初到蓝杰,才真正开始了我的代码生涯,以前也只是随便写过两句,没有一个大的项目或者目标,也不知道要去写什么,怎么去写。
首先,通过画图板项目,来学习java的基础知识。
各模块功能
drawUI类
1、 设计窗体(名称、大小、位置、背景、关闭时结束程序等)
JFrame ui = new JFrame();
//设置窗体属性
ui.setTitle("画图板--倪颢");
ui.setSize(1000, 800);
//ui.setLocation(300, 300);
ui.setLocationRelativeTo(null);//居中
//关闭时结束程序
ui.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
ui.setBackground(Color.yellow);
2、设置布局(具体内容会在之后的博客中)
FlowLayout flow = new FlowLayout();
ui.setLayout(flow);
3、添加鼠标监听器
mouselistener mousel = new mouselistener();
ui.addMouseListener(mousel);
4、添加组件(这里以添加按钮为例)
String textArr[] = {"直线", "点", "迭代"};
for (String text : textArr) {
JButton btn = new JButton(text);
Dimension botton = new Dimension(100, 50);
btn.setPreferredSize(botton);
ui.add(btn);
btn.addActionListener(mousel);
}
5、在窗体全都完成后,设置可见,并获取画布对象
ui.setVisible(true);
//创建画布对象,必须在设置可见后
Graphics g = ui.getGraphics();
mousel.setgraphic(g);
6、主函数
public static void main(String args[]) {
drawUI drawui = new drawUI();
drawui.showUI();
}
监听器类
1、鼠标点击
public void mouseClicked(MouseEvent e) {
a、选择“点”按钮
g.fillOval((int)startx - r, (int)starty-r, r,r);
b、选择“迭代”按钮
point[clicknum%4][0]=e.getX();
point[clicknum%4][1]=e.getY();
//g.drawLine(ax, ay, ax, ay);
g.fillOval(point[clicknum%4][0] - r, point[clicknum%4][1] - r, r, r);
clicknum++;
if (clicknum % 4 == 0) {
System.out.println("(" + point[0][0] + "," + point[0][1] + ")" + "(" + point[1][0] + "," + point[1][1] + ")" + "(" + point[2][0] + "," + point[2][1] + ")" + "(" + point[3][0] + "," + point[3][1] + ")");
for (int i = 0; i <= 10000; i++) {
int temp = 0;
temp = (int) (Math.random() * 3);
if (temp == 0) {
point[4][0] = (point[0][0] + point[3][0]) / 2;
point[4][1] = (point[0][1] + point[3][1]) / 2;
} else if (temp == 1) {
point[4][0] = (point[1][0] + point[3][0]) / 2;
point[4][1] = (point[1][1] + point[3][1]) / 2;
} else if (temp == 2) {
point[4][0] = (point[2][0] + point[3][0]) / 2;
point[4][1] = (point[2][1] + point[3][1]) / 2;
}
g.fillOval(point[3][0] - r, point[3][1] - r, r, r);
point[3][0] = point[4][0];
point[3][1] = point[4][1];
}
}
第二种迭代(还有很多有趣的图案,大家可以去试试)
startx = e.getX();
starty = e.getY();
for (int i = 0; i < 10000; i++) {
System.out.println("(" + startx + "," + starty + ")" );
g.drawLine((int)(startx*100)+300 - r, (int)(starty*100)+300-r,(int)(startx*100)+300 - r, (int)(starty*100)+300-r);
tempx = Math.sin(a * starty) + c * Math.cos(a * startx);
tempy = Math.sin(b * startx) + d * Math.cos(b * starty);
startx = tempx;
starty = tempy;
}
2、鼠标松开触发器
public void mouseReleased(MouseEvent e) {
if (bottonText.equals("直线")) {
endx = e.getX();
endy = e.getY();
for (int i = 0; i <= 256; i++) {
Color c = new Color(200, 200, i);
g.setColor(c);
g.drawLine((int)startx, (int)starty, (int)endx, (int)endy);
}
}
}
3、屏幕点击触发器
public void actionPerformed(ActionEvent e) {
if ("直线".equals(e.getActionCommand())) {
bottonText = "直线";
} else if ("点".equals(e.getActionCommand())) {
bottonText = "点";
} else if ("迭代".equals(e.getActionCommand())) {
bottonText = "迭代";
}
}
总结
也没什么好总结的,找到规律,写好代码其实不难,我今后会将写的项目和遇到的问题写成博客,欢迎各位大佬前来交流指导。
扫描二维码关注公众号,回复:
9406151 查看本文章
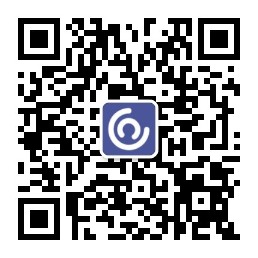
附录(源代码)
1、drawUI类
package drawline;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.FlowLayout;
import javax.swing.JFrame;
import java.awt.Graphics;
import javax.swing.JButton;
public class drawUI {
public void showUI() {
JFrame ui = new JFrame();
//设置窗体属性
ui.setTitle("画图板--倪颢");
ui.setSize(1000, 800);
//ui.setLocation(300, 300);
