Problem
On a N * N grid, we place some 1 * 1 * 1 cubes.
Each value v = grid[i][j] represents a tower of v cubes placed on top of grid cell (i, j).
Return the total surface area of the resulting shapes.
Example1
Input: [[2]]
Output: 10
Example2
Input: [[1,2],[3,4]]
Output: 34
Example3
Input: [[1,0],[0,2]]
Output: 16
Example4
Input: [[1,1,1],[1,0,1],[1,1,1]]
Output: 32
Example5
Input: [[2,2,2],[2,1,2],[2,2,2]]
Output: 46
Solution
对于每一个grid[i][j] ,如果grid[i][j]大于零,那么其表面积就是2 + 4 * grid[i][j]。
但是grid[i-1][j]和grid[i][j-1],即左侧和上侧会造成遮挡,要减去遮挡面积 min(grid[i][j],grid[i-1][j]) *2 和min(grid[i][j],grid[i][j-1]) *2。
class Solution {
public:
int surfaceArea(vector<vector<int>>& grid) {
if(grid.empty())
return 0;
int r = grid.size();
int c = grid[0].size();
int surface_area = 0;
for(int i = 0;i<r;++i)
{
for(int j = 0;j<c;++j)
{
int cubes = grid[i][j];
if(cubes > 0)
{
surface_area += (2 + cubes * 4);
if(i - 1 >= 0)
{
surface_area -= (min(cubes,grid[i-1][j]) * 2);
}
if(j - 1 >= 0)
{
surface_area -= (min(cubes,grid[i][j-1]) * 2);
}
}
}
}
return surface_area;
}
};
Ref
扫描二维码关注公众号,回复:
10228912 查看本文章
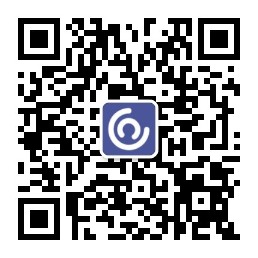