@PropertySource注解是将配置文件中 的值赋值给POJO
项目结构如下
一.创建一个Person.Java文件:
import org.springframework.boot.context.properties.ConfigurationProperties; import org.springframework.context.annotation.PropertySource; import org.springframework.stereotype.Component; import org.springframework.validation.annotation.Validated; import javax.validation.constraints.Email; import java.util.List; import java.util.Map; @Component @ConfigurationProperties(prefix = "person") //将全局配置文件(application.properties或者application.yml中的person)赋值给该bean
//下面这个注解的作用获取配置文件中的值,可以加载多个配置文件,放在{}里面,classpath表示类路径 @PropertySource(value={"classpath:person.properties"}) @Validated //表明这个类中的数据赋值要进行数据效验 public class Person { private String lastName; private Integer age; private Boolean boss; private Map<String,Object> maps; private List<String> lists; public Map<String, Object> getMaps() { return maps; } public void setMaps(Map<String, Object> maps) { this.maps = maps; } public List<String> getLists() { return lists; } public void setLists(List<String> lists) { this.lists = lists; } public Dog getDog() { return dog; } public void setDog(Dog dog) { this.dog = dog; } private Dog dog; @Override public String toString() { return "Person{" + "lastName='" + lastName + '\'' + ", age=" + age + ", boss=" + boss + ", maps=" + maps + ", lists=" + lists + ", dog=" + dog + '}'; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public Integer getAge() { return age; } public void setAge(Integer age) { this.age = age; } public Boolean getBoss() { return boss; } public void setBoss(Boolean boss) { this.boss = boss; } }
dog类的如下:
package com.gan.springboot03.bean; public class Dog { private String name; private Integer age; @Override public String toString() { return "Dog{" + "name='" + name + '\'' + ", age=" + age + '}'; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Integer getAge() { return age; } public void setAge(Integer age) { this.age = age; } }
创建一个person.properties文件,该文件的作用是给Person赋值
person.lastName=张三
person.age=12
person.birth=2017/2/2
persion.boss=true
person.maps.k1=v1
person.maps.k2=12
person.dog.name=dog
person.dog.age=15
在测试类中测试有没有获取到Person的值
package com.gan.springboot03; import com.gan.springboot03.bean.Person; import org.junit.jupiter.api.Test; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.context.ApplicationContext; import org.springframework.test.context.junit4.SpringRunner; @SpringBootTest public class SpringBoot03ApplicationTests { @Autowired public Person person; @Test public void contextLoads() { System.out.println(person); } }
测试结果如下

@@ImportResource注解是获取bean.xml配置文件
一般情况下,都是在applicationContext中配置bean组件,例如
在service包下创建一个HelloService类,类中的代码如下:
package com.gan.springboot03.service; public class HelloService { }
创建一个配置文件用来把HelloService组件放入spring容器中
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <bean id="helloService" class="com.gan.springboot03.service.HelloService"></bean> </beans>
在核心类中,引入该配置文件
/** * @improtResource注解也可以加入多个配置文件,不过在开发中写一个配置文件再配置,很麻烦 * springboot推荐使用组件,既创建一个配置类 */ @ImportResource(locations = {"classpath:bean.xml"}) @SpringBootApplication public class SpringBoot03Application { public static void main(String[] args) { SpringApplication.run(SpringBoot03Application.class, args); } }
在测试类中测试
package com.gan.springboot03; import com.gan.springboot03.bean.Person; import org.junit.jupiter.api.Test; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.context.ApplicationContext; import org.springframework.test.context.junit4.SpringRunner; @SpringBootTest public class SpringBoot03ApplicationTests { @Autowired ApplicationContext ioc; /** * 该方法是用来判断@importSource注解,该注解的作用是导入Spring里的配置文件 * 一般是加载在主配置类上,既启动类上 */ @Test public void helloService(){ //判断是否含有helloService这个bean Boolean b=ioc.containsBean("helloService"); System.out.println(b); }
测试结果如下,返回true
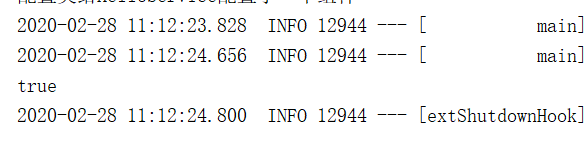
@Bean注解是为了解决每创建一个bean,就创建一个Bean配置文件,再使用注解将配置文件引入到核心类中
再config包中创建一个类MyConfig,
package com.gan.springboot03.config; import com.gan.springboot03.service.HelloService; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; /** * 配置类,用来加载Spring里面的配置文件 * Configuration注解表明这是一个配置类 */ @Configuration public class MyConfig { /** * @Bean注解将方法的返回值添加到容器中,容器中这个组件默认的id就是方法名 * @return */ @Bean public HelloService helloService(){ System.out.println("配置类给HelloService配置了一个组件"); return new HelloService(); } }
核心类配置 如下:注解掉引入配置文件的那行代码
package com.gan.springboot03; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.context.annotation.ImportResource; /** * @improtResource注解也可以加入多个配置文件,不过在开发中写一个配置文件再配置,很麻烦 * springboot推荐使用组件,既创建一个配置类 */ //@ImportResource(locations = {"classpath:bean.xml"}) @SpringBootApplication public class SpringBoot03Application { public static void main(String[] args) { SpringApplication.run(SpringBoot03Application.class, args); } }
测试类如下:
package com.gan.springboot03; import com.gan.springboot03.bean.Person; import org.junit.jupiter.api.Test; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.context.ApplicationContext; import org.springframework.test.context.junit4.SpringRunner; @SpringBootTest public class SpringBoot03ApplicationTests { @Autowired ApplicationContext ioc; /** * 该方法是用来判断@importSource注解,该注解的作用是导入Spring里的配置文件 * 一般是加载在主配置类上,既启动类上 */ @Test public void helloService(){ //判断是否含有helloService这个bean Boolean b=ioc.containsBean("helloService"); System.out.println(b); } }
测试结果如下:返回一个true
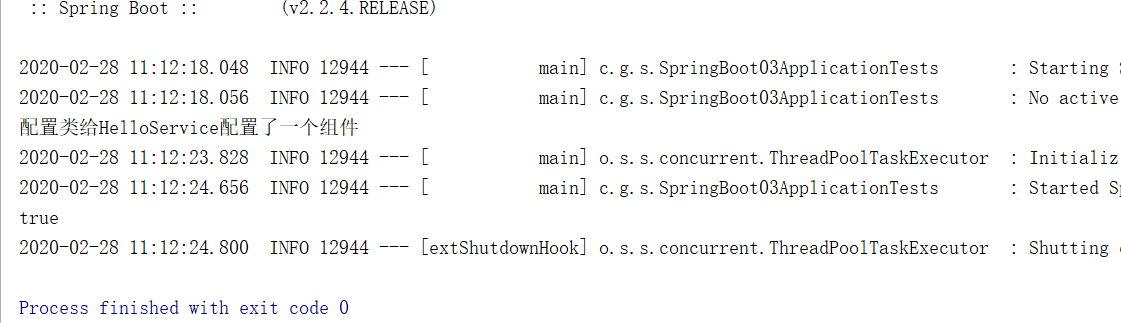