一、题目描述
Given an n-ary tree, return the preorder traversal of its nodes' values.
Nary-Tree input serialization is represented in their level order traversal,
each group of children is separated by the null value (See examples).
Follow up: Recursive solution is trivial, could you do it iteratively?
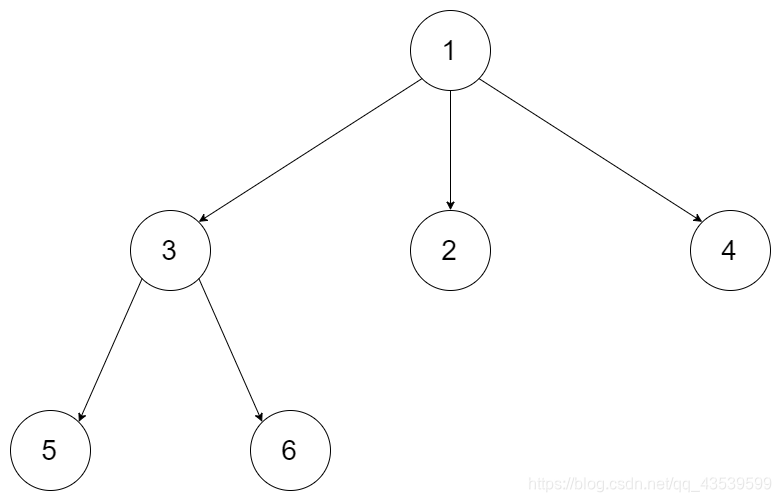
Input: root = [1,null,3,2,4,null,5,6]
Output: [1,3,5,6,2,4]
public class Node {
public int val;
public List<Node> children;
public Node() {}
public Node(int _val) {
val = _val;
}
public Node(int _val, List<Node> _children) {
val = _val;
children = _children;
}
}
二、题解
方法一:递归
联想二叉树的前序遍历,N 叉树的遍历过程类似,类似先遍历根节点 root,然后依次递归遍历每个子树 node child : root.child.
LinkedList<Integer> resList = null;
public List<Integer> preorder(Node root) {
resList = new LinkedList<>();
dfs(root);
return resList;
}
private void dfs(Node root) {
if (root == null)
return;
resList.add(root.val);
for (Node child : root.children) {
dfs(child);
}
}
复杂度分析
- 时间复杂度: ,
- 空间复杂度: ,
方法二:迭代
解释一下为什么从后往前遍历:因为遍历是从左到右的,所以先得把孩子从右往左压栈,取出结点时的顺序才是从左到右的顺序。
private void dfs(Node root) {
Stack<Node> stack = new Stack<>();
stack.push(root);
while (!stack.isEmpty()) {
Node node = stack.pop();
resList.add(node.val);
//if (root.children == null)
// continue;
for(int i = node.children.size()-1; i >= 0; i--) {
stack.add(node.children.get(i));
}
}
}
复杂度分析
- 时间复杂度: ,
- 空间复杂度: ,