14、
【{!expression}与{#expression}的比较】:
Using Expressions |
Data Binding Between Components
a、{!expression}数据双向绑定 - bound expressions,任何地方改变,所有相关的引入位置都随着改变;
b、{!#expression} - unbond expressions,作用在于赋初始值,之后被引用地相互不影响;
c、sample:exprApp.app / parentExpr.cmp | parentExprController.js / childExpr.cmp | childExprController.js
13、 【component中使用if-else条件表达式渲染组件】: 官方文档 | aura:set | {!v.body} | body in js | Conditional Expressions
12、 【通过js添加/移除CSS】: Adding and Removing Styles
a、overview:我们可以使用 行内CSS/也可以从static resource中 引用外部CSS / 创建STYLE tab 使用CSS;
b、particular attention:分清Top Level Elements/Nested Elements/All Top Level Elements With Class/All Nested Level Elements With Class/Elements With Unique ID;
c、sample:
5、remarks:从上面示例我们可以看到,从component bundle创建STYLE时, 支持css3选择器,并且 无法为html和body设置样式 (试想如果组件能设置body,那么如果组件被嵌套,且有各自的body,此时会乱套,违背统一设计原则),如果设置 .THIS样式,会 作用于组件中的 所有标签上。
10、 【在comonent中使用custom label】: Using Labels
在lightning中使用自定义标签和classic方式不一样
a、使用默认命名空间的标记表达式中的标签:
controller.js:
9、 【为component添加slds库里面的标准token】 :Standard Design Tokens | SLDS-Design-Tokens | Blog Reference
a、新建一个Lightning Tokens,如果是第一次创建token名字必须为 defaultTokens;
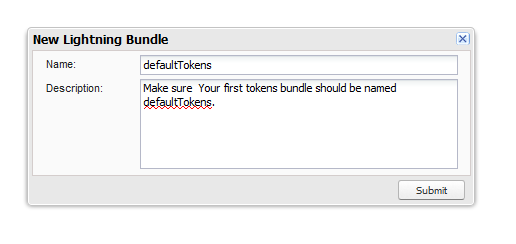
CSS如下:font-weight:token(fontWeightBold)或者t(fontWeightBold)都行;
8、 【如何实现在controller.js中定义全局变量计数的效果】:
我们知道controller.js中的method为json格式,假如想统计点击按钮的次数,是无法在json中定义全局变量的,因此,我们可以在component中通过属性来存放计数数量,然后每次点击按钮后先取属性变量,然后累加后,把值赋给它即可达到目的。
7、 【Javascript API reference in developing lightning component】: Using Javascript
a、概述:lightning组件在开发时,客户端会使用Js做交互,因此了解$A namespace | Util / Event / Component / Action / AuraLocalizationService Class及对应的方法就显得极为重要。
b、查看API方法: https://<myDomain>.lightning.force.com/auradocs/reference.app。
c、常用的方法总结:待续......
6、 【<aura:attribute />的type属性全集】: Basic Types | Function Type | Object Types | Sobject Types | Collection Types | ApexClass Types | Framework-Specific Types
5、 【在Lightning Component中使用Icons的几种方法】:
a、使用lightning:icon标签取代svg/use标签,示例如下: Icons | lightning:icon
扩展:如何自定义图标颜色和大小?
思路:svg有个属性叫做fill,可以通过改变该值来控制svg颜色,对于符合型的icon,可结合父元素的background与fill属性来控制整体颜色。
实例如下:
a、 最简单的官方推荐的方式是扩展extends: force:slds,扩展后css将自动更新为官方最新版本,具体用法如下:
Zip类型的引用:
3、 【Visualforce页面在手机端自适应的方式】 :对外层容器设置最大宽度,并水平居中
a、将包含slds的样式的代码放入<apex:slds>里面;
b、将样式上传到静态资源,之后再使用<apex:stylesheet>引用;
1、 【Path Settings】:在商机记录类型中看到有Sales Process这一列,起初不知道啥意思,如下图,后面才知道这个主要用在Lightning版本,会以甘特图形式展示当前单子的进度或状态。 并不是所有对象都可以设置 。
效果如下:
参考资料:
https://releasenotes.docs.salesforce.com/en-us/winter16/release-notes/rn_sales_sales_path_sfx.htm
https://trailhead.salesforce.com/en/modules/lex_migration_whatsnew/units/lex_migration_whatsnew_crm
a、{!expression}数据双向绑定 - bound expressions,任何地方改变,所有相关的引入位置都随着改变;
b、{!#expression} - unbond expressions,作用在于赋初始值,之后被引用地相互不影响;
c、sample:exprApp.app / parentExpr.cmp | parentExprController.js / childExpr.cmp | childExprController.js
<!--c:exprApp--> <aura:application > <c:parentExpr /> </aura:application>
<!--c:parentExpr--> <aura:component> <aura:attribute name="parentAttr" type="String" default="parent attribute"/> <!-- Instantiate the child component --> <c:childExpr childAttr="{!v.parentAttr}" /><!--situation1--> <!--<c:childExpr childAttr="{#v.parentAttr}" />--><!--situation2--> <p>parentExpr parentAttr: {!v.parentAttr}</p> <p><lightning:button label="Update parentAttr" onclick="{!c.updateParentAttr}"/></p> </aura:component>
<!--c:childExpr--> <aura:component> <aura:attribute name="childAttr" type="String"/> <p>childExpr childAttr: {!v.childAttr}</p> <p><lightning:button label="Update childAttr" onclick="{!c.updateChildAttr}"/></p> </aura:component>
