当我们做出一个软件的时候,如果想让用户有属于自己账号密码,这时候就需要用服务端来完成,而我们服务端的数据库一般就采用MySQL,服务端的开发环境我使用的是MyEclipse。
如果我们想要对数据库进行操作,就必须先获取连接。而要获取连接,我们需要4个非常重要的字符串,首先我们先在MyEclipse新建一个项目,新建一个后缀为Properties的文件,Properties就是集合中一种,该文件会以键值对的方式存储。前面说的4个重要的字符串就是要存储到这个文件中的值,至于键就看我们的喜好了,这是我的Properties文件
第一行字符串用于加载MySQL的JDBC驱动,当然我们要加载JDBC驱动,要在WebRoot文件下的WEB-INF-->lib文件放入一个启动JDBC的文件,下面附上下载链接
http://download.csdn.net/detail/ning109314/2543435
第二行字符串为服务端的主机项目地址,localhost为主机,3306为端口号,?后面为使用的字符集及编码集。主机可以用当前ip地址替代
第三第四就是注册数据库的时候使用的账号跟密码,照着你注册时输入即可
做完这些准备工作,我们就可以获取连接了。
下面是获取连接数据库,和关闭连接的方法
扫描二维码关注公众号,回复:
906115 查看本文章
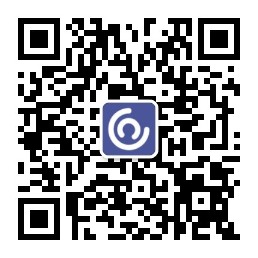
package com.zx.news.dbutil; import java.io.IOException; import java.io.InputStream; import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; import java.util.Properties; public class DBUtil { /** * 获取连接 * 要获取连接才能对数据库进行操作 * @throws IOException * @throws ClassNotFoundException * @throws SQLException */ public static Connection getConnection() throws IOException, ClassNotFoundException, SQLException{ //util包下的 Properties properties = new Properties(); DBUtil dbUtil = new DBUtil(); //获取流 Class<? extends DBUtil> class1 = dbUtil.getClass(); ClassLoader classLoader = class1.getClassLoader();//获取流的加载对象 InputStream inStream = classLoader.getResourceAsStream("com/zx/news/dbutil/DbConfig.Properties");//以流的形式获取资源 // FileInputStream inStream = new FileInputStream("/News/src/com/zx/news/dbutil/DbConfig.Properties"); properties.load(inStream);//读取流 //获取该文件下的值 String driver = properties.getProperty("driver"); String url = properties.getProperty("url"); String userName = properties.getProperty("username"); String password = properties.getProperty("password"); //加载MySQL的JDBC的驱动 Class.forName(driver); //获取连接 Connection connection = DriverManager.getConnection(url, userName, password); return connection; } /** * 关闭连接 */ public static void closeConnection(Connection connection) { if (connection != null) { try { connection.close(); } catch (SQLException e) { e.printStackTrace(); } } } }
当然,我们获取连接的目的是为了对数据库进行操作。对数据的操作就要用到SQL语句了,无外乎增、删、改、查。当然如果用户注册账号的话我们还需要判断这个账户是否存在,登陆需要判断账号或者密码是否正确。这些方法我们都应该提供,如下:
package com.zx.news.dbutil; import java.io.IOException; import java.sql.Connection; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; public class AccountManager { Connection connection; public AccountManager() throws IOException, ClassNotFoundException, SQLException { // 再构造方法中获取连接的对象 connection = DBUtil.getConnection(); } /** * 添加数据的方法 * * @return ture添加成功,反之失败 * @throws SQLException * @throws ClassNotFoundException * @throws IOException */ public boolean register(String userName, String password) throws SQLException { String sql = "insert into account(username,password) value('" + userName + "','" + password + "')"; return update(sql); } /** * 删除数据的方法 * * @throws SQLException * @throws ClassNotFoundException * @throws IOException */ public boolean delete(int id, String userName) throws SQLException, IOException, ClassNotFoundException { String sql = "delete from account where id ='" + id + "'or username ='" + userName + "'"; return update(sql); } /** * 增、删、改 * @throws SQLException */ public boolean update(String sql) throws SQLException { Statement statement = connection.createStatement(); int update = statement.executeUpdate(sql); if (update == 0) { return false; } else { return true; } } /** * 查找 * @throws SQLException */ public void select() throws SQLException { Statement statement = connection.createStatement(); String sql = "select * from account "; //返回的是ResultSet对象,与游标相似 ResultSet resultSet = statement.executeQuery(sql); // resultSet.moveToCurrentRow();//当前行 // resultSet.moveToInsertRow();//插入行? while (resultSet.next()/*若还有下一行,则返回true,并进入下一行*/) { // String userName = resultSet.getString("username");//根据列名查询 // String password = resultSet.getString("password"); } } /** * 查询用户是否存在 * @param userName * @return true-->存在 false-->不存在 * @throws SQLException */ public boolean userNmaeExist(String userName) throws SQLException { Statement statement = connection.createStatement(); String sql = "select * from account where username ='"+userName+"'"; ResultSet resultSet = statement.executeQuery(sql); if (resultSet.next()) { return true; }else { return false; } } /** * 查询用户跟密码是否正确 * @throws SQLException */ public boolean accountExist(String userName, String password) throws SQLException { Statement statement = connection.createStatement(); String sql = "select * from account where username = '"+userName+"'and password ='"+password+"'"; ResultSet resultSet = statement.executeQuery(sql); if (resultSet.next()) { return true; }else { return false; } } }