Leetcode198. House Robber
You are a professional robber planning to rob houses along a street. Each house has a certain amount of money stashed, the only constraint stopping you from robbing each of them is that adjacent houses have security system connected and it will automatically contact the police if two adjacent houses were broken into on the same night.
Given a list of non-negative integers representing the amount of money of each house, determine the maximum amount of money you can rob tonight without alerting the police.
Example 1:
Input: [1,2,3,1]
Output: 4
Explanation: Rob house 1 (money = 1) and then rob house 3 (money = 3).
Total amount you can rob = 1 + 3 = 4.
Example 2:
Input: [2,7,9,3,1]
Output: 12
Explanation: Rob house 1 (money = 2), rob house 3 (money = 9) and rob house 5 (money = 1).
Total amount you can rob = 2 + 9 + 1 = 12.
思路
需要求前 n 家商店最多能偷多少钱,并且知道了前 n - 1 家店最多能偷的钱数,前 n - 2 家店最多能偷的钱数。
对于第 n 家店,我们只能选择偷或者不偷。
如果偷的话,那么前 n 家商店最多能偷的钱数就是「前 n - 2 家店最多能偷的钱数」加上「第 n 家店的钱数」。因为选择偷第 n 家商店,第 n - 1 家商店就不可以偷了。
如果不偷的话,那么前 n 家商店最多能偷的钱数就是「前 n - 1 家店最多能偷的钱数」。
最终前 n 家商店最多能偷的钱数就是上边两种情况选择较大的值。
递归 -> 递归加缓冲 -> 动态规划 -> 动态规划空间复杂度优化
递归
当 n = 0,也就没有商店,那么能偷的最大钱数当然是 0 了。
当 n = 1,也就是只有一家店的时候,能偷的最大钱数就是当前店的钱数。
public int rob(int[] nums) {
return robHelpler(nums, nums.length);
}
private int robHelpler(int[] nums, int n) {
if (n == 0) {
return 0;
}
if (n == 1) {
return nums[0];
}
return Math.max(robHelpler(nums, n - 2) + nums[n - 1], robHelpler(nums, n - 1));
}
使用数组优化
public int rob(int[] nums) {
int[] map = new int[nums.length + 1];
Arrays.fill(map, -1);
return robHelpler(nums, nums.length, map);
}
private int robHelpler(int[] nums, int n, int[] map) {
if (n == 0) {
return 0;
}
if (n == 1) {
return nums[0];
}
if (map[n] != -1) {
return map[n];
}
int res = Math.max(robHelpler(nums, n - 2, map) + nums[n - 1], robHelpler(nums, n - 1, map));
map[n] = res;
return res;
}
动态规划
用dp[n]
数组表示前 n
天能够带来的最大收益。
dp[n] = Math.max(dp[n - 2] + nums[n - 1], dp[n - 1] )
。
初始条件:dp[0] = 0
以及 dp[1] = nums[0]
。
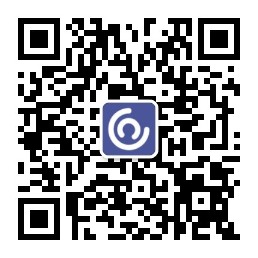
public int rob(int[] nums) {
int n = nums.length;
if (n == 0) {
return 0;
}
if (n == 1) {
return nums[0];
}
int[] dp = new int[n + 1];
dp[0] = 0;
dp[1] = nums[0];
for (int i = 2; i <= n; i++) {
dp[i] = Math.max(dp[i - 2] + nums[i - 1], dp[i - 1]);
}
return dp[n];
}
空间复杂度优化
public int rob(int[] nums) {
int n = nums.length;
if (n == 0) {
return 0;
}
if (n == 1) {
return nums[0];
}
int pre = 0; //代替上边代码中的 dp[i - 2]
int cur = nums[0]; //代替上边代码中的 dp[i - 1] 和 dp[i]
for (int i = 2; i <= n; i++) {
int temp = cur;
cur = Math.max(pre + nums[i - 1], cur);
pre = temp;
}
return cur;
}