写在开头
本文将带你深入理解之Promise篇
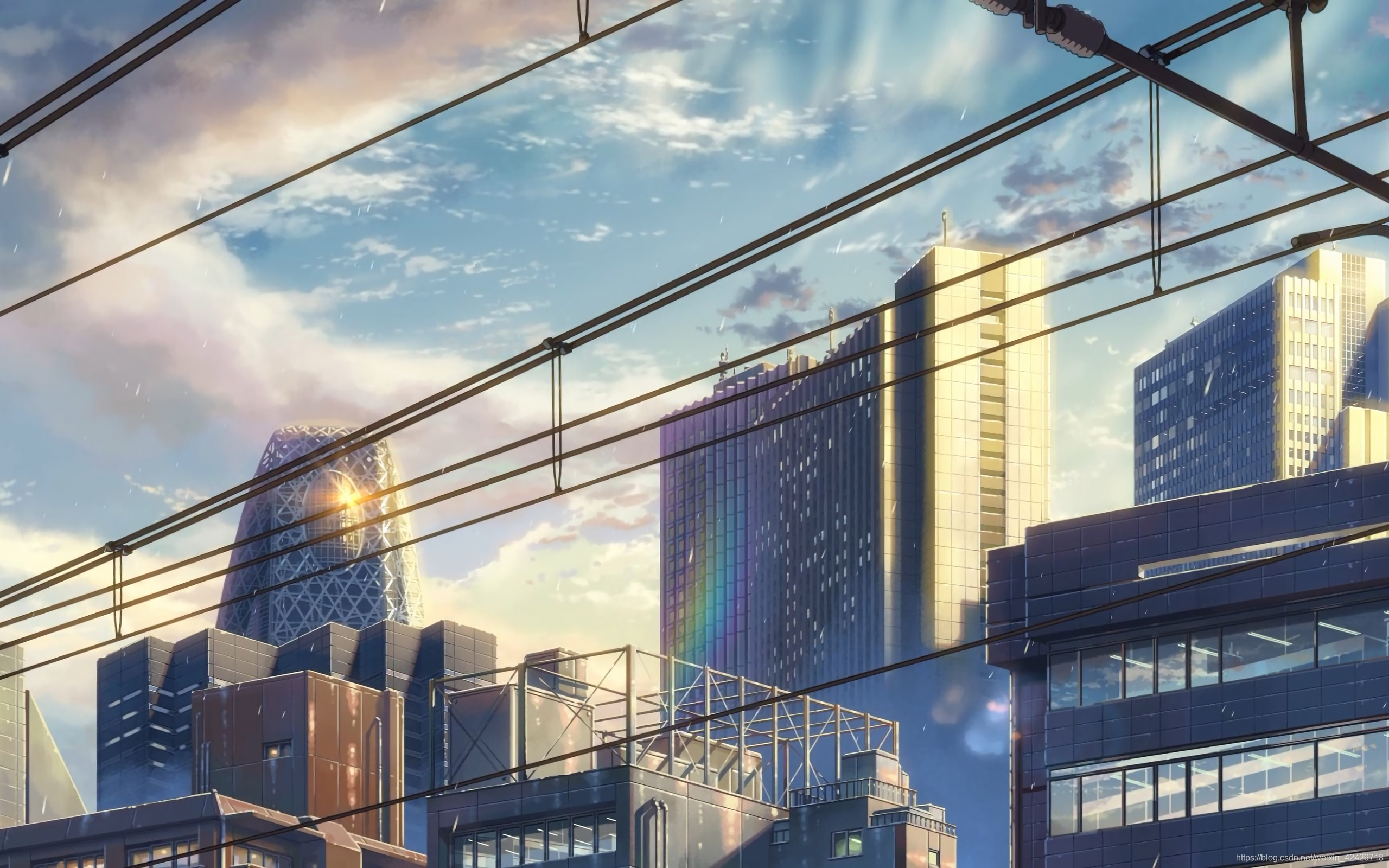
后续的文章都会与前端有关,欢迎各位同路途的人一起交流学习,3月份又是努力的开头,加油!
