C语言编程
程序原理
还是利用wiringPI调用树莓派的GPIO,利用PWM函数中的softPwmCreate()
和softPwmWrite()
,结合变色的函数,改变颜色。
softPwmCreate:该函数将会创建一个软件控制的 PWM 管脚。可以使用任何一个 GPIO 管脚,pwmRange 参数可以为 0(关) ~100(全开)。
#include <wiringPi.h>
#include <softPwm.h>
#include <stdio.h>
#define uchar unsigned char
#define LedPinRed 0
#define LedPinGreen 1
#define LedPinBlue 2
void ledInit(void)
{
softPwmCreate(LedPinRed, 0, 100);
softPwmCreate(LedPinGreen,0, 100);
softPwmCreate(LedPinBlue, 0, 100);
}
void ledColorSet(uchar r_val, uchar g_val, uchar b_val)
{
softPwmWrite(LedPinRed, r_val);
softPwmWrite(LedPinGreen, g_val);
softPwmWrite(LedPinBlue, b_val);
}
int main(void)
{
int i;
if(wiringPiSetup() == -1){ //when initialize wiring failed, print message to screen
printf("setup wiringPi failed !");
return 1;
}
//printf("linker LedPin : GPIO %d(wiringPi pin)\n",LedPin); //when initialize wiring successfully,print message to screen
ledInit();
while(1){
ledColorSet(0xff,0x00,0x00); //red 11111111 0000000 000000
delay(500);
ledColorSet(0x00,0xff,0x00); //green
delay(500);
ledColorSet(0x00,0x00,0xff); //blue
delay(500);
ledColorSet(0xff,0xff,0x00); //yellow
delay(500);
ledColorSet(0xff,0x00,0xff); //pick
delay(500);
ledColorSet(0xc0,0xff,0x3e);
delay(500);
ledColorSet(0x94,0x00,0xd3); // 10010100 00000000 11010011
delay(500);
ledColorSet(0x76,0xee,0x00); // 01110110 11101110 00000000
delay(500);
ledColorSet(0x00,0xc5,0xcd); // 00000000 11000101 11001101
delay(500);
}
return 0;
}
重点内容
wiringPiSetup()函数
使用前,需要包含相应的文件:
#include <wiringPi.h>
#include <softPwm.h>
当编译程序时,必须加上 pthread 库,如下:
gcc –o myprog myprog.c –lwiringPi –lpthread
PS:gcc test.c -o test
在树莓派4B中亲测不可用
必须使用 wiringPiSetup()
、 wiringPiSetupGpio()
或者wiringPiSetupPhys()
函数来初始化 wiringPi。 wiringPiSetupSys()是丌够快的,因此,必须使用sudo 命令来运行程序。一些外部扩展模块有可能足够快的处理软件 PWM,在 MCP23S17 GPIO 扩展器上已经测试通过了。
Python语言编程
本次RGB LED灯的python控制看似比上次复杂,原理也是利用函数对函数的调用,原理有点类似C的指针。
#!/usr/bin/env python
import RPi.GPIO as GPIO
import time
colors = [0xFF0000, 0x00FF00, 0x0000FF, 0xFFFF00, 0xFF00FF, 0x00FFFF]
R = 11
G = 12
B = 13
def setup(Rpin, Gpin, Bpin):
global pins
global p_R, p_G, p_B
pins = {'pin_R': Rpin, 'pin_G': Gpin, 'pin_B': Bpin}
GPIO.setmode(GPIO.BOARD) # Numbers GPIOs by physical location
for i in pins:
GPIO.setup(pins[i], GPIO.OUT) # Set pins' mode is output
GPIO.output(pins[i], GPIO.HIGH) # Set pins to high(+3.3V) to off led
p_R = GPIO.PWM(pins['pin_R'], 2000) # set Frequece to 2KHz
p_G = GPIO.PWM(pins['pin_G'], 1999)
p_B = GPIO.PWM(pins['pin_B'], 5000)
p_R.start(100) # Initial duty Cycle = 0(leds off)
p_G.start(100)
p_B.start(100)
def map(x, in_min, in_max, out_min, out_max):
return (x - in_min) * (out_max - out_min) / (in_max - in_min) + out_min
def off():
for i in pins:
GPIO.output(pins[i], GPIO.HIGH) # Turn off all leds
def setColor(col): # For example : col = 0x112233
R_val = (col & 0xff0000) >> 16
G_val = (col & 0x00ff00) >> 8
B_val = (col & 0x0000ff) >> 0
R_val = map(R_val, 0, 255, 0, 100)
G_val = map(G_val, 0, 255, 0, 100)
B_val = map(B_val, 0, 255, 0, 100)
p_R.ChangeDutyCycle(100-R_val) # Change duty cycle
p_G.ChangeDutyCycle(100-G_val)
p_B.ChangeDutyCycle(100-B_val)
def loop():
while True:
for col in colors:
setColor(col)
time.sleep(1)
def destroy():
p_R.stop()
p_G.stop()
p_B.stop()
off()
GPIO.cleanup()
if __name__ == "__main__":
try:
setup(R, G, B)
loop()
except KeyboardInterrupt:
destroy()
global作用
函数定义了本地作用域,而模块定义的是全局作用域。
如果想要在函数内定义全局作用域,需要加上global修饰符。
try方法作用
try是python里用来捕获异常的,一般下面会配套有except,finally一起使用。
这里用于出现异常后keyboardinterrupt(键盘中断,用户中断执行(通常是输入^C))。
更改占空比函数:
p.ChangeDutyCycle(dc) # 范围:0.0 <= dc >= 100.0
PS:还有一个更改频率的
扫描二维码关注公众号,回复:
9502328 查看本文章
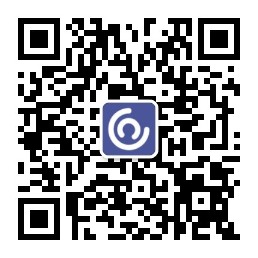
p.ChangeFrequency(freq) # freq 为设置的新频率,单位为 Hz
还有就是python内的逻辑和位移函数
语法和C相似但是对于很多的字母和符号在python中对用的数值不一样了。