空间复杂度为 。
JAVA版代码如下:
class Solution {
private int[] char2num;
private char nextChar(char exclude) {
char next;
if (exclude == 'a') {
next = char2num[1] > char2num[2] ? 'b' : 'c';
}
else if (exclude == 'b') {
next = char2num[0] > char2num[2] ? 'a' : 'c';
}
else if (exclude == 'c') {
next = char2num[0] > char2num[1] ? 'a' : 'b';
}
else {
next = char2num[0] > char2num[1] ? 'a' : 'b';
next = char2num[next - 'a'] > char2num[2] ? next : 'c';
}
return next;
}
public String longestDiverseString(int a, int b, int c) {
char2num = new int[] {a, b, c};
char[] result = new char[a + b + c];
int idx = 0;
while (char2num[0] != 0 || char2num[1] != 0 || char2num[2] != 0) {
char next;
if (idx < 2 || result[idx - 1] != result[idx - 2]) {
next = nextChar(' ');
}
else {
next = nextChar(result[idx - 1]);
}
if (char2num[next - 'a'] <= 0) {
break;
}
--char2num[next - 'a'];
result[idx++] = next;
}
return new String(result, 0, idx);
}
}
提交结果如下:
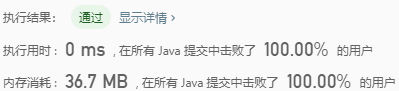
Python版代码如下:
class Solution:
def longestDiverseString(self, a: int, b: int, c: int) -> str:
char2num = {'a' : a, 'b' : b, 'c' : c}
def nextChar(exclude):
if exclude == 'a':
return 'b' if char2num['b'] > char2num['c'] else 'c'
elif exclude == 'b':
return 'a' if char2num['a'] > char2num['c'] else 'c'
elif exclude == 'c':
return 'a' if char2num['a'] > char2num['b'] else 'b'
else:
nc = 'a' if char2num['a'] > char2num['b'] else 'b'
return nc if char2num[nc] > char2num['c'] else 'c'
result = ''
while char2num['a'] > 0 or char2num['b'] > 0 or char2num['c'] > 0:
L = len(result)
nc = 'a'
if L < 2 or result[L - 1] != result[L - 2]:
nc = nextChar(' ')
else:
nc = nextChar(result[L - 1])
if char2num[nc] <= 0:
break
char2num[nc] -= 1
result += nc
return result
提交结果如下:
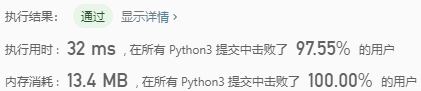