如果想更多了解ES6,请参考之前写过的一些文章:
ES6 一文弄懂 var let const 三剑客区别 吊打面试题
ES6 深入理解 …拓展运算符 合并数组及demo选项卡实例
ES6 深入理解之字符串篇 保姆级 教你用js写选项卡demo
ES6 深入理解之number篇 保姆级 教你用js写选项卡demo
ES6 深入理解之方法篇 保姆级 教你用js写选项卡demo
Pending、Resolved(决定)、Rejected(拒绝)
then
new Promise(function(Resolved,reject){
Resolved();
reject();
}).then(function(){
alert('成功')
},function(){
alert('失败')
})
Promise 是个对象
类似 一个事情
事情 就会 有 成功 或者 失败
只要触发一个 这个函数就完成了自己任务
类似于 jquery 中 one 只执行一次
then catch
new Promise(function(Resolved,reject){
Resolved('hehehehe');
//reject();
}).then(function(x){
console.log(x)
}).catch(function(){
console.log('2313213')
})
封装
function showPromise(res){
return new Promise((resolve,reject)=>{
resolve(res)
}).then((x)=>{console.log(x)},()=>{
})
}
showPromise('666');
showPromise('123');
showPromise('哈哈哈');
promise的无限回调
promise 厉害之处就是能无限的回调
new Promise(function(Resolved,reject){
Resolved('hehehehe');
}).then(function(x){
alert(x);
return 'ashkdajs';
}).then(function(x){
alert(x);
return '101010'
}).then(function(x){
alert(x);
})
用promise写一个简易显示隐藏卡片
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style type="text/css">
#{
margin: 0;
padding: 0;
list-style: none;
}
div{
width: 200px;
height: 200px;
background: #CCCCCC;
display: block;
}
</style>
</head>
<body>
<input type="button" id="ipt" value="显示/隐藏" />
<div id="div1"></div>
<script type="text/javascript">
let cc=0;
ipt.onclick = x =>{
cc++;
new Promise((success,error)=>{
cc%2==1?success():error();
}).then(()=>{
div1.style.display="block";
}).catch(()=>{
div1.style.display="none";
})
}
</script>
</body>
</html>
throw 抛出异常
抛出 异常throw ‘出大事了 下面就别执行了’
类似的有:
console.error();
console.warn();
throw '抛出异常'
race 竞速方法
race ->只看第一个promise 成功就成功 ,失败就失败
Promise.race([new Promise((succ,error)=>{
//succ('12312');
setTimeout(error,1000,'1');
}),new Promise((succ,error)=>{
setTimeout(succ,1200,'2');
}),new Promise((succ,error)=>{
setTimeout(succ,1400,'3');
})]).then((x)=>{
console.log(x+'suss');
},(x)=>{
console.log(x+'error')
})
all 规整方法
all ->要不然就是全胜 要不然就是 失败
Promise.all([new Promise((succ,error)=>{
setTimeout(succ,1000);
}),new Promise((succ,error)=>{
setTimeout(succ,2000);
}),new Promise((succ,error)=>{
setTimeout(error,1000);
})]).then(()=>{
console.log('成功!')
}).catch(()=>{
console.log('失败')
})
reject
Promise.reject(['1','2']).then((f,x)=>{
//console.log(x)
}).catch((a)=>{
console.log(a)
})
自动选项卡 demo
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style type="text/css">
input.active{
background: red;
}
.outNode>div{width: 200px;height: 200px;background: #ccc;display: none;}
.outNode>div:first-of-type{
display: block;
}
</style>
</head>
<body>
<script type="text/javascript">
const $=obj=>[...document.querySelectorAll(obj)];
const jsonDate={
'vlaueName':['小黑','小白','小红'],
'innnerHtml':[
'变黑了','变瘦了','变高了'
]
};
let Index = 0;
const createNode=json=>`<div class=outNode>
${json.vlaueName.map((x)=>`<input type=button value=${x}>`).join('')}
${json.innnerHtml.map((x)=>`
<div>${x}</div>
`).join('')}
</div>`;
document.body.innerHTML = createNode(jsonDate);
let allInput = new Set($('input'));
let allDiv = new Set($('.outNode>div'));
[...allInput][0].className = 'active';
[...allInput].forEach((x,y)=>{
x['onclick']=()=>{
[...allDiv].forEach(f=>f.style.display='none');
[...allDiv][y].style.display = 'block';
}
});
setInterval(()=>{
Index++;
(Index == [...allInput].length) &&(Index=0);
[...allInput].forEach(x=>x.className='');
[...allInput][Index].className = 'active';
[...allDiv].forEach(x=>x.style.display='none');
[...allDiv][Index].style.display = 'block';
},1000);
</script>
</body>
</html>
总结
Pending、Resolved(决定)、Rejected(拒绝)
catch
race 竞速方法
all 规整方法
学如逆水行舟,不进则退