ncstoj 1354成绩排名
Description
读入n名学生的姓名、学号、成绩,分别输出成绩最高和成绩最低学生的姓名和学号。
Input
每个测试输入包含1个测试用例,第一行n代表学生的个数(0 < n < 100)
Output
对每个测试用例输出2行,第1行是成绩最高学生的姓名和学号,第2行是成绩最低学生的姓名和学号,字符串间有1空格。
Sample Input
3
Joe Math990112 89
Mike CS991301 100
Mary EE990830 95
Sample Output
Mike CS991301
Joe Math990112
结构体入门题
C++写法1:
#include <bits/stdc++.h>
using namespace std;
const int maxn = 105;
typedef struct STU {
string name;
string num;
int score;
bool operator<(const STU &S) const { return score < S.score; }
} STU;
STU s[maxn];
int main() {
int n;
cin >> n;
for (int i = 0; i < n; i++)
cin >> s[i].name >> s[i].num >> s[i].score;
sort(s, s + n);
cout << s[n - 1].name << " " << s[n - 1].num << endl;
cout << s[0].name << " " << s[0].num << endl;
return 0;
}
C++写法2与C语言对比:
ncstoj 1598单词排序
Description
输入一长串字符串,将其中的单词按字典序输出。
Input
输入由K个英文单词组成的字符串,1≤K≤20。
保证每个字母均由小写字母构成,且每个单词至多出现一次。
Output
将全部单词按字典序输出,每两个单词之间由空格隔开
Sample Input
tom likes apples
Sample Output
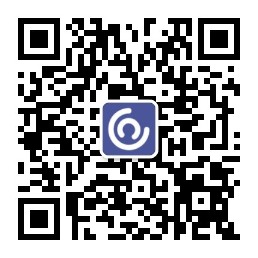
apples likes tom
STL set:使用集合排序并去重
编译出问题的同学,请看这个视频3分50秒: 编程环境配置与OJ介绍
#include <bits/stdc++.h>
using namespace std;
set<string> s;
int main() {
int n;
string str;
while (cin >> str) {
s.insert(str);
}
for (auto elem : s)
cout << elem << " ";
return 0;
}
学妹写的C语言做法(她这个输入过程写复杂了)
写法4: sort+vector
#include <algorithm>
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<string> v;
string str;
while (cin >> str) {
v.push_back(str);
}
sort(v.begin(), v.end());
for (auto x : v) {
cout << x << " ";
}
puts("");
return 0;
}
ncstoj 1599相对分子质量
Description
小明在大学里学习了编程以后,决定要解决一些高中时期最困惑他的问题之一:计算化学物质相对分子质量。
Input
输入的第一行是一个正整数n,表示有n组测试数据。
接下来n行每行输入一个字符串,表示某个分子式,分子式中只包含大写字母和数字。
注意:输入数据只包含3种元素,而这3种元素的相对原子质量如下:H(1),C(12),O(16)。
Output
对于每组输入,输出相对分子质量。
Sample Input
2
H2O
CH4
Sample Output
18
16
这道题算法很多,怎么做都行
通过哈希表unordered_map(或map)构建查找元素表
#include <iostream>
#include <unordered_map>
using namespace std;
unordered_map<char, int> m1;
bool isDigit(char c) { return c <= '9' && c >= '1'; }
int main() {
// freopen("/Users/zhbink/Documents/C++/C++/in.txt", "r", stdin);
m1['H'] = 1;
m1['C'] = 12;
m1['O'] = 16;
int T;
cin >> T;
while (T--) {
string str;
cin >> str;
int ans = 0;
char last_alp = 'a';
for (int i = 0; i < str.length(); i++) {
char c = str[i];
if (isDigit(c))
ans += m1[last_alp] * (c - '0');
else
ans += m1[last_alp];
last_alp = c;
}
if (!isDigit(str.back()))
ans += m1[last_alp];
cout << ans << endl;
}
return 0;
}
学弟写的算法二:
#include<iostream>
using namespace std;
int main(){
int n,num,sum;
string a;
cin>>n;
while(n--){
sum=0;
cin>>a;
for(int i=0;i<a.length();i++){
if(a[i]=='H'){
num=1;
sum+=1;
}else if(a[i]=='O'){
num=16;
sum+=16;
}else if(a[i]=='C'){
num=12;
sum+=12;
}else if(a[i]<60){
sum+=num*(a[i]-'0'-1);
}
}
cout<<sum<<endl;
}
return 0;
}
算法二的C语言版:
注意用getchar()吃换行符