foreword
This section contains a small example of getting started with torch
The line-by-line code video explanation is at: https://www.bilibili.com/video/BV1nS4y1u76S?spm_id_from=333.999.0.0
Mainly about linear regression examples
Create your own data
Build a Linear Regression Model
complete the training process
Drawing display
Linear model
where k is the weight and b is the bias term.
In general, the linear model is to fit the k and b of which are actually w and bias
like here
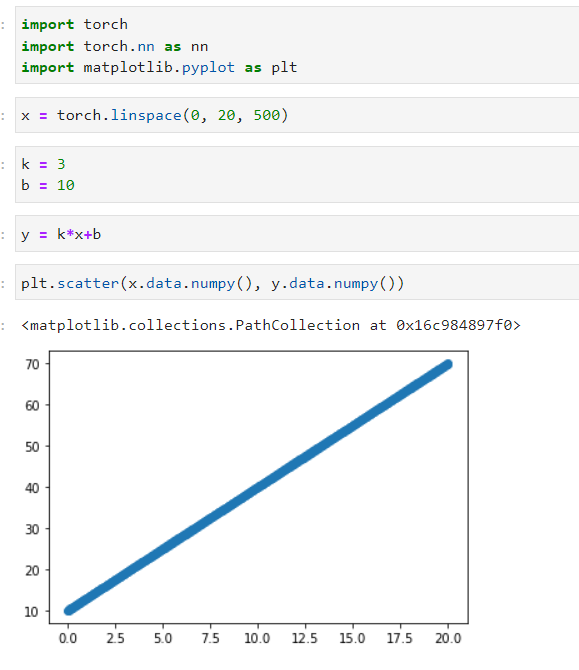
code as a whole
Simulation data
Add Gaussian white noise (a group of random numbers that conform to a normal distribution with a mean of 0 and a variance of 1), and set x to 512 points, that is, the number of samples is 512
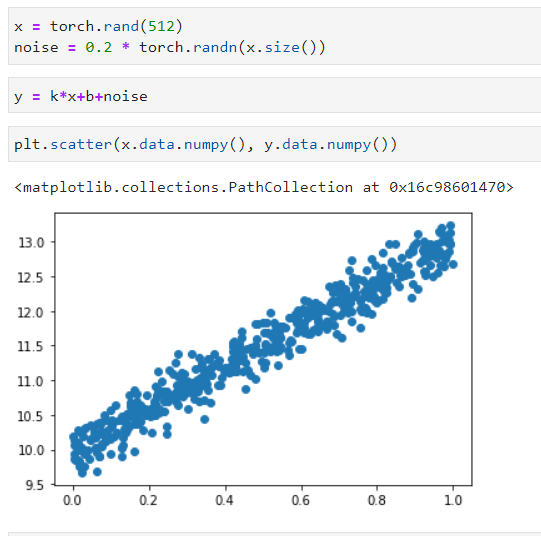
Linear model
Because each value of the input and each value of the output is actually a dimension of 1, feature_num=1, the linear model is
class LinearModel(nn.Module):
def __init__(self, in_fea, out_fea):
super(LinearModel, self).__init__()
self.out = nn.Linear(in_fea, out_fea)
def forward(self, x):
x = self.out(x)
return x
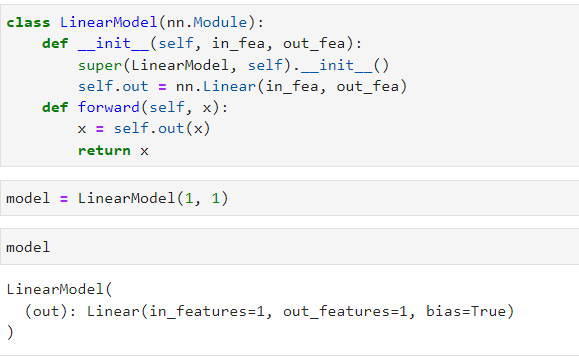
Define loss function and optimizer
optimizer = torch.optim.SGD(model.parameters(), lr=0.02)
loss_func = nn.MSELoss()
Change the dimension of the data to the model input dimension
Add one dimension to the dimension of the feature
training and visualization
The routine is
forward reasoning
算loss
clear gradient
backpropagation
update weights
plt.ion()
for step in range(200):
prediction = model(x)
loss = loss_func(prediction, y)
optimizer.zero_grad()
loss.backward()
optimizer.step()
if step%10 == 0:
plt.cla()
plt.scatter(x.data.numpy(), y.data.numpy())
plt.plot(x.data.numpy(), prediction.data.numpy(), 'r-', lw=5)
plt.xlim(0,1.1)
plt.ylim(0, 20)
[w, b] = model.parameters()
plt.text(0, 0.5, 'loss=%.4f, k=%.2f, b=%2f'%(loss.item(), w.item(), b.item() ),fontdict={'size': 20, 'color': 'red'})
plt.pause(0.5)
plt.ioff()
plt.show()
Among them, ion is to open the interactive mode, and ioff is to close the interactive mode, and you can dynamically draw the picture to see the changes.