ui.setLocationRelativeTo(null);//居中
ui.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
ui.setBackground(Color.yellow);
//设置布局
FlowLayout flow = new FlowLayout();
ui.setLayout(flow);
//添加鼠标监听器
mouselistener mousel = new mouselistener();
ui.addMouseListener(mousel);
//添加组件(按钮)
String textArr[] = {"直线", "点", "迭代"};
for (String text : textArr) {
JButton btn = new JButton(text);
Dimension botton = new Dimension(100, 50);
btn.setPreferredSize(botton);
ui.add(btn);
btn.addActionListener(mousel);
}
//设置可见
ui.setVisible(true);
//创建画布对象,必须在设置可见后
Graphics g = ui.getGraphics();
mousel.setgraphic(g);
}
public static void main(String args[]) {
drawUI drawui = new drawUI();
drawui.showUI();
}
}
2、mouselistener类(刚开始名称书写还是不规范)
package drawline;
import java.awt.Color;
import java.awt.Graphics;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import javax.swing.JOptionPane;
public class mouselistener implements MouseListener, ActionListener {
double startx, starty, endx, endy, tempx, tempy;
double a=-1.8,b=-2.0,c=-0.5,d=-0.9;
int[][] point = new int[5][2];
int r = 6;//设置点的半径
int clicknum = 0;
String bottonText = "";
Graphics g;
public void setgraphic(Graphics g) {
this.g = g;
}
public void mouseClicked(MouseEvent e) {
if (bottonText.equals("迭代")) {
point[clicknum%4][0]=e.getX();
point[clicknum%4][1]=e.getY();
//g.drawLine(ax, ay, ax, ay);
g.fillOval(point[clicknum%4][0] - r, point[clicknum%4][1] - r, r, r);
clicknum++;
if (clicknum % 4 == 0) {
System.out.println("(" + point[0][0] + "," + point[0][1] + ")" + "(" + point[1][0] + "," + point[1][1] + ")" + "(" + point[2][0] + "," + point[2][1] + ")" + "(" + point[3][0] + "," + point[3][1] + ")");
for (int i = 0; i <= 10000; i++) {
int temp = 0;
temp = (int) (Math.random() * 3);
if (temp == 0) {
point[4][0] = (point[0][0] + point[3][0]) / 2;
point[4][1] = (point[0][1] + point[3][1]) / 2;
} else if (temp == 1) {
point[4][0] = (point[1][0] + point[3][0]) / 2;
point[4][1] = (point[1][1] + point[3][1]) / 2;
} else if (temp == 2) {
point[4][0] = (point[2][0] + point[3][0]) / 2;
point[4][1] = (point[2][1] + point[3][1]) / 2;
}
g.fillOval(point[3][0] - r, point[3][1] - r, r, r);
point[3][0] = point[4][0];
point[3][1] = point[4][1];
}
}
/*
startx = e.getX();
starty = e.getY();
for (int i = 0; i < 10000; i++) {
System.out.println("(" + startx + "," + starty + ")" );
g.drawLine((int)(startx*100)+300 - r, (int)(starty*100)+300-r,(int)(startx*100)+300 - r, (int)(starty*100)+300-r);
tempx = Math.sin(a * starty) + c * Math.cos(a * startx);
tempy = Math.sin(b * startx) + d * Math.cos(b * starty);
startx = tempx;
starty = tempy;
}*/
} else if (bottonText.equals("点")) {
g.fillOval((int)startx - r, (int)starty-r, r,r);
}
}
public void mousePressed(MouseEvent e) {
if (bottonText.equals("")) {
JOptionPane.showMessageDialog(null, "请选择一个按钮", "错误", JOptionPane.ERROR_MESSAGE);
}
startx = e.getX();
starty = e.getY();
}
public void mouseReleased(MouseEvent e) {
if (bottonText.equals("直线")) {
endx = e.getX();
endy = e.getY();
for (int i = 0; i <= 256; i++) {
Color c = new Color(200, 200, i);
g.setColor(c);
g.drawLine((int)startx, (int)starty, (int)endx, (int)endy);
}
}
}
public void mouseEntered(MouseEvent e) {
}
public void mouseExited(MouseEvent e) {
}
public void actionPerformed(ActionEvent e) {
if ("直线".equals(e.getActionCommand())) {
bottonText = "直线";
} else if ("点".equals(e.getActionCommand())) {
bottonText = "点";
} else if ("迭代".equals(e.getActionCommand())) {
bottonText = "迭代";
}
}
}