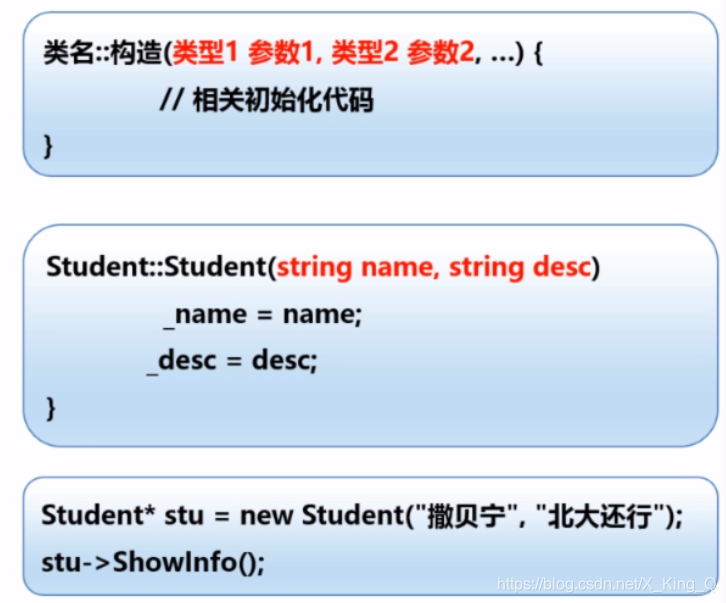
代码
Student.h
#ifndef STUDENT_H
#define STUDENT_H
#include <iostream>
using namespace std;
class Student
{
public:
//构造函数的重载与普通函数的重载规则相同
//重载时不关注返回值类型,只关注参数类型
//如果构造函数中,只有一个是带唯一的参数:Student student4 = 40;
Student();
Student(string,string);//带参构造
Student(int);
~Student();
void ShowInfo();
string GetName() {
return m_Name;
}
void SetName(string val) {
m_Name = val;
}
string Getdesc() {
return m_desc;
}
void Setdesc(string val) {
m_desc = val;
}
int Getage() {
return m_age;
}
void Setage(int val) {
if(val<0){
m_age = 18;
}else{
m_age = val;
}
}
protected:
private:
string m_Name;
string m_desc;
int m_age;
};
#endif // STUDENT_H
Student.cpp
#include "Student.h"
Student::Student()
{
cout<<"默认构造"<<endl;
}
//Student::Student(string name,string desc){
// cout<<"带参构造:Student(string name,string desc)"<<endl;
//
// m_Name = name;//等价写法:SetName(name);
//
// m_desc = desc;//等价写法:Setdesc(desc);
//}
Student::Student(string name,string desc):m_Name(name),m_desc(desc)//初始化参数列表写法,列表顺序与定义顺序无关
{
cout<<"带参构造:Student(string name,string desc)"<<endl;
}
Student::Student(int age){
cout<<"带参构造:Student(int age)"<<endl;
Setage(age);
}
void Student::ShowInfo(){
cout<<m_Name<<" "<<m_desc<<endl;
}
Student::~Student()
{
//dtor
}
main.cpp
#include <iostream>
#include "Student.h"
using namespace std;
int main()
{
cout<<"student1"<<endl;
Student student1;//直接调用默认构造。在栈内存中直接分配空间
cout<<endl<<"student2"<<endl;
Student student2("马云","一无所有杰克马");
student2.ShowInfo();
cout<<endl<<"student3 4"<<endl;
Student student3(40);
Student student4 = 40;//与student3结果相同
//Student student1 可以直接使用类和对象,方法一
//方法二
cout<<endl<<"student5"<<endl;
Student * student5 = new Student("马化腾","普通家庭");//Student 指针类型。分配内存的同时调用构造函数
student5->ShowInfo();//指针访问属性或者方法要用 ->
return 0;
}
运行结果
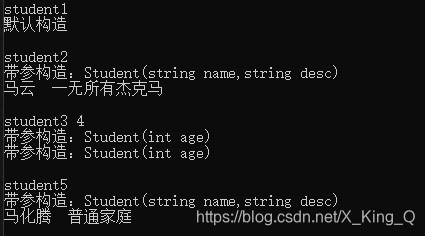