1、安装NuGet包
1、Install-Package NLog.Web.AspNetCore
2、Install-Package NLog
在csproj中编辑:
<PackageReference Include="NLog" Version="4.5.3" />
<PackageReference Include="NLog.Web.AspNetCore" Version="4.5.3" />
2、创建一个nlog.config文件。

<?xml version="1.0" encoding="utf-8" ?> <nlog xmlns="http://www.nlog-project.org/schemas/NLog.xsd" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" autoReload="true" throwConfigExceptions="true" internalLogLevel="info" internalLogFile="D:\temp\internal-nlog.txt"> <!-- 要写的目标--> <targets> <!--将日志写入文件 --> <target xsi:type="File" name="allfile" fileName="D:\temp\nlog-all-${shortdate}.log" layout="${longdate}|${event-properties:item=EventId_Id:whenEmpty=0}|${uppercase:${level}}|${logger}|${message} ${exception:format=tostring}" /> <!-- 启用asp.net核心布局渲染器 --> <target xsi:type="File" name="ownFile-web" fileName="D:\temp\nlog-own-${shortdate}.log" layout="${longdate}|${event-properties:item=EventId_Id:whenEmpty=0}|${uppercase:${level}}|${logger}|${message} ${exception:format=tostring}|url: ${aspnet-request-url}|action: ${aspnet-mvc-action}|${callsite}" /> </targets> <!-- 从记录器名称映射到目标的规则 --> <rules> <!--所有的记录,包括从微软--> <logger name="*" minlevel="Trace" writeTo="allfile" /> <!--跳过非关键微软日志,因此只记录自己的日志--> <logger name="Microsoft.*" maxlevel="Info" final="true" /> <!-- BlackHole --> <logger name="*" minlevel="Trace" writeTo="ownFile-web" /> </rules> </nlog>
布局渲染器使用指南
- $ {aspnet-MVC-Action} - ASP.NET MVC动作名称
- $ {aspnet-MVC-Controller} - ASP.NET MVC控制器名称
- $ {aspnet-Application} - ASP.NET应用程序变量。
- $ {aspnet-Item} - ASP.NET
HttpContext
项目变量。 - $ {aspnet-Request} - ASP.NET请求变量。
- $ {aspnet-Request-Cookie} - ASP.NET请求cookie内容。
- $ {aspnet-Request-Host} - ASP.NET请求主机。
- $ {aspnet-Request-Method} - ASP.NET请求方法(GET,POST等)。
- $ {aspnet-Request-QueryString} - ASP.NET请求查询字符串。
- $ {aspnet-Request-Referrer} - ASP.NET请求引用者。
- $ {aspnet-Request-UserAgent} - ASP.NET请求useragent。
- $ {aspnet-Request-Url} - ASP.NET请求URL。
- $ {aspnet-Session} - ASP.NET Session变量。
- $ {aspnet-SessionId} - ASP.NET会话ID变量。
- $ {aspnet-TraceIdentifier} - ASP.NET跟踪标识符
- $ {aspnet-UserAuthType} - ASP.NET用户身份验证。
- $ {aspnet-UserIdentity} - ASP.NET用户变量。
- $ {iis-site-name} - IIS站点名称。
3、在csproj
手动编辑文件并添加
<ItemGroup> <Content Update="nlog.config" CopyToOutputDirectory="Always" /> </ItemGroup>
4、更新program.cs

public static void Main(string[] args) { // NLog:首先设置记录器以捕获所有错误 var logger = NLog.Web.NLogBuilder.ConfigureNLog("nlog.config").GetCurrentClassLogger(); try { logger.Debug("init main"); BuildWebHost(args).Run(); } catch (Exception exception) { // NLog:catch安装错误 logger.Error(exception, "Stopped program because of exception"); throw; } finally { //确保在退出应用程序之前刷新并停止内部定时器/线程(避免Linux上的分段错误) NLog.LogManager.Shutdown(); } } public static IWebHost BuildWebHost(string[] args) => WebHost.CreateDefaultBuilder(args) .UseStartup<Startup>() .ConfigureLogging(logging => { logging.ClearProviders(); logging.SetMinimumLevel(Microsoft.Extensions.Logging.LogLevel.Trace); }) .UseNLog() // NLog:setup NLog用于依赖注入 .Build();
5、配置appsettings.json

{ "Logging": { "IncludeScopes": false, "LogLevel": { "Default": "Warning", "Microsoft": "Information" } } }
6、写日志

private readonly ILogger _logger; public HomeController(ILogger<HomeController> logger) { _logger = logger; _logger.LogDebug(1, "NLog injected into HomeController"); } public IActionResult Index() { _logger.LogInformation("Hello, this is the index!"); return View(); }
7、输出示例
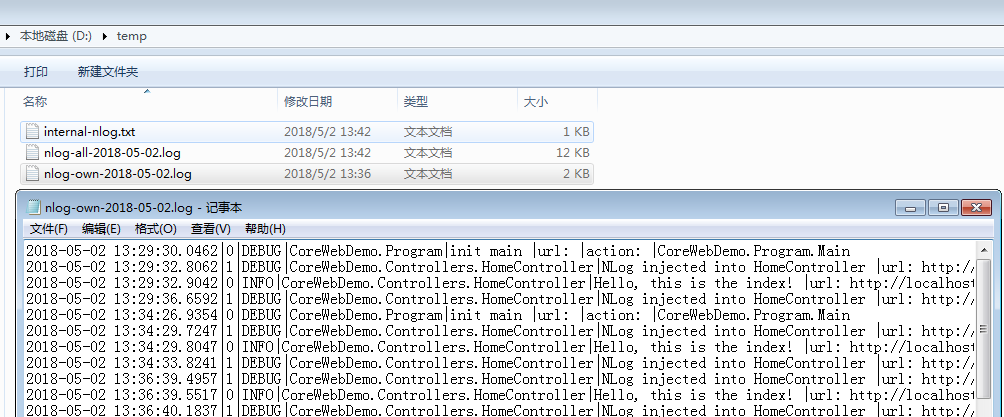