简介
上一节学习了配置管理中心Config,如果在实际生产环境中,众多微服务只用一个配置中心,那显然是不合理的,一旦这个配置中心挂掉,将会影响其所提供的服务,影响其它微服务不可用。那么这一节,我们基于上节学习的代码来把Config改造成高可用的集群模式。
创建Eureka注册中心
由于集群的Config配置中心需要通信,所以我们需要配置Eureka Server,新建一个Eureka Server的项目模块,之前学习过Eureka Server的相关配置,我们把POM文件直接复制拿过来用,改一改名称之类
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.dothwinds</groupId>
<artifactId>spring-cloud-study-config</artifactId>
<version>1.0.0</version>
</parent>
<groupId>org.dothwinds</groupId>
<artifactId>spring-cloud-study-eureka-server</artifactId>
<version>1.0.0</version>
<name>spring-cloud-study-eureka-server</name>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-server</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
</project>
然后把配置文件application.yml的内容也复制过来
spring:
application:
name: eureka-server
server:
port: 7001
eureka:
client:
fetch-registry: false #是否需要去注册中心获取其它服务地址,集群的时候要用,单server不用
register-with-eureka: false #是否去eureka注册,本身就是服务中心,无需注册
service-url:
defaultZone: http://localhost:8101/eureka #指向与本地实例相同的主机。
instance:
hostname: localhost
之后修改启动类,加入@EnableEurekaServer注解
package org.dothwinds.springcloudstudyeurekaserver;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer;
@SpringBootApplication
@EnableEurekaServer
public class SpringCloudStudyEurekaServerApplication {
public static void main(String[] args) {
SpringApplication.run(SpringCloudStudyEurekaServerApplication.class, args);
}
}
改造Config Server工程模块
主要改造的目的是:可以指向Eureka Server,也就是把Config Server变成跟其他微服务一样,注册到Eureka Server中去。
首先修改POM,引入Eureka Client需要的包
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.dothwinds</groupId>
<artifactId>spring-cloud-study-config</artifactId>
<version>1.0.0</version>
</parent>
<groupId>org.dothwinds</groupId>
<artifactId>spring-cloud-study-service-config</artifactId>
<version>1.0.0</version>
<name>spring-cloud-study-service-config</name>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-config-server</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
</dependencies>
</project>
启动类加入@EnableEurekaClient的注解
package org.dothwinds.springcloudstudyserviceconfig;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.config.server.EnableConfigServer;
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
@SpringBootApplication
@EnableConfigServer
@EnableEurekaClient
public class SpringCloudStudyServiceConfigApplication {
public static void main(String[] args) {
SpringApplication.run(SpringCloudStudyServiceConfigApplication.class, args);
}
}
修改配置文件application.yml,加入指向的Eureka Server
spring:
application:
name: config-server
cloud:
config:
server:
git:
uri: https://gitee.com/dothwinds/spring-cloud-config-server #git服务器地址,这里我们在gitee上建立一个仓库
username: #访问此GIT地址所用用户名
password: #访问此GIT地址所用密码
search-paths: configs #git目录下存放的文件夹
label: master #GIT仓库所用分支名称
server:
port: 12000
eureka:
client:
service-url:
defaultZone: http://localhost:7001/eureka
改造Config Client工程模块
改造的想法及方式同Config Server,修改POM引入Eureka client的依赖;修改启动类加入@EnableEurekaClient注解;修改配置文件application.yml指向Eureka Server,详细代码请去文后下载获取。
验证
依次启动Eureka Server、Config Server和Config Client测试。
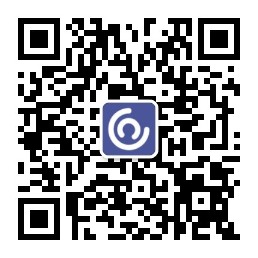
那么我们修改Config Server端口,再次启动一个Config Server,如下图:
在此刷新访问接口,返回值没有问题,那么我们把最早启动Config Server关闭掉,可以看到仅剩的Config Server依旧会同步文件
Adding property source: file:/C:/Users/ADMINI~1/AppData/Local/Temp/config-repo-8148845676945610893/configs/config-client-dev.yml
参考资料:https://cloud.spring.io/spring-cloud-static/Greenwich.SR5/single/spring-cloud.html
代码地址:https://gitee.com/dothwinds/Spring-Cloud-Study/tree/master/spring-cloud-study-config