/* parentExprController.js */ ({ updateParentAttr: function(cmp) { cmp.set("v.parentAttr", "updated parent attribute"); } })
/* childExprController.js */ ({ updateChildAttr: function(cmp) { cmp.set("v.childAttr", "updated child attribute"); } })d、preview:
- situation1和situation2在页面初始化时均为下图:
- situation2点击Update childAttr时如下图:
- situation2点击Update parentAttr时如下图:
- situation1点击先后点击两个按钮均为下图:
13、 【component中使用if-else条件表达式渲染组件】: 官方文档 | aura:set | {!v.body} | body in js | Conditional Expressions
<aura:component> <aura:attribute name="display" type="Boolean" default="false"/> <aura:if isTrue="{!v.display}"> Show this if condition is true <aura:set attribute="else"> <ui:button label="Save" press="{!c.saveRecord}" /> </aura:set> </aura:if> </aura:component>效果说明:如果display为true将仅展示"Show this if condition is true",否则仅展示button。
12、 【通过js添加/移除CSS】: Adding and Removing Styles
{ applyCSS: function(cmp, event) { var cmpTarget = cmp.find('changeIt'); $A.util.addClass(cmpTarget, 'changeMe'); }, removeCSS: function(cmp, event) { var cmpTarget = cmp.find('changeIt'); $A.util.removeClass(cmpTarget, 'changeMe'); } }11、 【在lightning component中使用CSS】: 3 Ways to Use CSS
a、overview:我们可以使用 行内CSS/也可以从static resource中 引用外部CSS / 创建STYLE tab 使用CSS;
b、particular attention:分清Top Level Elements/Nested Elements/All Top Level Elements With Class/All Nested Level Elements With Class/Elements With Unique ID;
c、sample:
<aura:component implements="force:appHostable"> <p>PI的值为:{!v.pi}</p> <h3 class="title">testing design token</h3> <div class="wrap"> <ul id="ul"> <li>Digital</li> <li class="impt">Salesforce</li> <li>SAP</li> </ul> </div> </aura:component>
.THIS { } .THIS p {/*invalid*/ color: #666; } p.THIS { font-weight: t(fontWeightBold); background: token(colorBackgroundInverse); color: #fff; } .THIS h3 {/*invalid*/ color: 00f; } h3.THIS { color: #f00; } .THIS .title {/*invalid - h3的class*/ font-weight: bold; } .title.THIS { font-weight: bold; } .THIS .wrap {/*invalid*/ background: #0f0; padding: 5px; } .wrap.THIS { background: #0f0; padding: 5px; } .THIS ul { border: 2px solid #000;; } .THIS #ul { background: #f0f; } .THIS li { margin-top: 5px; } .THIS li.impt { font-weight: 700; } .THIS li:last-of-type {/*支持css3选择器*/ margin-bottom: 5px; } .THIS ul>li { color: #00f; }4、preview:
5、remarks:从上面示例我们可以看到,从component bundle创建STYLE时, 支持css3选择器,并且 无法为html和body设置样式 (试想如果组件能设置body,那么如果组件被嵌套,且有各自的body,此时会乱套,违背统一设计原则),如果设置 .THIS样式,会 作用于组件中的 所有标签上。
10、 【在comonent中使用custom label】: Using Labels
在lightning中使用自定义标签和classic方式不一样
a、使用默认命名空间的标记表达式中的标签:
{!$Label.c.labelName}b、组织拥有命名空间时Javascript代码中的标签:
$A.get("$Label.namespace.labelName")c、针对第二种情况示例如下:
controller.js:
({ doInit : function(component, event, helper) { var pi = $A.get("$Label.DTT.PI"); component.set('v.pi', pi); } })component:
<aura:component implements="force:appHostable"> <aura:attribute name="pi" type="String"/> <aura:handler name="init" value="{!this}" action="{!c.doInit}"/> <p>PI的值为:{!v.pi}</p> </aura:component>preview:
9、 【为component添加slds库里面的标准token】 :Standard Design Tokens | SLDS-Design-Tokens | Blog Reference
a、新建一个Lightning Tokens,如果是第一次创建token名字必须为 defaultTokens;
<aura:tokens extends="force:base"> </aura:tokens>b、在CSS里面直接使用即可,假如我们想使用下图的样式:
CSS如下:font-weight:token(fontWeightBold)或者t(fontWeightBold)都行;
.THIS .slds-form-element__label { font-size: 0.92rem; color: #0070d2; font-weight: t(fontWeightBold); }c、使用token意义:待续......
