1、概念
当一个二维数组中大部分元素为0或则为同一个值时,可以使用稀疏数组保存这个二维数组
2、处理方式
1)稀疏数据的第一行分别存二维数组的几行几列以及二维数据中不为0的元素个数
2)将二维数据的行列几元素值存放到稀疏数组中
3、示例
原数组如下:
转换为稀疏数组如下:
4、java代码实现如下
扫描二维码关注公众号,回复:
9663178 查看本文章
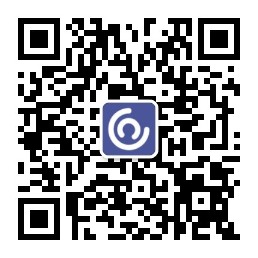
public class SparseArray {
public static void main(String[] args) {
System.out.println("-------------------申明一个二维数据------------------------");
//1.申明一个普通的二维数组代表围棋棋盘 数组的值为0时表示空白,为1时表示白子,为2时表示黑子
int[][] chessArray = new int[11][11];
chessArray[1][2] = 1;
chessArray[2][3] = 2;
chessArray[3][4] = 2;
//2.遍历普通二维数组,并在控制台打印出来
for (int[] row :chessArray) {
for (int data : row) {
System.out.printf("%d\t", data);
}
System.out.println();
}
System.out.println("------------------二维数据转稀疏数据-------------------------");
//将普通二维数组转换为稀疏数组
//1.遍历普通二维数组,获取不为0的数值个数
int sum = 0;
for (int[] row :chessArray) {
for (int data : row) {
if(data != 0){
sum ++;
}
}
}
//2.声明一个稀疏数组,并且将第一行赋值
int[][] sparseArray = new int[sum + 1][3];
sparseArray[0][0] = 11;
sparseArray[0][1] = 11;
sparseArray[0][2] = sum;
//3.给稀疏数组其余行赋值
int count = 0;
for (int i = 0; i < 11; i++) {
for (int j = 0; j < 11; j++) {
if(chessArray[i][j] != 0){
count ++;
sparseArray[count][0] = i;
sparseArray[count][1] = j;
sparseArray[count][2] = chessArray[i][j];
}
}
}
//3.遍历稀疏数组
for (int i = 0; i < sparseArray.length; i++) {
System.out.printf("%d\t%d\t%d\t", sparseArray[i][0], sparseArray[i][1], sparseArray[i][2]);
System.out.println();
}
String fileName = "D:\\MyProject\\DataStructures\\src\\resource\\map.data";
//4.保存稀疏数组到文件中
writeSpareToFile(sparseArray, fileName);
System.out.println("------------------稀疏数据转二维数据-------------------------");
//将稀疏数组转为普通二维数组
//1.从文件中获取稀疏数组数据
int[][] sparseArray1 = readFileToSpare(fileName);
//2.通过稀疏数据的第一行声明一个普通二维数据
int[][] chessArray1 = new int[sparseArray1[0][0]][sparseArray1[0][1]];
//3.遍历稀疏数据赋值给普通二维数据
for (int i = 1; i < sparseArray1.length; i++) {
chessArray1[sparseArray1[i][0]][sparseArray1[i][1]] = sparseArray1[i][2];
}
//4.遍历普通二维数组
for (int[] array : chessArray1) {
for (int data : array) {
System.out.printf("%d\t", data);
}
System.out.println();
}
}
/**
* @description: 将稀疏数据写入文件中
* @param sparseArray
* @param fileName
* @return: void
* @author: sukang
* @date: 2019/12/25 17:10
*/
public static void writeSpareToFile(int[][] sparseArray, String fileName){
File file = new File(fileName);
FileWriter fw = null;
BufferedWriter bw = null;
try {
fw = new FileWriter(file);
bw = new BufferedWriter(fw);
for (int i = 0; i < sparseArray.length; i++) {
StringBuffer sb = new StringBuffer();
for (int j = 0; j < sparseArray[i].length; j++) {
sb.append(sparseArray[i][j] + " ");
}
sb.append("\r\n");
bw.write(sb.toString());
bw.flush();
}
} catch ( IOException e ) {
e.printStackTrace();
} finally {
try {
if(bw != null){
bw.close();
}
if(fw != null){
fw.close();
}
} catch ( IOException e ) {
e.printStackTrace();
}
}
}
/**
* @description: 从文件中读取稀疏数组
* @param fileName
* @return: int[][]
* @author: sukang
* @date: 2019/12/26 14:29
*/
public static int[][] readFileToSpare(String fileName){
int[][] spare = {};
File file = new File(fileName);
FileReader fr = null;
BufferedReader br = null;
try {
List<String> strs = new ArrayList<>();
fr = new FileReader(file);
br = new BufferedReader(fr);
String line = null;
while ((line = br.readLine()) != null){
strs.add(line);
}
spare = new int[strs.size()][strs.get(0).split(" ").length];
for (int i = 0; i < strs.size(); i++) {
String[] str = strs.get(i).split(" ");
for (int j = 0; j < str.length; j++) {
spare[i][j] = Integer.parseInt(str[j]);
}
}
} catch ( FileNotFoundException e ) {
e.printStackTrace();
} catch ( IOException e ) {
e.printStackTrace();
}finally {
try {
if(br != null){
br.close();
}
if(fr != null){
fr.close();
}
} catch ( IOException e ) {
e.printStackTrace();
}
return spare;
}
}
}