Vue安装
npm install -g @vue/cli
Vue创建项目过程
1.通过命令行
vue create my-project
2.还有一种方式就是通过vue ui来打开可视化的页面安装,这里记录下我用vue ui来创建项目
vue ui
存在一个问题,就是有电脑上面会报错,如下:
pc@pc-PC MINGW64 /e/vue/test
$ vue ui
module.js:549
throw err;
^
Error: Cannot find module 'core-js/proposals/array-flat-and-flat-map'
at Function.Module._resolveFilename (module.js:547:15)
at Function.Module._load (module.js:474:25)
at Module.require (module.js:596:17)
at require (internal/module.js:11:18)
at Object.<anonymous> (C:\Users\zyz\AppData\Roaming\npm\node_modules\@vue\cli\node_modules\apollo-env\lib\polyfills\array.js:3:1)
at Module._compile (module.js:652:30)
at Object.Module._extensions..js (module.js:663:10)
at Module.load (module.js:565:32)
at tryModuleLoad (module.js:505:12)
at Function.Module._load (module.js:497:3)
解决方式(重新安装一遍vue):
$ npm uninstall @vue/cli -g
$ npm install @vue/cli -g
运行
$ vue ui
说明:这里可以不选择Linter/Formatter,该选项的作用是在后来写代码的时候使用ESLint规范,这个规范比较麻烦,就像严格的老师一样,总是报错,比如这个地方应该用多少个空格啦,这个地方应该用const啦,当然这是一个好的代码风格。
说明:第一个选项可以选择,意思是项目是否用history模式,这种模式下项目地址中将不会有“#”
3.一个用于浏览器上的Vue调试插件
Vue.js devtools
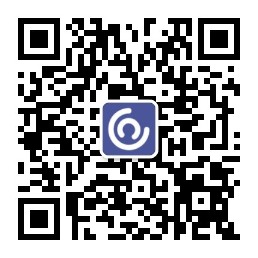
Vue项目文件结构
以上文件目录也仅仅是我自己的理解,我的想法是每个views文件下的Vue组件对应于每一个页面,在这每一个Vue组件页面中又可以包含多个子组件,这些子组件放在components文件夹下,components文件夹下包含多个文件夹,每个文件夹对应相应页面的子组件,assets文件夹我把它来存放一些Imgs,public文件夹下存放的是base.css,用于规定页面中一些基本的css,这里可以参考css reset,或者是 normalize.css。
webstorm中使用ESLint
安装ESLint插件
配置ESLint插件
第一个框填写nodejs的地址,第二个框填写项目中eslint.cmd的地址,第三个框填写.eslintrc.js
ESLint的使用
Vuex的使用说明
state: {
enterpriseLeft: Object,
loginData: Object,
enterpriseInfo: Array,
count: 1
},
mutations: {
changeEnterpriseLeft: function (state,data) {
this.state.enterpriseLeft = data;
},
login: function (state,data) {
this.state.loginData = data
},
getEnterpriseInfo: function (state,data) {
this.state.enterpriseInfo = data
},
doubleCount: funciton (state) {
this.state.count*=2;
},
changeCount:function (state,data) {
state.count=data;
}
},
actions: {
//同步操作
ansy: function (context) {
context.commit('count');
},
//异步操作,其中包含有setTimeout这类异步函数
async: function (context) {
setTimeout(() => {
context.commit('count');
}, 2000)
},
changeCount (context,payload){
context.commit('changeCount',payload);
context.commit('changeCount',payload.payload);
}
}
- action用于异步请求,多数情况下action处理异步数据最终提交到数据库
- mutation用来同步数据处理 ,Vuex中store数据改变唯一的方法就是mutation
- Action 提交的是 mutation,而不是直接变更状态。
import store from '@/store'
mounted: function () {
store.commit('count');
store.dispatch('async');
}
以上代码展示的是在vue组件中调用mutation和action方法的区别
store.commit('login',response.data);
methods: {
changeCount: function(){
this.$store.dispatch('changeCount',this.input_value);
this.$store.dispatch({
type: 'changeCount',
payload: this.input_value
});
},
}
以上代码展示的是利用commit和dispatch来传递参数的方式
router的使用说明
routes: [{
path: '/',
name: 'home',
component: () =>
import ( /* webpackChunkName: "about" */ './views/Home.vue')
},
{
path: '/query',
name: 'query',
component: () =>
import ( /* webpackChunkName: "about" */ './views/Query.vue')
},
{
path: '/login',
name: 'login',
component: () =>
import ( /* webpackChunkName: "about" */ './views/Login.vue')
},
{
path: '/enterprise',
name: 'enterprise',
component: () =>
import ( /* webpackChunkName: "about" */ './views/Enterprise.vue')
}]
axios的使用说明
import axios from 'axios'
import qs from 'qs'
changeRightInfo: function () {
axios.post('/enterprise',qs.stringify({
title: this.list.title,
listChoose: this.listChoose
}),
{
headers: {
'Content-Type': 'application/x-www-form-urlencoded',
'timeout': 6000
}
})
.then((response) => {
if(response.status === 200){
store.commit('getEnterpriseInfo',response.data);
//调用子组件中的函数
this.$refs.enterpriseInformation.init();
}
})
.catch((error) => {
console.log(error);
});
}
模拟服务端的返回利用axios-mock-adapter
import MockAdapter from 'axios-mock-adapter'
const mock = new MockAdapter(axios);
mock.onPost('/enterprise').reply(200, [{
name: '若水上善',
value: ''
}]);
Vue编程中的一些小问题
问题1:
@click中有时候会有莫名的问题,加上阻止默认行为.prevent以及阻止冒泡.stop就可以了,在@click中执行多个methods中的方法
@click.prevent.stop="changeRightHeader(id);changeRightInfo(id)"
问题2:
父组件调用子组件的方法
<enterpriseInformation ref="enterpriseInformation"></enterpriseInformation>
在父组件的需要调用的地方利用refs,子组件的methods中写上init方法
this.$refs.enterpriseInformation.init();
问题3:
子组件调用父组件的方法
this.$emit('refreshList');
// 如果要传递给父组件很多值,这些值要作为参数依次列出 如 this.$emit('valueUp', this.inputValue, this.mesFather);
在父组件中有,changeLeftList()为父组件中定义的方法
<EnterpriseBanner @refreshList="changeLeftList()"></EnterpriseBanner>
问题4:
有关vue中图片的处理
我的想法是通过父组件的属性向子组件传值
//父组件中属性设置
<EnterpriseDemonstrate
enterpriseName="智慧农业软件平台"
enterpriseDescribe="智诚乐创"
enterpriseImg="1"
></EnterpriseDemonstrate>
//子组件中props
props:{
enterpriseName: String,
enterpriseDescribe: String,
enterpriseImg: String
},
vue中加载图片的方式是通过require('图片的地址'),其中图片的地址还不能带有变量,否则会报错
data() {
return{
imgUrl1: require('../../assets/QueryImgs/e1.jpg'),
imgUrl2: require('../../assets/QueryImgs/e2.jpg')
}
},
methods: {
imgChoose: function () {
switch (parseInt(this.enterpriseImg)) {
case 1:
return(this.imgUrl1);
break;
case 2:
return(this.imgUrl2);
break;
}
}
}
<img :src="imgChoose()" alt="" class="enterprise-img"/>