Selenium是一个用于Web应用程序测试的工具。Selenium测试直接运行在浏览器中,就像真正的用户在操作一样。selenium兼容大多数常用的浏览器。这个工具的主要功能包括:测试与浏览器的兼容性——测试你的应用程序看是否能够很好得工作在不同浏览器和操作系统之上。
1、Web自动化测试环境搭建:Chrome浏览器
安装教程请点击这里。
from selenium import webdriver
from selenium.webdriver import ActionChains
from selenium.webdriver.chrome.options import Options
from selenium.webdriver.support.wait import WebDriverWait
options = Options()
# 本地chrome的路径
options.binary_location = 'C:\\Program Files (x86)\\Google\Chrome\\Application\\chrome.exe'
# 打开一个浏览器
browser = webdriver.Chrome(executable_path='D:\\softwarePacket\\chromedriver_win32\\chromedriver.exe')
此时,本地自动打开一个chrome浏览器。
2、使用selenium中的webdriver模块对浏览器进行操作
更详细的使用请参看:官方文档
browser.get('http://www.baidu.com') # 打开一个网页
element = browser.find_element_by_id('kw') # 定位一个元素,百度输入框
element.send_keys('查找的内容') # 向搜索框输入的内容
element.clear() # 清空搜索框的内容
button = browser.find_element_by_id('su') # 定位搜索框
button.click() # 点击
browser.back() # 后退
3、webdriver模块对浏览器进行操作:元素定位
3.1 webdriver API :
元素名称 | webdriver API |
---|---|
id | find_element_by_id() |
name | find_element_by_name() |
class name | find_element_by_class_name() |
tag name | find_element_by_tag_name() |
link text | find_element_by_link_text() |
partial link text | find_element_by_partial_link_text() |
xpath | find_element_by_xpath() |
css selector | find_element_by_css_selector() |
3.2 Xpath定位元素
W3School:xpath语法
3.2.1 定位元素
表达式 | 描述 |
---|---|
nodename | 选取此节点的所有子节点。 |
/ |
从根节点选取。 |
// |
从匹配选择的当前节点选择文档中的节点,而不考虑它们的位置。 |
. |
选取当前节点。 |
.. |
选取当前节点的父节点。 |
@ |
选取属性。 |
使用绝对路径
<html lang="en">
<head>
<meta charset="UTF-8">
<title>我是标题</title>
</head>
<body>
<p>我是段落1</p>
<form>
<input type="text" name="firstname"/>
<input type="text" name="lastname"/>
</form>
</body>
</html>
element = browser.find_element_by_xpath('/html/head/title') # 定位title
print(element.text)
element = browser.find_element_by_xpath('/html/body/form/input')
firstname = element.get_attribute('name')
print(firstname )
input1= browser.find_element_by_xpath('/html/body/form/input[1]')
input2= browser.find_element_by_xpath('/html/body/form/input[2]')
使用相对路径
element = browser.find_element_by_xpath('//title') #
print(element.text)
input1= browser.find_element_by_xpath('//input') # 默认返回找到的第一个
firstname = element.get_attribute('name')
print(firstname )
相对路径+绝对路径:
input1= browser.find_element_by_xpath('//form/input[1]')
input2= browser.find_element_by_xpath('//form/input[2]')
3.2.2 谓词
谓语用来查找某个特定的节点或者包含某个指定的值的节点。
谓语被嵌在方括号中。
路径表达式 | 结果 |
---|---|
/bookstore/book[1] |
选取属于 bookstore 子元素的第一个 book 元素。 |
/bookstore/book[last()] |
选取属于 bookstore 子元素的最后一个 book 元素。 |
/bookstore/book[last()-1] |
选取属于 bookstore 子元素的倒数第二个 book 元素。 |
/bookstore/book[position() < 3] |
选取最前面的两个属于 bookstore 元素的子元素的 book 元素。 |
//title[@lang] |
选取所有拥有名为 lang 的属性的 title 元素。 |
//title[@lang='eng'] |
选取所有 title 元素,且这些元素拥有值为 eng 的 lang 属性。 |
/bookstore/book[price>35.00] |
选取 bookstore 元素的所有 book 元素,且其中的 price 元素的值须大于 35.00。 |
/bookstore/book[price>35.00]/title |
选取 bookstore 元素中的 book 元素的所有 title 元素,且其中的 price 元素的值须大于 35.00。 |
3.2.3 使用统计函数
扫描二维码关注公众号,回复:
9464228 查看本文章
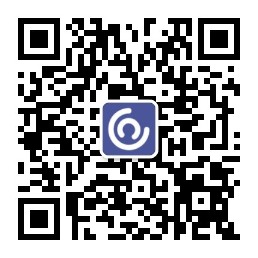
4、ActionChains类与输入事件
更多案例请点击Selenium之动作链(ActionChains)
ActionChains基本用法
首先需要了解ActionChains
的执行原理,当你调用ActionChains
的方法时,不会立即执行,而是会将所有的操作按顺序存放在一个队列里,当你调用perform()
方法时,队列中的事件会依次执行。
这种情况下我们可以有两种调用方法:
- 链式写法
from selenium.webdriver import ActionChains
menu = driver.find_element_by_css_selector(".nav")
hidden_submenu = driver.find_element_by_css_selector(".nav #submenu1")
ActionChains(driver).move_to_element(menu).click(hidden_submenu).perform()
- 分步写法
menu = driver.find_element_by_css_selector(".nav")
hidden_submenu = driver.find_element_by_css_selector(".nav #submenu1")
actions = ActionChains(driver)
actions.move_to_element(menu)
actions.click(hidden_submenu)
actions.perform()
ActionChains方法列表
鼠标事件:
click(on_element=None) ——单击鼠标左键
click_and_hold(on_element=None) ——点击鼠标左键,不松开
context_click(on_element=None) ——点击鼠标右键
double_click(on_element=None) ——双击鼠标左键
drag_and_drop(source, target) ——拖拽到某个元素然后松开
drag_and_drop_by_offset(source, xoffset, yoffset) ——拖拽到某个坐标然后松开
move_by_offset(xoffset, yoffset) ——鼠标从当前位置移动到某个坐标
move_to_element(to_element) ——鼠标移动到某个元素
move_to_element_with_offset(to_element, xoffset, yoffset) ——移动到距某个元素(左上角坐标)多少距离的位置
perform() ——执行链中的所有动作
release(on_element=None) ——在某个元素位置松开鼠标左键
键盘事件:
key_down(value, element=None) ——按下某个键盘上的键
key_up(value, element=None) ——松开某个键
send_keys(*keys_to_send) ——发送某个键到当前焦点的元素
send_keys_to_element(element, *keys_to_send) ——发送某个键到指定元素
5、selenium的三种等待方式
我们在做WEB自动化时,一般要等待页面元素加载完成后,才能执行操作,否则会报找不到元素的错误,这样就要求我们在有些场景下加等待时间。
我们平常用到的有三种等待方式:
- 强制等待
- 隐式等待
- 显示等待
细节请点击。
6、处理alter对象
方法 | 说明 |
---|---|
swich_to_altert() |
切换到alert |
accept |
确认 |
dismiss |
取消 |
send_keys() |
有输入框才能使用,否则会报错 |
driver.switch_to_alert().accept() # 点击弹出里面的确定按钮