8、 【如何实现在controller.js中定义全局变量计数的效果】:
我们知道controller.js中的method为json格式,假如想统计点击按钮的次数,是无法在json中定义全局变量的,因此,我们可以在component中通过属性来存放计数数量,然后每次点击按钮后先取属性变量,然后累加后,把值赋给它即可达到目的。
7、 【Javascript API reference in developing lightning component】: Using Javascript
a、概述:lightning组件在开发时,客户端会使用Js做交互,因此了解$A namespace | Util / Event / Component / Action / AuraLocalizationService Class及对应的方法就显得极为重要。
b、查看API方法: https://<myDomain>.lightning.force.com/auradocs/reference.app。
c、常用的方法总结:待续......
6、 【<aura:attribute />的type属性全集】: Basic Types | Function Type | Object Types | Sobject Types | Collection Types | ApexClass Types | Framework-Specific Types
5、 【在Lightning Component中使用Icons的几种方法】:
a、使用lightning:icon标签取代svg/use标签,示例如下: Icons | lightning:icon
<lightning:icon iconName="action:approval" size="large" alternativeText="Indicates approval"/> <lightning:icon iconName="action:add_contact" size="large" alternativeText="Indicates add contact"/> <lightning:icon iconName="custom:custom1" size="large" alternativeText="Indicates custom1"/> <lightning:icon iconName="doctype:attachment" size="large" alternativeText="Indicates attachment"/> <lightning:icon iconName="standard:account" size="large" alternativeText="Indicates account"/> <lightning:icon iconName="utility:announcement" size="large" alternativeText="Indicates announcement"/>注意:iconName属性值的 icon类型部分必须小写,首字母大写会报undefined的错。
扩展:如何自定义图标颜色和大小?
思路:svg有个属性叫做fill,可以通过改变该值来控制svg颜色,对于符合型的icon,可结合父元素的background与fill属性来控制整体颜色。
实例如下:
<aura:application extends="force:slds"> <lightning:icon class="icon1" iconName="custom:custom1" title="custom1" size="large" alternativeText="Indicates heart"/> </aura:application>
.THIS .icon1{ background:transparent !important; } .THIS .icon1 svg{ width: 4rem;/* large => 3rem */ height: 4rem; fill: #e92444; border: 1px solid #f00; }4、 【Application引入Lightning Design System】 :使用后,亦可直接在component中使用lightning design system css.
a、 最简单的官方推荐的方式是扩展extends: force:slds,扩展后css将自动更新为官方最新版本,具体用法如下:
<aura:application extends="force:slds"> <!-- customize your application here --> </aura:application>b、使用 static source方式指定某一版本的css, 推荐命名规范:SLDS+版本号(如:SLDS260 - Version 2.6.0),方便归档,在application/component中引用方式如下:
Zip类型的引用:
<aura:component> <ltng:require styles="{!$Resource.SLDS252 + '/styles/salesforce-lightning-design-system.min.css'}" /> </aura:component>对单独上传到静态资源的css文件的引用:
<ltng:require styles="{!$Resource.DTT__lightningCss}" />参考资料: https://developer.salesforce.com/docs/atlas.en-us.lightning.meta/lightning/apps_slds.htm
3、 【Visualforce页面在手机端自适应的方式】 :对外层容器设置最大宽度,并水平居中
<meta charset="utf-8"/> <meta http-equiv="X-UA-Compatible" content="IE=edge"/> <meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=no"/>2、 【Visualforce引入Lightning Design System的两种方式】: slds | bootstrap
a、将包含slds的样式的代码放入<apex:slds>里面;
b、将样式上传到静态资源,之后再使用<apex:stylesheet>引用;
1、 【Path Settings】:在商机记录类型中看到有Sales Process这一列,起初不知道啥意思,如下图,后面才知道这个主要用在Lightning版本,会以甘特图形式展示当前单子的进度或状态。 并不是所有对象都可以设置 。
效果如下:
参考资料:
https://releasenotes.docs.salesforce.com/en-us/winter16/release-notes/rn_sales_sales_path_sfx.htm
https://trailhead.salesforce.com/en/modules/lex_migration_whatsnew/units/lex_migration_whatsnew_crm