Chapter 1 练习题
环境: CLion + MinGW
1.1 查阅所使用的编译器的文档,确定它所使用的文件命名约定,编译并运行第二页的main程序
略
1.2 改写程序,让它返回-1。返回值-1通常被当作程序错误的标识。重新编译并运行你的程序,观察你的系统如何处理main返回的错误标识
int main(){
return -1;
}
g++ main.cpp
a.exe
echo %ERRORLEVEL%
-1
命令行运行结果,查看状态得到-1
1.3 编写程序,在标准输出上打印Hello, World
#include <iostream>
int main(){
std::cout << "Hello, World" << std::endl;
return 0;
}
1.4 我们的程序使用假发运算符+来将两个数相加,编写程序使用乘法运算符*,来打印两个数的积
#include <iostream>
int main(){
int a = 0, b = 0;
std::cin >> a >> b;
std::cout << "The product of " << a << " and " << b
<< " is " << a * b << std::endl;
return 0;
}
1.5 我们将所有输出操作放在一条很长的语句中,重写程序将每个运算对象的打印操作放在一条独立语句中
略
1.6 解释下面程序片段是否合法,若不合法应如何修正
std::cout << "The sum of " << v1;
<< " and " << v2;
<< " is " << v1 + v2 << std::endl;
不合法,可在第二三行开头加上std::cout
,构成三条语句,或去掉第一二行末尾分号,合成一条语句
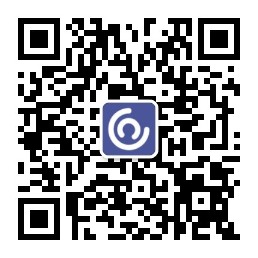
1.7 编译一个包含不正确的嵌套注释的程序,观察编译器返回的错误信息
int main(){
/*
*/* incorrect comment*/
*
*/
return 0;
}
In function 'int main()':
error: 'incorrect' was not declared in this scope
*/* incorrect comment*/
由于嵌套使用界定符对,使得incorrect comment被视为注释外的语句
1.8 指出下列那些输出语句是合法的
std::cout << "/*";
std::cout << "*/";
std::cout << /*"*/" */;
std::cout << /*"*/" /* "/*" */;
(1) 合法,打印/*
(2) 合法,打印*/
(3) 非法,相当于std::cout << "*/;
(4) 合法,相当于std::cout << " /* ";
,打印 /*
1.9 编写程序,使用while循环将50到100的整数相加
#include <iostream>
int main(){
int i = 50, sum = 0;
while(i <= 100){
sum += i;
i += 1;
}
std::cout << sum << std::endl;
return 0;
}
1.10 除了++运算符将运算对象的值增加1之外,还有一个递减运算符(–)实现将值减少1。编写程序,使用递减运算符将在循环中按递减顺序打印出10到0之间的整数
#include <iostream>
int main(){
int i = 10;
while(i >= 0){
std::cout << i << " ";
i --;
}
std::cout << std::endl;
return 0;
}
1.11 编写程序,提示用户输入两个整数,打印出这两个整数所指定的范围内的所有整数
#include <iostream>
int main(){
int low, high;
std::cout << "Enter two numbers: ";
std::cin >> low >> high;
if(low > high){ // 若用户按降序输入, 则调换两个变量的值
int tmp = low;
low = high;
hight = tmp;
}
int i = low;
while(i <= high){
std::cout << i << " ";
i ++;
}
std::cout << std::endl;
return 0;
}
1.12 下面的for循环完成了什么功能?sum的终值是什么?
int sum = 0;
for(int i = -100; i <= 100; ++i)
sum += i;
对[-100, 100]之间的整数求和,sum的终值为0
1.13 使用for循环重做1.4.1节中的所有练习
略
1.14 对比for循环和while循环,两种形式的优缺点各是什么?
循环次数已知则使用for循环,更为简洁易懂,循环次数未知或用特定条件控制循环则使用while,更为灵活
1.16 编写程序,从cin读取一组数,输出其和
#include <iostream>
int main(){
int val = 0, sum = 0;
while(std::cin >> val){
sum += val;
}
std::cout << sum << std::endl;
return 0;
}
1.17 如果输入的所有值都是相等的,本节的程序会输出什么?如果没有重复值,输出又会是怎样的?
#include <iostream>
int main(){
int val = 0, cur = 0, cnt = 1;
if(std::cin >> val){
while(std::cin >> cur){
if(cur == val){
cnt += 1;
}else{
std::cout << val << " occurs " << cnt <<" times." << std::endl;
val = cur; // reset val, count
cnt = 1;
}
}
std::cout << val << " occurs " << cnt <<" times." << std::endl;
}
return 0;
}
所有输入值一样时
1 1 1 1 1 1
^D
1 occurs 6 times.
没有重复值时
1 2 3 4 5
1 occurs 1 times.
2 occurs 1 times.
3 occurs 1 times.
4 occurs 1 times.
^D
5 occurs 1 times.
1.18 同上
1.19 修改为1.4.1节练习所编写的程序,使其能处理用户输入的第一个数比第二个数小的情况
练习1.11程序已具备该功能
1.20 拷贝官方网站的头文件Sales_item.h,并用它编写一个程序,读取一组书籍销售记录,将每条记录打印到标准输出上
# include <iostream>
# include "Sales_item.h"
using namespace std;
int main()
{
Sales_item book;
while(cin >> book){
cout << book << endl;
}
return 0;
}
1.21 编写程序,读取两个ISBN相同的Sales_item对象,输出它们的和
# include <iostream>
# include "Sales_item.h"
using namespace std;
int main()
{
Sales_item book1, book2;
cin >> book1 >> book2;
if(book1.isbn() == book2.isbn()){
cout << book1 + book2 << endl;
return 0;
}else{
cout << "The books' ISBNs must be the same." << endl;
return -1;
}
}
1.22 编写程序,读取多个ISBN相同的Sales_item对象,输出它们的和
# include <iostream>
# include "Sales_item.h"
using namespace std;
int main()
{
Sales_item book, total;
if(cin >> total){
while(cin >> book){
if(book.isbn() == total.isbn()){
total += book;
}else{
cout << "The books' ISBNs must be the same." << endl;
return -1;
}
}
cout << total << endl;
return 0;
}else{
cout << "No data." << endl;
return -1;
}
}
1.23 编写程序,读取多条销售记录,并统计每个ISBN有几条销售记录
# include <iostream>
# include "Sales_item.h"
using namespace std;
int main()
{
Sales_item total;
if(cin >> total){
Sales_item currBook;
while(cin >> currBook){
if(currBook.isbn() == total.isbn())
total += currBook;
else{
cout << total << endl;
total = currBook;
}
}
cout << total << endl;
return 0;
}else{
cerr << "No data" << endl;
return -1;
}
}