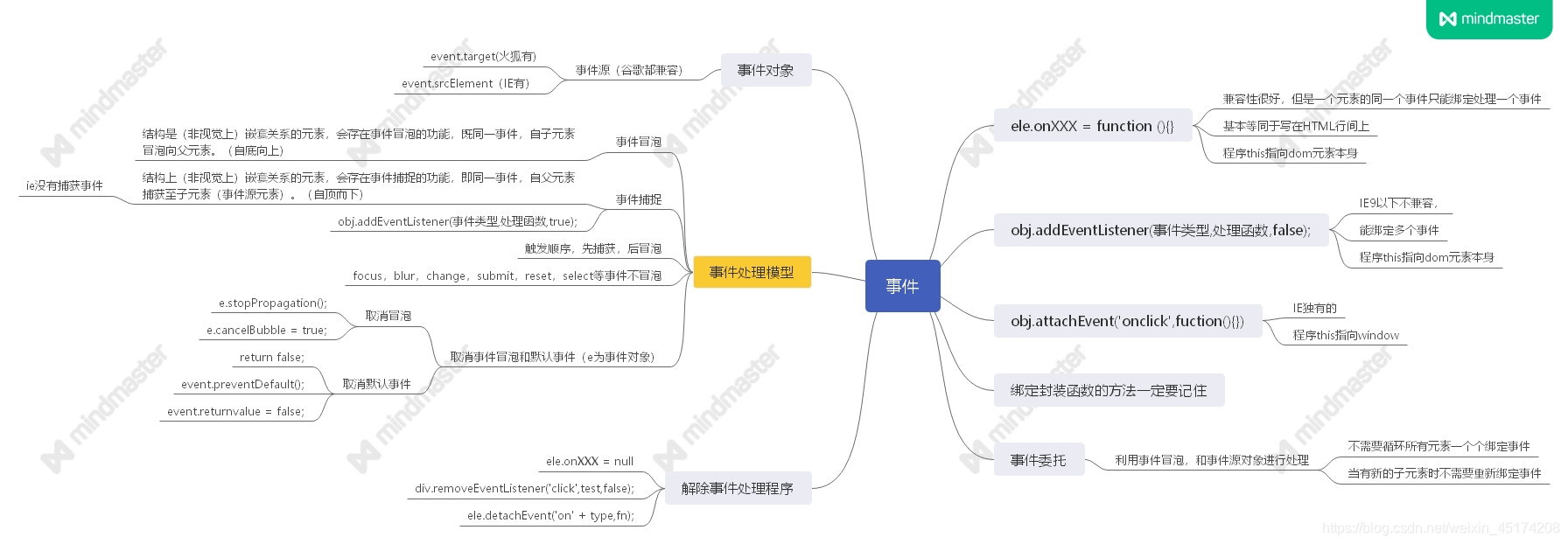
<!DOCTYPE html>
<html>
<head>
<title></title>
</head>
<body>
<!--
<div style="width: 100px;height: 100px;background-color: red;" onclick="console.log('a') "></div> -->
<div style="width: 100px; height: 100px; background-color: red;"></div>
<ul>
<li>1</li>
<li>2</li>
<li>3</li>
</ul>
<script type="text/javascript">
var div = document.getElementsByTagName('div')[0];
// div.onclick = function () {
// this.style.backgroundColor = 'green';
// console.log('a');
// this.onclick = null;
// }
// div.onclick = null;
div.addEventListener('click',test,false);
div.removeEventListener('click',test,false);
function test() {
console.log('a');
}
// div.addEventListener('click',function () {
// console.log(this);//指向dom元素本身
// },false);
//IE独用的
// div.attachEvent('onclick',function () {
// console.log('a');
// })
var li = document.getElementsByTagName('li');
var len = li.length;
for(var i = 0; i < len; i ++){
//事件出现在循环里面一定要考虑闭包
(function (i) {
li[i].addEventListener('click',function () {
console.log(i);
},false);
}(i))
}
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
.wrapper{
width: 300px;
height: 300px;
background-color: red;
}
.content{
width: 200px;
height: 200px;
background-color: green;
}
.box{
width: 100px;
height: 100px;
background-color: aqua;
}
</style>
</head>
<body>
<!-- 取消默认事件 -->
<a href="javascript:void(false)">4242424</a>
<div class="wrapper">
<div class="content">
<div class="box"></div>
</div>
</div>
<script>
var wrapper = document.getElementsByClassName('wrapper')[0];
var content = document.getElementsByClassName('content')[0];
var box = document.getElementsByClassName('box')[0];
//事件冒泡 结构上 的 由子元素到父元素上的
//事件捕获 从父元素到子元素false --> true
//先捕获后冒泡
// wrapper.addEventListener('click', function () {
// console.log('wrapper222')
// }, false);
// content.addEventListener('click', function () {
// console.log('content222')
// }, false);
//事件执行的顺序谁先绑定谁先执行
// box.addEventListener('click', function () {
// console.log('box222')
// }, false);
// wrapper.addEventListener('click', function () {
// console.log('wrapper')
// }, true);
// content.addEventListener('click', function () {
// console.log('content')
// }, true);
// box.addEventListener('click', function () {
// console.log('box')
// }, true);
//事件源对象
box.onclick = function (e) {
var event = e || window.event;
var target = event.target || event.srcElement;
console.log(target);
console.log(event);
}
document.onclick = function () {
console.log('你闲的啊');
}
wrapper.onclick = function (e) {
this.style.background = 'green';
// 取消冒泡事件的两种方式 e为事件对象
// e.stopPropagation();
e.cancelBubble = true;
}
//取消默认事件
document.oncontextmenu = function (e) {
console.log('a');
// e.returnValue = false;
// e.preventDefault();
// return false;
}
//取消a标签的默认事件
var a = document.getElementsByTagName('a')[0];
a.onclick = function () {
// return false;
}
</script>
</body>
</html>