一、先创建 .net core Web 应用程序
二、程序包控制台输入以下命令行以安装 Nuget 包:
install-package Nlog
install-package Nlog.Web.AspNetCore
三、添加配置文件: nlog.config , 注意,右键属性中选择 “复制到目录”-》“如果较新则复制”
<?xml version="1.0" encoding="utf-8" ?>
<nlog xmlns="http://www.nlog-project.org/schemas/NLog.xsd"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
autoReload="true"
internalLogLevel="Warn"
internalLogFile="internal-nlog.txt">
<!--define various log targets-->
<targets>
<!--write logs to file-->
<!--<target xsi:type="File" name="allfile" fileName="nlog-all-${shortdate}.log"
layout="${longdate}|${logger}|${uppercase:${level}}|${message} ${exception}" />-->
<target xsi:type="File" name="ownFile-web" fileName="LogFiles/${shortdate}.log"
layout="${longdate}|${logger}|${uppercase:${level}}|${message} ${exception}" />
<!--<target xsi:type="Null" name="blackhole" />-->
</targets>
<rules>
<!--All logs, including from Microsoft-->
<!-- 记录微软所有日志,一般不开 -->
<!--<logger name="*" minlevel="Trace" writeTo="allfile" />-->
<!--Skip Microsoft logs and so log only own logs-->
<!--<logger name="Microsoft.*" minlevel="Fatal" writeTo="blackhole" final="true" />-->
<logger name="*" minlevel="Critical" writeTo="ownFile-web" />
</rules>
</nlog>
四、修改 Startup.cs , 其实除了引用之外, 也就是加两行代码而已:
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Logging;
using NLog.Extensions.Logging;
using NLog.Web;
namespace WebApplication1
{
public class Startup
{
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
public IConfiguration Configuration { get; }
// This method gets called by the runtime. Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services)
{
services.Configure<CookiePolicyOptions>(options =>
{
// This lambda determines whether user consent for non-essential cookies is needed for a given request.
options.CheckConsentNeeded = context => true;
options.MinimumSameSitePolicy = SameSiteMode.None;
});
services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IHostingEnvironment env, ILoggerFactory loggerFactory)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseCookiePolicy();
//使用NLog作为日志记录工具
loggerFactory.AddNLog();
//引入Nlog配置文件
env.ConfigureNLog("nlog.config");
app.UseMvc(routes =>
{
routes.MapRoute(
name: "default",
template: "{controller=Home}/{action=Index}/{id?}");
});
}
}
}
五、修改 Controller, 输出日志:
private ILogger<HomeController> logger;
public HomeController(ILogger<HomeController> _logger)
{
logger = _logger;
}
public IActionResult Index()
{
logger.LogInformation("普通信息日志-----------");
logger.LogDebug("调试日志-----------");
logger.LogError("错误日志-----------");
logger.LogCritical("异常日志-----------");
logger.LogWarning("警告日志-----------");
logger.LogTrace("跟踪日志-----------");
logger.Log(LogLevel.Warning, "Log日志------------------");
return View();
}
日志级别:Trace -》Debug-》 Information -》Warning-》 Error-》 Critical
日志级别由小到大, Trace 就包含了所有日志。
如果想修改日志的输出级别,应该在 nlog.config 中修改 minlevel="Trace"
试了一下, Critical 与 Fatal 的输出是一致的。
使用说明: https://github.com/NLog/NLog.Web/wiki/Getting-started-with-ASP.NET-Core-2
详细的配置参考: https://github.com/NLog/NLog/wiki/Configuration-file
中文配置文章: http://www.cnblogs.com/fuchongjundream/p/3936431.html
扫描二维码关注公众号,回复:
2525428 查看本文章
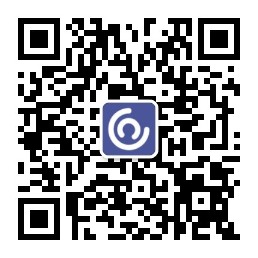