Dynamically look at the fitted line transformation
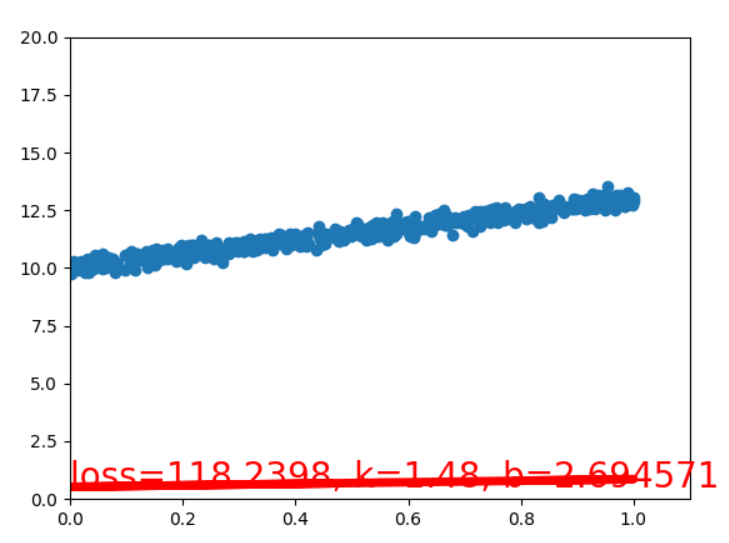
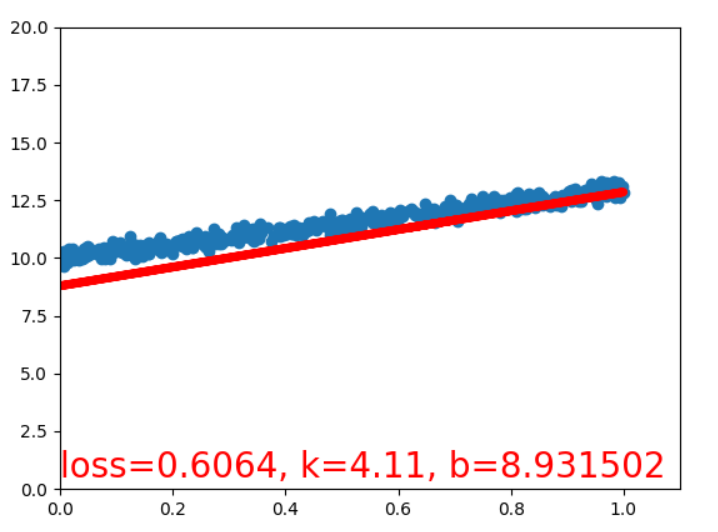
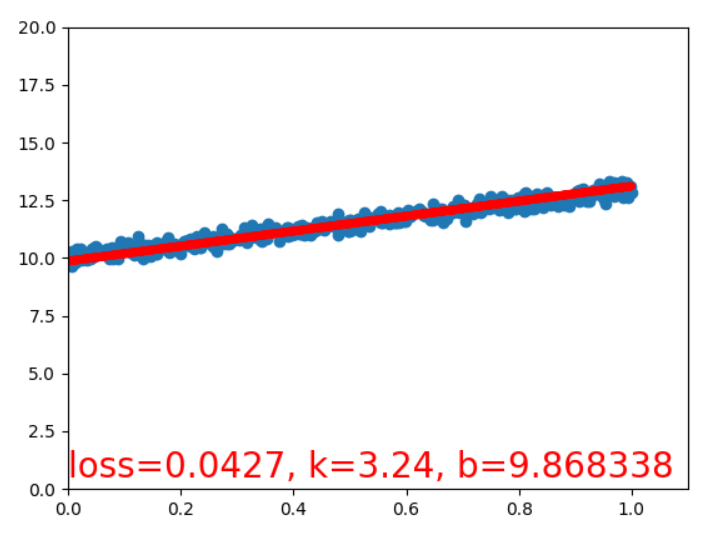
Recommended reading:
My 2022 Internet School Recruitment Sharing
Talking about the difference between algorithm post and development post
Internet school recruitment research and development salary summary
For time series, everything you can do.
Public number: AI snail car
Stay humble, stay disciplined, stay progressive
Send [Snail] to get a copy of "Hands-on AI Project" (AI Snail Car)
Send [1222] to get a good leetcode brushing note
Send [AI Four Classics] to get four classic AI e-books