该题是LeetCode 4月组织的30天挑战赛,是第一题。
该题是easy,比较简单,但效率比较高有难度,方案还有待继续提高。
题目相关
【题目解读】
从一个给定的非空数组中找出只出现一次的元素。
【原题描述】原题链接
Given a non-empty array of integers, every element appears twice except for one. Find that single one.
Note:
Your algorithm should have a linear runtime complexity. Could you implement it without using extra memory?
Example 1:
Input: [2,2,1]
Output: 1
Example 2:
Input: [4,1,2,1,2]
Output: 4
【难度】Easy
Solution
以此写下自己在解决该问题时想到的几种解决方法,前面两种都比较直观
- 从前往后以此遍历数组,每一个元素都去后面的元素中进行查找,看是否存在。该算法的时间复杂度为 ,没采用。这里的查找可以利用STL的find函数
- 利用map,将元素作为key,出现次数作为value,遍历数组,遍历数组时,以此插入到map中,最后便利所获得的map,再找出value为1的元素。该方法的时间复杂度为 ,空间复杂度也为 ,暂未采用。
- 运用STL的sort函数对原数组进行排序,之后遍历数组,进行逐元素比较。
在提交的版本中采用了上面的第三种先排序在查找的方法,代码如下,但效果不好,只达到了7%。
#include <algorithm>
class Solution {
public:
int singleNumber(vector<int>& nums) {
std::sort(nums.begin(), nums.end());
size_t len = nums.size();
if(len == 1)
return nums[0];
size_t i=0;
while(i< len-1)
{
if(nums[i] == nums[i+1])
i += 2;
else return nums[i];
}
// 数组中最大元素就是要找的元素的情况
return nums[i];
}
};
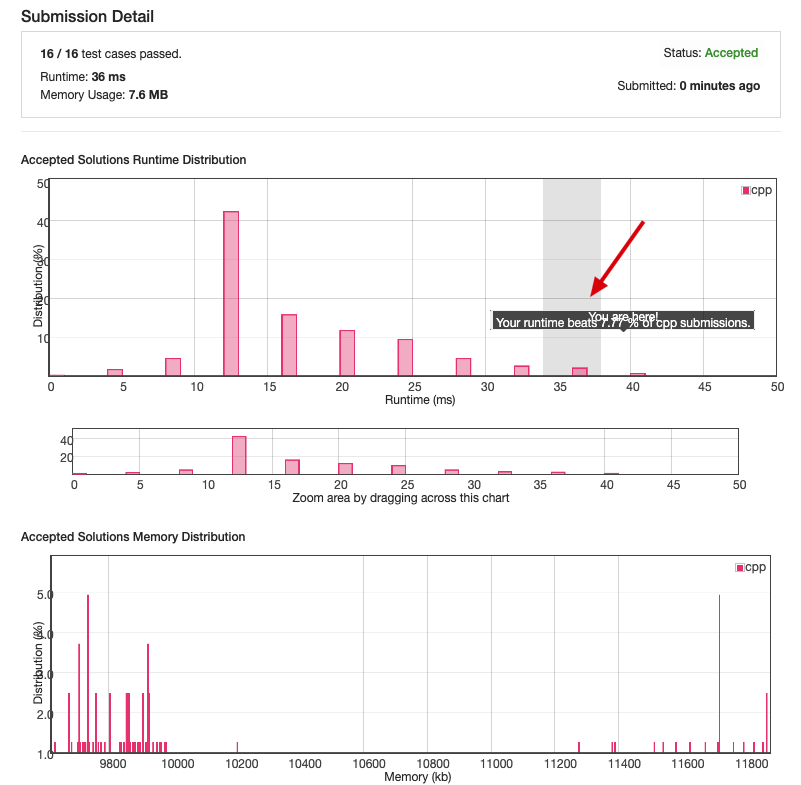
使用map
该方法未完成,后面完善下该方法
#include <set>
class Solution {
public:
int singleNumbert(vector<int>& nums) {
map<int, int> mymap;
for(size_t i = 0; i < nums.size(); i++)
{
}
}
};