写在前面: 很久没有好好打,松懈了,只打出三道,而且有错误,第四道是经典枚举,没想到是很不应该的。
A. Add Odd or Subtract Even
time limit per test2 seconds
memory limit per test256 megabytes
inputstandard input
outputstandard output
You are given two positive integers a and b.
In one move, you can change a in the following way:
Choose any positive odd integer x (x>0) and replace a with a+x;
choose any positive even integer y (y>0) and replace a with a−y.
You can perform as many such operations as you want. You can choose the same numbers x and y in different moves.
Your task is to find the minimum number of moves required to obtain b from a. It is guaranteed that you can always obtain b from a.
You have to answer t independent test cases.
Input
The first line of the input contains one integer t (1≤t≤104) — the number of test cases.
Then t test cases follow. Each test case is given as two space-separated integers a and b (1≤a,b≤109).
Output
For each test case, print the answer — the minimum number of moves required to obtain b from a if you can perform any number of moves described in the problem statement. It is guaranteed that you can always obtain b from a.
Example
inputCopy
5
2 3
10 10
2 4
7 4
9 3
outputCopy
1
0
2
2
1
Note
In the first test case, you can just add 1.
In the second test case, you don’t need to do anything.
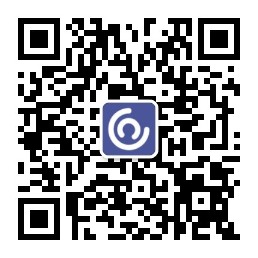
In the third test case, you can add 1 two times.
In the fourth test case, you can subtract 4 and add 1.
In the fifth test case, you can just subtract 6.
思路: 水题,找一下情况就可,a > b两种,a < b两种。
#include <iostream>
#include <algorithm>
#include <cstdio>
#include <string>
#include <cstring>
#include <vector>
#include <map>
#include <cmath>
#include <set>
using namespace std;
#define endl '\n'
typedef long long ll;
int main()
{
int t;
cin >> t;
while (t--)
{
ll a, b;
cin >> a >> b;
if (a == b)
{
cout << "0" << endl;
continue;
}
if (a < b && a % 2 == 0 && b % 2== 1)
{
cout << "1" << endl;
continue;
}
if ((a - b) > 0 && (a - b) % 2 == 0)
{
cout << "1" << endl;
continue;
}
if ((b - a) > 0 && (b - a) % 2 == 1)
{
cout << "1" << endl;
continue;
}
cout << "2" << endl;
}
return 0;
}
B. WeirdSort
time limit per test2 seconds
memory limit per test256 megabytes
inputstandard input
outputstandard output
You are given an array a of length n.
You are also given a set of distinct positions p1,p2,…,pm, where 1≤pi<n. The position pi means that you can swap elements a[pi] and a[pi+1]. You can apply this operation any number of times for each of the given positions.
Your task is to determine if it is possible to sort the initial array in non-decreasing order (a1≤a2≤⋯≤an) using only allowed swaps.
For example, if a=[3,2,1] and p=[1,2], then we can first swap elements a[2] and a[3] (because position 2 is contained in the given set p). We get the array a=[3,1,2]. Then we swap a[1] and a[2] (position 1 is also contained in p). We get the array a=[1,3,2]. Finally, we swap a[2] and a[3] again and get the array a=[1,2,3], sorted in non-decreasing order.
You can see that if a=[4,1,2,3] and p=[3,2] then you cannot sort the array.
You have to answer t independent test cases.
Input
The first line of the input contains one integer t (1≤t≤100) — the number of test cases.
Then t test cases follow. The first line of each test case contains two integers n and m (1≤m<n≤100) — the number of elements in a and the number of elements in p. The second line of the test case contains n integers a1,a2,…,an (1≤ai≤100). The third line of the test case contains m integers p1,p2,…,pm (1≤pi<n, all pi are distinct) — the set of positions described in the problem statement.
Output
For each test case, print the answer — “YES” (without quotes) if you can sort the initial array in non-decreasing order (a1≤a2≤⋯≤an) using only allowed swaps. Otherwise, print “NO”.
