在终端某个群组添加机器人之后,可以获取到 webhook 地址,然后我们按以下说明构造 post data,向这个地址发起 HTTP POST 请求,就可以实现给该群组发送消息。
假设我们在群组里添加机器人后获取的 webhook 是:https://qyapi.weixin.qq.com/cgi-bin/webhook/send?key=69e3bed5-a20e-45be-9999-e30c0398c16c(这个需要自己在群组里创建企业微信机器人之后,从 webhook 中摘取并替换)。
1. 文本类型消息
1.1. 通过企业微信机器人发送文本类型消息
import requests
import json
class WXWork_SMS :
# 文本类型消息
def send_msg_txt(self) :
headers = {"Content-Type" : "text/plain"}
send_url = "https://qyapi.weixin.qq.com/cgi-bin/webhook/send?key=69e3bed5-a20e-45be-9999-e30c0398c16c"
send_data = {
"msgtype": "text", # 消息类型,此时固定为text
"text": {
"content": "上海今日天气:32度,大部分多云,降雨概率:10%", # 文本内容,最长不超过2048个字节,必须是utf8编码
"mentioned_list":["@all"], # userid的列表,提醒群中的指定成员(@某个成员),@all表示提醒所有人,如果开发者获取不到userid,可以使用mentioned_mobile_list
"mentioned_mobile_list":["@all"] # 手机号列表,提醒手机号对应的群成员(@某个成员),@all表示提醒所有人
}
}
res = requests.post(url = send_url, headers = headers, json = send_data)
print(res.text)
if __name__ == '__main__' :
sms = WXWork_SMS()
sms.send_msg_txt()
1.2. 参数说明
参数 | 必须 | 说明 |
---|---|---|
msgtype | true | 消息类型,此时固定为text |
content | true | 文本内容,最长不超过2048个字节,必须是utf8编码 |
mentioned_list | false | userid的列表,提醒群中的指定成员(@某个成员),@all表示提醒所有人,如果开发者获取不到userid,可以使用mentioned_mobile_list |
mentioned_mobile_list | false | 手机号列表,提醒手机号对应的群成员(@某个成员),@all表示提醒所有人 |
1.3. 发送结果
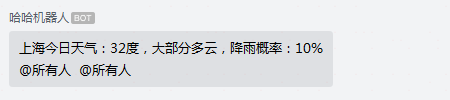
2. Markdown类型消息
2.1. 通过企业微信机器人发送 Markdown 类型消息
import requests
import json
class WXWork_SMS :
# Markdown类型消息
def send_msg_markdown(self) :
headers = {"Content-Type" : "text/plain"}
send_url = "https://qyapi.weixin.qq.com/cgi-bin/webhook/send?key=69e3bed5-a20e-45be-9999-e30c0398c16c"
send_data = {
"msgtype": "markdown", # 消息类型,此时固定为markdown
"markdown": {
"content": "# **提醒!实时新增用户反馈**<font color=\"warning\">**123例**</font>\n" + # 标题 (支持1至6级标题,注意#与文字中间要有空格)
"#### **请相关同事注意,及时跟进!**\n" + # 加粗:**需要加粗的字**
"> 类型:<font color=\"info\">用户反馈</font> \n" + # 引用:> 需要引用的文字
"> 普通用户反馈:<font color=\"warning\">117例</font> \n" + # 字体颜色(只支持3种内置颜色)
"> VIP用户反馈:<font color=\"warning\">6例</font>" # 绿色:info、灰色:comment、橙红:warning
}
}
res = requests.post(url = send_url, headers = headers, json = send_data)
print(res.text)
if __name__ == '__main__' :
sms = WXWork_SMS()
# Markdown类型消息
sms.send_msg_markdown()
2.2. 参数说明
参数 | 必须 | 说明 |
---|---|---|
msgtype | true | 消息类型,此时固定为markdown |
content | true | markdown内容,最长不超过4096个字节,必须是utf8编码 |
目前支持的 markdown 语法是如下的子集:
2.2.1.标题 (支持1至6级标题,注意#与文字中间要有空格)
# 标题一
## 标题二
### 标题三
#### 标题四
##### 标题五
###### 标题六
2.2.2.加粗
**bold**
2.2.3.链接
[这是一个链接](http://work.weixin.qq.com/api/doc)
2.2.4.行内代码段(暂不支持跨行)
`code`
2.2.5.引用
> 引用文字
2.2.6.字体颜色(只支持3种内置颜色)
<font color="info">绿色</font>
<font color="comment">灰色</font>
<font color="warning">橙红色</font>
2.3. 发送结果
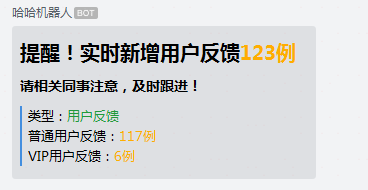
3. 图文类型消息
3.1. 通过企业微信机器人发送图文类型消息
import requests
import json
class WXWork_SMS :
# 图文类型消息
def send_msg_txt_img(self) :
headers = {"Content-Type" : "text/plain"}
send_url = "https://qyapi.weixin.qq.com/cgi-bin/webhook/send?key=69e3bed5-a20e-45be-9999-e30c0398c16c"
send_data = {
"msgtype": "news", # 消息类型,此时固定为news
"news": {
"articles" : [ # 图文消息,一个图文消息支持1到8条图文
{
"title" : "中秋节礼品领取", # 标题,不超过128个字节,超过会自动截断
"description" : "今年中秋节公司有豪礼相送", # 描述,不超过512个字节,超过会自动截断
"url" : "www.baidu.com", # 点击后跳转的链接。
"picurl" : "http://res.mail.qq.com/node/ww/wwopenmng/images/independent/doc/test_pic_msg1.png" # 图文消息的图片链接,支持JPG、PNG格式,较好的效果为大图 1068*455,小图150*150。
},
{
"title" : "我的CSDN - 魏风物语", # 标题,不超过128个字节,超过会自动截断
"description" : "坚持每天写一点点", # 描述,不超过512个字节,超过会自动截断
"url" : "https://blog.csdn.net/itanping", # 点击后跳转的链接。
"picurl" : "http://res.mail.qq.com/node/ww/wwopenmng/images/independent/doc/test_pic_msg1.png" # 图文消息的图片链接,支持JPG、PNG格式,较好的效果为大图 1068*455,小图150*150。
}
]
}
}
res = requests.post(url = send_url, headers = headers, json = send_data)
print(res.text)
if __name__ == '__main__' :
sms = WXWork_SMS()
# 图文类型消息
sms.send_msg_txt_img()
3.2. 参数说明
参数 | 必须 | 说明 |
---|---|---|
msgtype | true | 消息类型,此时固定为news |
articles | 是 | 图文消息,一个图文消息支持1到8条图文 |
title | 是 | 标题,不超过128个字节,超过会自动截断 |
description | 否 | 描述,不超过512个字节,超过会自动截断 |
url | 是 | 点击后跳转的链接。 |
picurl | 否 | 图文消息的图片链接,支持JPG、PNG格式,较好的效果为大图 1068*455,小图150*150。 |
3.3. 发送结果
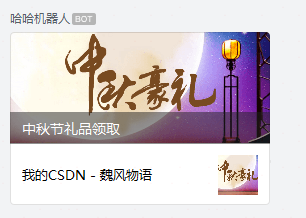
注意:
1.消息发送频率限制:每个机器人发送的消息不能超过20条/分钟
2.上述代码中的 send url 的 key 需要自己创建企业微信机器人之后,从 webhook 中摘取并替换
本例代码: https://download.csdn.net/download/itanping/11643509