1.创建一个maven项目
2.导入相应的jar包
<repositories>
<repository>
<id>cloudera</id>
<url>https://repository.cloudera.com/artifactory/cloudera-repos/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>org.apache.hadoop</groupId>
<artifactId>hadoop-client</artifactId>
<version>2.6.0-mr1-cdh5.14.0</version>
</dependency>
<dependency>
<groupId>org.apache.hbase</groupId>
<artifactId>hbase-client</artifactId>
<version>1.2.0-cdh5.14.0</version>
</dependency>
<dependency>
<groupId>org.apache.hbase</groupId>
<artifactId>hbase-server</artifactId>
<version>1.2.0-cdh5.14.0</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>6.14.3</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.0</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
<encoding>UTF-8</encoding>
<!-- <verbal>true</verbal>-->
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-shade-plugin</artifactId>
<version>2.2</version>
<executions>
<execution>
<phase>package</phase>
<goals>
<goal>shade</goal>
</goals>
<configuration>
<filters>
<filter>
<artifact>*:*</artifact>
<excludes>
<exclude>META-INF/*.SF</exclude>
<exclude>META-INF/*.DSA</exclude>
<exclude>META-INF/*/RSA</exclude>
</excludes>
</filter>
</filters>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
3.编写代码
1.判断表是否存在
/** * 判断表是否存在 * * @param name * @return * @throws IOException */ public static boolean tableExists(String name) throws IOException { // 配置hbase的相关配置 HBaseConfiguration configuration = new HBaseConfiguration(); configuration.set("hbase.zookeeper.property.clientPort", "2181"); configuration.set("hbase.zookeeper.quorum", "node01"); // 获取连接对象 Connection connection = ConnectionFactory.createConnection(configuration); // 获取hbase 的超级管理员 Admin admin = connection.getAdmin(); boolean b = admin.tableExists(TableName.valueOf(name)); // 关闭资源 admin.close(); // 结果反回 return b; }
2.准备工作 相关的配置抽出来当作变量
static Admin admin = null; static Connection connection = null; static { try { // 配置hbase的相关配置 HBaseConfiguration configuration = new HBaseConfiguration(); configuration.set("hbase.zookeeper.property.clientPort", "2181"); configuration.set("hbase.zookeeper.quorum", "node01"); connection = ConnectionFactory.createConnection(configuration); admin = connection.getAdmin(); } catch (IOException e) { e.printStackTrace(); } }
3.创建表
/** * 创建表 * * @param tableName 表名 * @param cfs 列族 * @throws IOException */ public static void createTable(String tableName, String... cfs) throws IOException { // 判断表是否存在 if (tableExists(tableName)) { System.out.println("你创建的" + tableName + "已经存在了"); return; } // 创建表描述器 HTableDescriptor tableDescriptor = new HTableDescriptor(TableName.valueOf(tableName)); for (String cf : cfs) { // 创建列祖描述器 HColumnDescriptor hColumnDescriptor = new HColumnDescriptor(cf); tableDescriptor.addFamily(hColumnDescriptor); } // 创建表 admin.createTable(tableDescriptor); // 关闭资源 admin.close(); connection.close(); System.out.println("创建成功"); }
4.删除表
/** * 删除表 * * @param tableName 表名 * @throws IOException */ public static void deleteName(String tableName) throws IOException { if (!tableExists(tableName)) { System.out.println("你删除的表不存在"); return; } // 设置表不可用 admin.disableTable(TableName.valueOf(tableName)); // 删除表 admin.deleteTable(TableName.valueOf(tableName)); System.out.println("删除成功"); // 关闭资源 admin.close(); connection.close(); }
4.增 改
** * 增 改 * * @param tableName 表名 * @param rowKey rk * @param cf 列族 * @param cn 族名 * @param value 值 */ public static void putData(String tableName, String rowKey, String cf, String cn, String value) throws IOException { // 获取table 对象 Table table = connection.getTable(TableName.valueOf(tableName)); // 获取put 对象 Put put = new Put(Bytes.toBytes(rowKey)); // 添加数据 put.addColumn(Bytes.toBytes(cf), Bytes.toBytes(cn), Bytes.toBytes(value)); // 执行添加操作 table.put(put); System.out.println("添加完成"); // 关闭资源 table.close(); admin.close(); connection.close(); }
5.删除
/** * 删除 * * @param tableName 删除表名 * @param rowKey 删除rok * @param cf 删除 列族 * @param cn 删除列 * @throws IOException */ public static void delete(String tableName, String rowKey, String cf, String cn) throws IOException { // 获取table 对象 Table table = connection.getTable(TableName.valueOf(tableName)); // 获取delete对象 Delete delete = new Delete(Bytes.toBytes(rowKey)); /* // 删除列 delete.addColumns(Bytes.toBytes(cf),Bytes.toBytes(cn)); // 删除 列祖 delete.addFamily(Bytes.toBytes(cf));*/ // 删除操作 table.delete(delete); // 关闭资源 table.close(); admin.close(); connection.close(); }
6.查询所有
/** * 查询所有 * @param tableName 表名 * @throws IOException */ public static void scanTableAll(String tableName) throws IOException { // 获取table对象 Table table = connection.getTable(TableName.valueOf(tableName)); // 构建扫描器 Scan scan = new Scan(); ResultScanner results = table.getScanner(scan); // 遍历并打印 for (Result result : results) { Cell[] cells = result.rawCells(); for (Cell cell : cells) { byte[] cloneRow = CellUtil.cloneRow(cell); byte[] cloneFamily = CellUtil.cloneFamily(cell); byte[] cloneQualifier = CellUtil.cloneQualifier(cell); byte[] value = CellUtil.cloneValue(cell); System.out.print("RK: "+Bytes.toString(cloneRow)); System.out.print(",CF: "+Bytes.toString(cloneFamily)); System.out.print(",CN: "+Bytes.toString(cloneQualifier)); System.out.println(",VALUE: "+Bytes.toString(value)); } } // 关闭资源 table.close(); admin.close(); connection.close(); }
7.查询指定rowKey 或指定的列族或列
/** * 查询指定rowkey * @param tableName 表名 * @param rowKey * @param fs * @param cn * @throws IOException */ public static void getData(String tableName,String rowKey,String fs,String cn) throws IOException { // 获取去tables对象 Table table = connection.getTable(TableName.valueOf(tableName)); Get get = new Get(Bytes.toBytes(rowKey)); // 获取指定列族和列 get.addColumn(Bytes.toBytes(fs),Bytes.toBytes(cn)); Result result = table.get(get); Cell[] cells = result.rawCells(); // 遍历输出 for (Cell cell : cells) { byte[] cloneRow = CellUtil.cloneRow(cell); byte[] cloneFamily = CellUtil.cloneFamily(cell); byte[] cloneQualifier = CellUtil.cloneQualifier(cell); byte[] value = CellUtil.cloneValue(cell); System.out.print("RK: "+Bytes.toString(cloneRow)); System.out.print(",CF: "+Bytes.toString(cloneFamily)); System.out.print(",CN: "+Bytes.toString(cloneQualifier)); System.out.println(",VALUE: "+Bytes.toString(value)); } // 关闭资源 table.close(); admin.close(); connection.close(); }
扫描二维码关注公众号,回复:
9591757 查看本文章
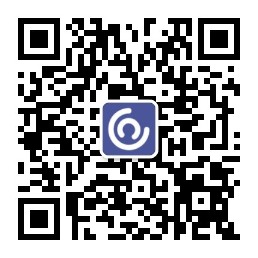