Example
inputCopy
6
3 2
3 2 1
1 2
4 2
4 1 2 3
3 2
5 1
1 2 3 4 5
1
4 2
2 1 4 3
1 3
4 2
4 3 2 1
1 3
5 2
2 1 2 3 3
1 4
outputCopy
YES
NO
YES
YES
NO
YES
思路: 题意是判断是不是能够通过交换达成不递减数列,我用结构体记录下标与值,然后对值重新排序,新的下标到原来的下标之间所有的插值都必须存在才可以移动。注意 如果是相同则可以不移动到要求位置,直接跳出就可。
#include <iostream>
#include <algorithm>
#include <cstdio>
#include <string>
#include <cstring>
#include <vector>
#include <map>
#include <cmath>
#include <set>
using namespace std;
#define endl '\n'
typedef long long ll;
struct node {
int data;
int num;
};
bool cmp(node a, node b)
{
return a.data < b.data;
}
int main()
{
int t;
cin >> t;
while (t--)
{
int n, m;
int a[1001], p[1001], kk[1001];
for (int i = 0; i <= 500; ++i)
kk[i] = 0;
node b[1001];
cin >> n >> m;
for (int i = 1; i <= n; ++i)
{
cin >> a[i];
b[i].data = a[i];
b[i].num = i;
}
for (int i = 0; i < m; ++i)
{
cin >> p[i];
kk[p[i]] = 1;
}
sort(b + 1, b + 1 + n, cmp);
int f = 0;
for (int i = 1; i <= n; ++i)
{
if (b[i].num > i)
for (int j = b[i].num - 1; j >= i; --j)
{
if (b[j].data == b[i].data)
break;
if (kk[j] == 0)
{
f = 1;
break;
}
}
else if (b[i].num < i)
for (int j = i - 1; j >= b[i].num; --j)
{
if (b[j].data == b[i].data)
break;
if (kk[j] == 0)
{
f = 1;
break;
}
}
if (f)
break;
}
if (f)
cout << "NO" << endl;
else
cout << "YES" <<endl;
}
return 0;
}
C. Perform the Combo
time limit per test2 seconds
memory limit per test256 megabytes
inputstandard input
outputstandard output
You want to perform the combo on your opponent in one popular fighting game. The combo is the string s consisting of n lowercase Latin letters. To perform the combo, you have to press all buttons in the order they appear in s. I.e. if s=“abca” then you have to press ‘a’, then ‘b’, ‘c’ and ‘a’ again.
You know that you will spend m wrong tries to perform the combo and during the i-th try you will make a mistake right after pi-th button (1≤pi<n) (i.e. you will press first pi buttons right and start performing the combo from the beginning). It is guaranteed that during the m+1-th try you press all buttons right and finally perform the combo.
I.e. if s=“abca”, m=2 and p=[1,3] then the sequence of pressed buttons will be ‘a’ (here you’re making a mistake and start performing the combo from the beginning), ‘a’, ‘b’, ‘c’, (here you’re making a mistake and start performing the combo from the beginning), ‘a’ (note that at this point you will not perform the combo because of the mistake), ‘b’, ‘c’, ‘a’.
Your task is to calculate for each button (letter) the number of times you’ll press it.
You have to answer t independent test cases.
Input
The first line of the input contains one integer t (1≤t≤104) — the number of test cases.
Then t test cases follow.
The first line of each test case contains two integers n and m (2≤n≤2⋅105, 1≤m≤2⋅105) — the length of s and the number of tries correspondingly.
The second line of each test case contains the string s consisting of n lowercase Latin letters.
The third line of each test case contains m integers p1,p2,…,pm (1≤pi<n) — the number of characters pressed right during the i-th try.
It is guaranteed that the sum of n and the sum of m both does not exceed 2⋅105 (∑n≤2⋅105, ∑m≤2⋅105).
Output
For each test case, print the answer — 26 integers: the number of times you press the button ‘a’, the number of times you press the button ‘b’, …, the number of times you press the button ‘z’.
