今天初步学习到了Fragment ,如果说activity是一堵墙, 那么它类似于一个便利贴,能将内容写在上面粘贴到任意一面墙上, 而不需要每一堵墙上都写满相同的内容 ,
下面我们来了解 怎样编写 Fragment 以及 动静态加载 传参 的代码
Fragment 之 静态传参
第一步:创建一个 FragmentA.java 文件, 编写如下代码
package com.lym.week12.fragment; import android.os.Bundle; import android.support.annotation.Nullable; import android.support.v4.app.Fragment; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import com.lym.week12.R; public class FragmentA extends Fragment{ //初始化布局和控件 @Nullable @Override public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) { /** * LayoutInflater 布局加载器 */ View view = inflater.inflate(R.layout.fragment_a,null); View textView = view.findViewById(R.id.text_view); return view; } }
第二步:在layout下编写上诉代码中需要用到的 fragment.fragment_a.xml 文件
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#ff0000" android:gravity="center"> <TextView android:id="@+id/text_view" android:textColor="#fff" android:textSize="30sp" android:text="学校" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </LinearLayout>
第三步:编写 activity_main.xml 文件
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity" android:orientation="vertical"> <fragment android:id="@+id/fragment_a" android:layout_width="match_parent" android:layout_height="100dp" android:name="com.lym.week12.fragment.FragmentA" tools:layout="@layout/fragment_a"/> </LinearLayout>
第四步:编写 MainActivity.java 文件
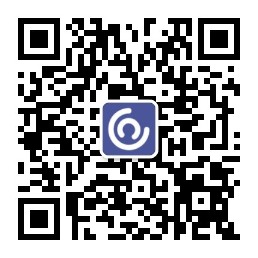
package com.lym.week12; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; public class ActivityWechat extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } }
Fragment 之 动态传参
第一步:编写一个 fragmentB.java 文件
package com.lym.week12.fragment; import android.os.Bundle; import android.support.annotation.Nullable; import android.support.v4.app.Fragment; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import com.lym.week12.R; public class FragmentB extends Fragment{ @Nullable @Override public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) { View view = inflater.inflate(R.layout.fragment_b, null); return view; } }
第二步:在layout中编写上述代码中用到的 fragment_b.xml 文件
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#542525" android:gravity="center"> <TextView android:id="@+id/text_view" android:textColor="#fff" android:textSize="30sp" android:text="病毒" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </LinearLayout>
第三步:编写 activity_main.xml 文件
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity" android:orientation="vertical"> <FrameLayout android:id="@+id/fragment_b" android:layout_width="match_parent" android:layout_height="100dp"/> </LinearLayout>
第四步:编写 MainActivity.java 文件
package com.lym.week12; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.widget.Button; import com.lym.week12.fragment.FragmentB; import com.lym.week12.fragment.FragmentC; public class MainActivity extends AppCompatActivity { private FragmentC fragmentC; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); loadFragmentB(); } /** * 1.动态加载FragmentB */ private void loadFragmentB() { //1.获取fragment管理器 android.support.v4.app.FragmentManager manager = getSupportFragmentManager(); //2.开启一个事务 android.support.v4.app.FragmentTransaction transaction = manager.beginTransaction(); FragmentB fragmentB = new FragmentB(); // 3.向容器中添加fragment transaction.add(R.id.fragment_b,fragmentB); // 4.提交事务 transaction.commit(); } }
此时 Fragment 的 动态传参 和 静态传参 已经编写完成,也可以发现两者 代码上只有些许的不同
但是 动态传参中 还有一些小小的缺陷,如上面动态传参代码中的数据也都是 初始化加载 得到的,
并不是我們自己传入其中的, 那么 我们 怎样 来实现 真正得 动态传参 呢?
话不多少,我们上代码
第一步:我们再次创建 一个 fragmentC.java 文件
package com.lym.week12.fragment; import android.os.Bundle; import android.support.annotation.Nullable; import android.support.v4.app.Fragment; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.TextView; import com.lym.week12.R; public class FragmentC extends Fragment{ private TextView textView; @Nullable @Override public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) { View view = inflater.inflate(R.layout.fragment_c, null); textView = view.findViewById(R.id.text_view); Bundle bundle = getArguments(); //获取 MainActivity.java 发过来的参数 if (bundle!=null){ //如果bundle不为空 String data1 = bundle.getString("data"); //把得到data里的值赋给字符串data1 textView.setText(data1); // 把data1传给fragment_c中TextView 的 text } return view; } public void sendData(String data){ textView.setText(data); } }
第二步,我们分别编写上述代码中 高亮部分 的两个文件 fragment_c.xml 与 MainActivity.java
fragment_c.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#f4b64c" android:gravity="center"> <TextView android:id="@+id/text_view" android:textColor="#fff" android:textSize="30sp" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </LinearLayout>
MainActivity.java
package com.lym.week12; import android.support.v4.app.FragmentManager; import android.support.v4.app.FragmentTransaction; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import com.lym.week12.fragment.FragmentB; import com.lym.week12.fragment.FragmentC; public class MainActivity extends AppCompatActivity { private FragmentC fragmentC; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); loadFragmentC(); Button button = findViewById(R.id.btn); button.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { fragmentC.sendData("打游戏"); } }); } private void loadFragmentC() { // 1.获取fragment管理器 FragmentManager manager = getSupportFragmentManager(); // 2.开启一个事务 FragmentTransaction transaction = manager.beginTransaction(); fragmentC = new FragmentC(); Bundle bundle = new Bundle(); // 传入参数 bundle.putString("data", "家(杨明飞)"); bundle.putInt("int", 1000); fragmentC.setArguments(bundle); // 3.向容器中添加fragment transaction.add(R.id.fragment_c, fragmentC); // 4.提交事务 transaction.commit(); } }
第三步:编写activity_main.xml文件
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity" android:orientation="vertical"> <FrameLayout android:id="@+id/fragment_c" android:layout_width="match_parent" android:layout_height="100dp" /> <Button android:id="@+id/btn" android:text="打游戏" android:layout_width="100dp" android:layout_height="100dp" /> </LinearLayout>
到这里 Fragment 简单的 静态传参 和 动态传参 就 写好了!
如有不对,多多指正!