Example
inputCopy
3
4 2
abca
1 3
10 5
codeforces
2 8 3 2 9
26 10
qwertyuioplkjhgfdsazxcvbnm
20 10 1 2 3 5 10 5 9 4
outputCopy
4 2 2 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0
0 0 9 4 5 3 0 0 0 0 0 0 0 0 9 0 0 3 1 0 0 0 0 0 0 0
2 1 1 2 9 2 2 2 5 2 2 2 1 1 5 4 11 8 2 7 5 1 10 1 5 2
Note
The first test case is described in the problem statement. Wrong tries are “a”, “abc” and the final try is “abca”. The number of times you press ‘a’ is 4, ‘b’ is 2 and ‘c’ is 2.
In the second test case, there are five wrong tries: “co”, “codeforc”, “cod”, “co”, “codeforce” and the final try is “codeforces”. The number of times you press ‘c’ is 9, ‘d’ is 4, ‘e’ is 5, ‘f’ is 3, ‘o’ is 9, ‘r’ is 3 and ‘s’ is 1.
思路: 统计字母出现次数,有点后缀和的意思。
#include <iostream>
#include <algorithm>
#include <cstdio>
#include <string>
#include <cstring>
#include <vector>
#include <map>
#include <cmath>
#include <set>
using namespace std;
#define endl '\n'
typedef long long ll;
map<char, int>mp;
int main()
{
int t;
cin >> t;
while (t--)
{
int n, m;
cin >> n >> m;
string s;
cin >> s;
int p[200005];
for (int i = 0; i <= n; ++i)
p[i] = 0;
// map<int, int>mt;
ll mn[26];
for (int i = 0; i < 26; ++i)
mn[i] = 0;
for (int i = 0; i < m; ++i)
{
int x;
cin >> x;
p[x - 1]++;
// mt[p[i]]++;
}
ll num = 0;
for (int i = n - 1; i >= 0; --i)
{
if (p[i] != 0)
num += p[i];
mn[s[i] - 'a'] += (num + 1);
}
for (int i = 0; i < 26; ++i)
if (i == 0)
cout << mn[i];
else
cout << " " << mn[i];
cout << endl;
}
return 0;
}
D. Three Integers
time limit per test2 seconds
memory limit per test256 megabytes
inputstandard input
outputstandard output
You are given three integers a≤b≤c.
In one move, you can add +1 or −1 to any of these integers (i.e. increase or decrease any number by one). You can perform such operation any (possibly, zero) number of times, you can even perform this operation several times with one number. Note that you cannot make non-positive numbers using such operations.
You have to perform the minimum number of such operations in order to obtain three integers A≤B≤C such that B is divisible by A and C is divisible by B.
You have to answer t independent test cases.
Input
The first line of the input contains one integer t (1≤t≤100) — the number of test cases.
The next t lines describe test cases. Each test case is given on a separate line as three space-separated integers a,b and c (1≤a≤b≤c≤104).
Output
For each test case, print the answer. In the first line print res — the minimum number of operations you have to perform to obtain three integers A≤B≤C such that B is divisible by A and C is divisible by B. On the second line print any suitable triple A,B and C.
Example
inputCopy
8
1 2 3
123 321 456
5 10 15
15 18 21
100 100 101
1 22 29
3 19 38
6 30 46
outputCopy
1
1 1 3
102
114 228 456
4
4 8 16
6
18 18 18
1
100 100 100
7
1 22 22
2
1 19 38
8
6 24 48
思路: 就是一个简单枚举,没想出来,自闭!!!
感谢大佬帮我指出错误,枚举应该更大,然后才可。补题hack了。。。
#include <iostream>
#include <algorithm>
#include <cstdio>
#include <string>
#include <cstring>
#include <vector>
#include <map>
#include <cmath>
#include <set>
using namespace std;
#define endl '\n'
typedef long long ll;
map<char, int>mp;
int main()
{
int t;
cin >> t;
while (t--)
{
ll m = 1e9 + 7;
int a, b, c, aa, bb, cc;
cin >> a >> b >> c;
for (int i = 1; i <= 20000; ++i)
for (int j = i; j <= 20000; j += i)
for (int k = j; k <= 20000; k += j)
{
ll mx = abs(a - i) + abs(b - j) + abs(c - k);
if (mx < m)
{
m = mx;
aa = i, bb = j, cc = k;
}
}
cout << m << endl;
cout << aa << " " << bb << " " << cc << endl;
}
return 0;
}